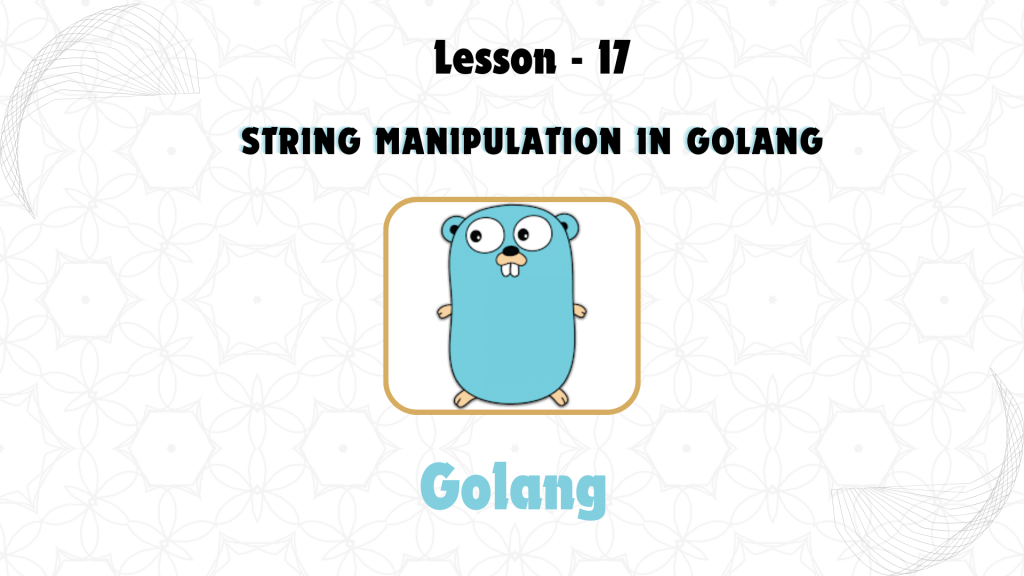
In this lesson, we will explore how to work with strings in GoLang. Strings are an essential part of any programming language, and GoLang provides powerful tools and functions for handling them efficiently. In GoLang, strings are immutable sequences of bytes, which means once a string is created, it cannot be modified directly. However, Go offers a rich set of functions to manipulate and work with strings.
1. String Functions in GoLang
GoLang’s strings
package offers many utility functions to manipulate and analyze strings.
Common String Functions:
strings.Contains()
: Checks if a substring exists within a string.strings.HasPrefix()
: Checks if a string starts with a specified prefix.strings.HasSuffix()
: Checks if a string ends with a specified suffix.strings.Index()
: Returns the index of the first occurrence of a substring.strings.ToLower()
/strings.ToUpper()
: Converts a string to lowercase or uppercase.
Example:
package main
import (
"fmt"
"strings"
)
func main() {
s := "Hello, GoLang!"
fmt.Println(strings.Contains(s, "Go")) // true
fmt.Println(strings.HasPrefix(s, "Hel")) // true
fmt.Println(strings.HasSuffix(s, "!")) // true
fmt.Println(strings.Index(s, "Go")) // 7
fmt.Println(strings.ToLower(s)) // hello, golang!
fmt.Println(strings.ToUpper(s)) // HELLO, GOLANG!
}
Explanation:
- These basic string functions allow us to check for substrings, manipulate case, and search within strings easily.
2. String Formatting in GoLang
String formatting is useful for generating dynamic strings that include variables and data types. GoLang uses the fmt
package to format strings.
Common Formatting Verbs:
%s
: For strings%d
: For integers%f
: For floating-point numbers%v
: For any value (default format)%T
: For the type of the value
Example:
package main
import "fmt"
func main() {
name := "Alice"
age := 25
salary := 50000.50
formattedStr := fmt.Sprintf("Name: %s, Age: %d, Salary: %.2f", name, age, salary)
fmt.Println(formattedStr)
}
Explanation:
- The
fmt.Sprintf()
function formats the string using the placeholders and the provided values.
3. Text Templates in GoLang
Text templates allow you to create dynamic content by embedding variables or logic within the string. GoLang provides the text/template
package to handle templates efficiently.
Example:
package main
import (
"os"
"text/template"
)
type Person struct {
Name string
Age int
}
func main() {
tmpl := "Name: {{.Name}}, Age: {{.Age}}"
t, _ := template.New("person").Parse(tmpl)
p := Person{Name: "Alice", Age: 25}
t.Execute(os.Stdout, p)
}
Explanation:
- In the template string
{{.Name}}
and{{.Age}}
represent the fields of thePerson
struct. - The
template.Execute()
method fills in the template with the provided data.
4. Regular Expressions in GoLang
Regular expressions are patterns used to match and manipulate text. GoLang provides the regexp
package to work with regular expressions.
Common Functions:
regexp.MatchString()
: Checks if a string matches the regular expression.regexp.FindString()
: Finds the first match for the regular expression.regexp.ReplaceAllString()
: Replaces all occurrences of the regular expression with a replacement string.
Example:
package main
import (
"fmt"
"regexp"
)
func main() {
re := regexp.MustCompile(`\d+`)
s := "There are 100 apples and 50 oranges."
fmt.Println(re.MatchString(s)) // true
fmt.Println(re.FindString(s)) // 100
fmt.Println(re.ReplaceAllString(s, "XX")) // There are XX apples and XX oranges.
}
Explanation:
- In this example,
\d+
is the regular expression for matching one or more digits in the string. regexp.MustCompile()
compiles the pattern, and functions likeMatchString()
andFindString()
work on it.
Key Takeaways:
- The
strings
package provides powerful built-in functions to manipulate strings in GoLang. - String formatting using the
fmt
package allows you to dynamically create formatted strings. - The
text/template
package enables building dynamic content with template strings. - Regular expressions offer a way to match patterns within strings for more complex text manipulation tasks.
Understanding how to effectively manipulate strings and work with templates and regular expressions will enhance your ability to build robust GoLang applications.