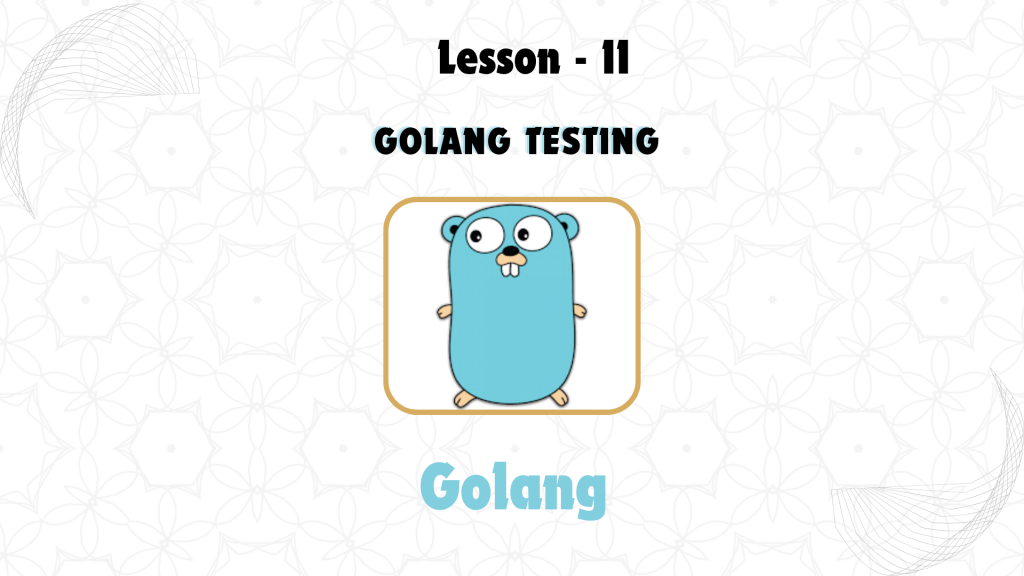
Testing is an essential part of software development, and GoLang offers a simple yet powerful testing framework built directly into the language. The Go testing framework allows developers to write unit tests, benchmarks, and examples to ensure the correctness and performance of their code.
1. Writing Unit Tests in Go
GoLang has a built-in package testing
to write and execute unit tests. A unit test in Go typically checks if a function or small part of the code behaves as expected.
Unit test files should follow these conventions:
- Test files should be in the same package as the code they test.
- The test file must have the suffix
_test.go
. - Each test function must start with
Test
and take a single parameter of type*testing.T
.
Example: Let’s say you have the following simple function that adds two integers:
// main.go
package main
func Add(a, b int) int {
return a + b
}
To test the Add
function, you would write a test in main_test.go
:
// main_test.go
package main
import "testing"
func TestAdd(t *testing.T) {
result := Add(2, 3)
expected := 5
if result != expected {
t.Errorf("Add(2, 3) = %d; want %d", result, expected)
}
}
- The
t.Errorf
function is used to log errors when the actual result doesn’t match the expected value. - If any test fails, Go reports it when running the tests.
2. Using the testing
Package
Go’s testing
package provides tools to create unit tests, benchmarks, and example-based tests.
- Test Functions: As demonstrated, test functions are prefixed with
Test
and take a*testing.T
parameter. - Assertions: Go doesn’t have built-in assertion libraries like other languages. Instead, you compare values manually and use
t.Error
ort.Fatalf
to report mismatches.
To run the tests, you can use the following command:
go test
This will automatically detect and run all test functions in your package.
3. Benchmarking Code
Go also provides support for performance testing through benchmarks. A benchmark function in Go must start with Benchmark
and take a *testing.B
parameter. The function should run the code multiple times to get an accurate measure of performance.
Example of a Benchmark Test:
func BenchmarkAdd(b *testing.B) {
for i := 0; i < b.N; i++ {
Add(2, 3)
}
}
- The
b.N
value tells Go how many times the function should be executed to measure performance. - To run benchmark tests, you can use:
go test -bench=.
This command will run all benchmarks in your package and output the performance results.
4. Example Tests
GoLang also allows you to write example functions that serve as both documentation and tests. Example functions start with Example
and provide sample usage of a function.
Example:
func ExampleAdd() {
fmt.Println(Add(2, 3))
// Output: 5
}
- The
Output
comment specifies the expected output when the example is run. If the output doesn’t match, the example test will fail.
You can run example tests using:
go test
5. Table-Driven Tests
A common pattern in Go testing is the table-driven test, where multiple test cases are defined in a table (a slice of structs), and the test logic is applied to each case.
Example of a Table-Driven Test:
func TestAddTable(t *testing.T) {
tests := []struct {
a, b, expected int
}{
{1, 2, 3},
{5, 5, 10},
{-1, -1, -2},
}
for _, tt := range tests {
result := Add(tt.a, tt.b)
if result != tt.expected {
t.Errorf("Add(%d, %d) = %d; want %d", tt.a, tt.b, result, tt.expected)
}
}
}
- In this case, the
tests
slice defines various inputs and expected outputs for theAdd
function. - The test loop runs each case and checks the result against the expected value.
6. Subtests
Go also supports subtests, which allow you to run a set of related tests under a single parent test. This is useful for testing multiple aspects of a function or testing with different configurations.
Example of Subtests:
func TestAddSubTests(t *testing.T) {
t.Run("Positive numbers", func(t *testing.T) {
result := Add(1, 2)
if result != 3 {
t.Errorf("Expected 3 but got %d", result)
}
})
t.Run("Negative numbers", func(t *testing.T) {
result := Add(-1, -1)
if result != -2 {
t.Errorf("Expected -2 but got %d", result)
}
})
}
- Each subtest is executed as part of the parent test and can be viewed separately in test output.
Key Takeaways:
- Unit Testing: Ensures each function works as expected by testing individual components of your program.
- Benchmarking: Measures the performance of your code, identifying bottlenecks.
- Example Tests: Provides documentation that doubles as a test to verify that examples behave as intended.
- Table-Driven Tests: Streamlines multiple test cases for a function into a single, easy-to-read table format.
- Subtests: Useful for running related tests as individual subtests, providing detailed reporting and control.
By utilizing GoLang’s built-in testing framework, you can ensure your code is reliable, performant, and maintainable. Effective testing practices will help you catch bugs early and improve your code’s overall quality.