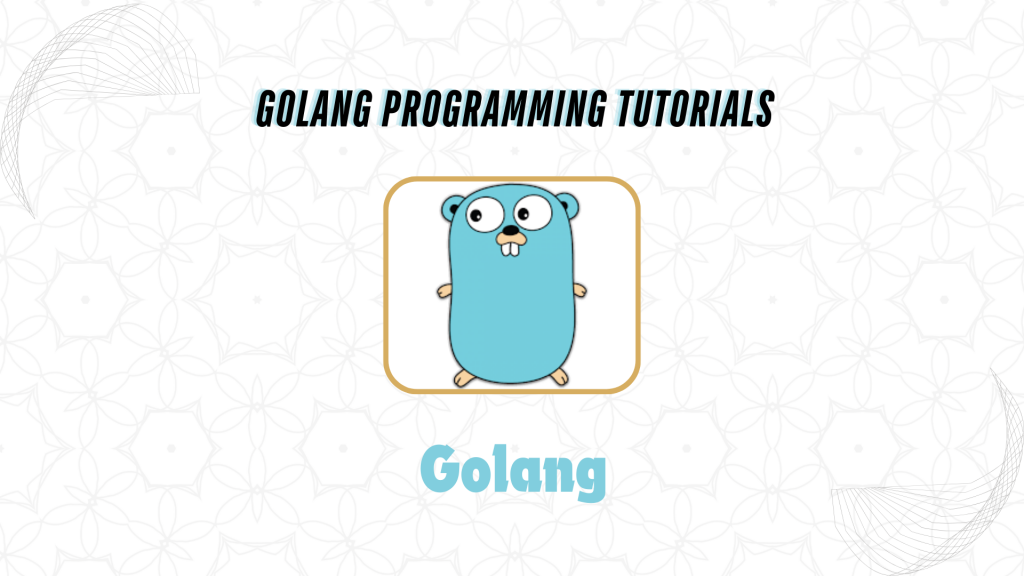
Welcome to the GoLang Programming Tutorials! Below is a structured guide that will take you from the basics of GoLang to advanced concepts. Click on each lesson to dive deeper into GoLang programming.
Lesson 1: Introduction to GoLang
- What is GoLang?
- Why Learn GoLang?
- Key Features of Go
- Setting Up the GoLang Environment
- Writing Your First Go Program
Lesson 2: Variables, Constants, Data Types, and Values
- Understanding Variables in Go
- Working with Constants
- Overview of Data Types in Go
- Using Values in GoLang
- Type Conversion in GoLang
Lesson 3: Control Structures in GoLang
- Conditional Statements
- Loops in Go (for loop, range loop)
- Switch Case in GoLang
- Break and Continue in Loops
Lesson 4: Functions in GoLang
- Defining and Calling Functions
- Function Parameters and Return Values
- Variadic Functions
- Anonymous Functions and Closures
Lesson 5: Arrays, Slices, and Maps in GoLang
- Introduction to Arrays
- Working with Slices
- Using Maps for Key-Value Pairs
Lesson 6: Structs and Methods in GoLang
- Understanding Structs in Go
- Defining and Using Methods
- Embedding Structs
Lesson 7: GoLang Interfaces
- What are Interfaces?
- Implementing Interfaces
- Interface Practical Use Cases
Lesson 8: Error Handling in GoLang
- Handling Errors in Go
- Using defer, panic, and recover
Lesson 9: Concurrency in GoLang
- Understanding Goroutines
- Using Channels for Communication
- Syncing Goroutines with WaitGroup
- Select Statement for Multiplexing
Lesson 10: Working with Packages in GoLang
- Standard Library Packages
- Creating and Importing Custom Packages
- Package Visibility and Scope
Lesson 11: GoLang Testing
- Writing Unit Tests in Go
- Using the testing Package
- Benchmarking Code
Lesson 12: Timers and Tickers
- Timers
- Tickers
Lesson 13: Worker Pools and Synchronization
- Worker Pools
- WaitGroups
- Rate Limiting
Lesson 14: Atomic Operations and Mutexes
- Atomic Counters
- Mutexes
- Stateful Goroutines
Lesson 15: Sorting and Advanced Functions
- Sorting
- Sorting by Functions
Lesson 16: Panic, Defer, and Recover
- Panic
- Defer
- Recover
Lesson 17: String Manipulation
- String Functions
- String Formatting
- Text Templates
- Regular Expressions
Lesson 18: Working with Data Formats
- JSON
- XML
Lesson 19: Time and Dates
- Time
- Epoch
- Time Formatting / Parsing
Lesson 20: Random Numbers and Hashing
- Random Numbers
- Number Parsing
- URL Parsing
- SHA256 Hashes
- Base64 Encoding
Lesson 21: File Handling
- Reading Files
- Writing Files
- Line Filters
- File Paths
- Directories
- Temporary Files and Directories
- Embed Directive
Lesson 22: Testing and Benchmarking
- Testing and Benchmarking
Lesson 23: Command-Line Utilities
- Command-Line Arguments
- Command-Line Flags
- Command-Line Subcommands
Lesson 24: Environment Variables and Logging
- Environment Variables
- Logging
Lesson 25: HTTP and Web Development
- HTTP Client
- HTTP Server
Lesson 26: Context and Processes
- Context
- Spawning Processes
- Exec’ing Processes
- Signals
- Exit