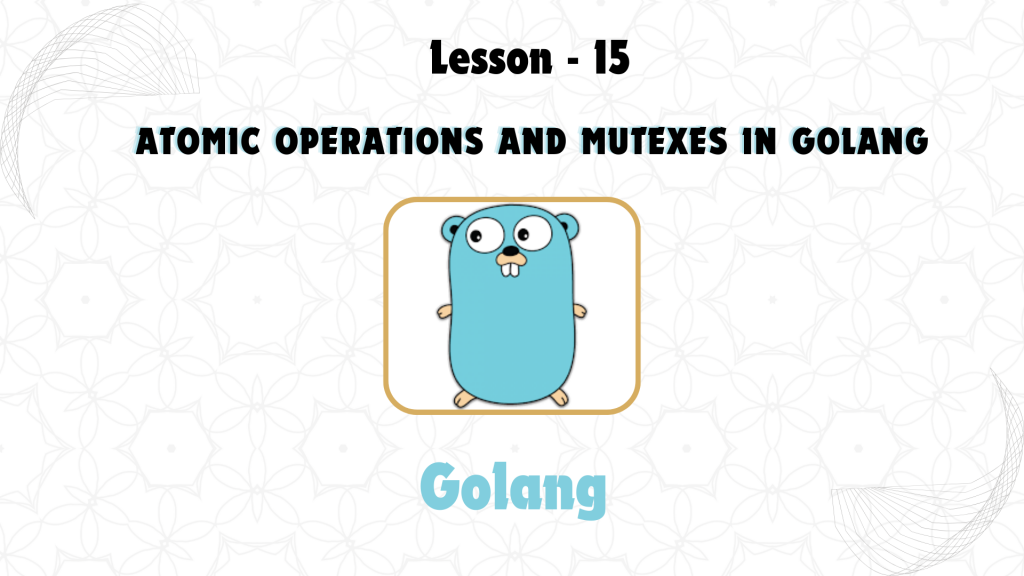
Goroutines and channels are key features of GoLang that make it well-suited for concurrent programming. Goroutines are lightweight, efficient threads managed by the Go runtime, and channels are used for communication between these goroutines. This lesson delves into how to use goroutines and channels to build scalable, concurrent applications.
1. What are Goroutines?
A goroutine is a function that runs concurrently with other functions. Unlike traditional threads, goroutines are more lightweight and consume less memory. They are managed by the Go runtime, which handles the scheduling of these goroutines.
Syntax for Starting a Goroutine:
go functionName(arguments)
The go
keyword starts a function as a goroutine, allowing it to run in the background concurrently.
Example:
package main
import (
"fmt"
"time"
)
func printMessage(message string) {
for i := 0; i < 3; i++ {
fmt.Println(message)
time.Sleep(time.Second)
}
}
func main() {
go printMessage("Hello from Goroutine")
printMessage("Hello from Main Function")
}
Explanation:
- The
printMessage
function is called both directly and as a goroutine. - The main function and the goroutine run concurrently.
- The program may terminate before the goroutine finishes unless proper synchronization is used (e.g.,
WaitGroup
).
2. Channels in GoLang
Channels are the primary mechanism for communication between goroutines. They allow you to pass data between goroutines in a safe and synchronized way.
Basic Channel Syntax:
- Declaring a Channel:
var channelName chan dataType
Sending Data to a Channel:
channelName <- value
Receiving Data from a Channel:
value := <-channelName
Example:
package main
import (
"fmt"
)
func sendData(channel chan string) {
channel <- "Hello from Goroutine"
}
func main() {
ch := make(chan string)
go sendData(ch)
message := <-ch
fmt.Println(message)
}
Explanation:
- A channel
ch
is created usingmake(chan string)
. - The
sendData
function sends a message to the channel. - The main function receives the message from the channel using
<-ch
.
3. Buffered vs. Unbuffered Channels
- Unbuffered Channels: The sender will block until the receiver is ready to receive the data, and vice versa.
- Buffered Channels: Buffered channels allow you to specify a capacity, meaning the sender can send data even if the receiver is not ready (up to the buffer’s limit).
Syntax for Buffered Channel:
channel := make(chan dataType, capacity)
Example of Buffered Channel:
package main
import (
"fmt"
)
func sendData(channel chan string) {
channel <- "Message 1"
channel <- "Message 2"
}
func main() {
ch := make(chan string, 2)
go sendData(ch)
fmt.Println(<-ch)
fmt.Println(<-ch)
}
Explanation:
- A buffered channel
ch
is created with a capacity of 2. - Two messages are sent without blocking because the buffer can hold them both.
4. Synchronization with WaitGroup
Sometimes, you need to ensure that all goroutines finish their work before the program exits. The sync.WaitGroup
type is used to wait for a collection of goroutines to finish.
Example:
package main
import (
"fmt"
"sync"
)
func printMessage(wg *sync.WaitGroup, message string) {
defer wg.Done() // Mark this goroutine as done
fmt.Println(message)
}
func main() {
var wg sync.WaitGroup
wg.Add(1) // Add a goroutine to the waitgroup
go printMessage(&wg, "Hello from Goroutine")
wg.Wait() // Wait for all goroutines to finish
}
Explanation:
wg.Add(1)
increments the counter, signaling that a goroutine is starting.wg.Done()
decrements the counter when the goroutine finishes.wg.Wait()
blocks until all goroutines have completed.
5. Channel Direction
In GoLang, channels can be specified to only send or receive data, improving type safety. A send-only channel is a channel that can only send data, while a receive-only channel can only receive data.
Example:
package main
import (
"fmt"
)
func sendData(channel chan<- string) { // send-only channel
channel <- "Hello from Sender"
}
func receiveData(channel <-chan string) { // receive-only channel
fmt.Println(<-channel)
}
func main() {
ch := make(chan string)
go sendData(ch)
receiveData(ch)
}
Explanation:
- The
sendData
function only sends data to the channel, and thereceiveData
function only receives data. - Specifying the direction of the channel ensures that channels are used properly, avoiding mistakes.
6. Select Statement
The select statement is a powerful control structure that allows you to wait on multiple channel operations. It lets a goroutine monitor multiple channels at once and perform actions based on which channel is ready.
Example:
package main
import (
"fmt"
"time"
)
func main() {
ch1 := make(chan string)
ch2 := make(chan string)
go func() {
time.Sleep(1 * time.Second)
ch1 <- "Message from Channel 1"
}()
go func() {
time.Sleep(2 * time.Second)
ch2 <- "Message from Channel 2"
}()
select {
case msg1 := <-ch1:
fmt.Println(msg1)
case msg2 := <-ch2:
fmt.Println(msg2)
}
}
Explanation:
- The
select
statement listens on bothch1
andch2
. - As soon as one of the channels is ready (in this case
ch1
after 1 second), the corresponding case is executed.
Key Takeaways:
- Goroutines are lightweight, concurrent functions managed by Go’s runtime, allowing parallel execution.
- Channels are the primary means of communication between goroutines, ensuring data is passed safely.
- Buffered channels allow for non-blocking communication up to a certain limit, whereas unbuffered channels block until both sender and receiver are ready.
- The select statement provides a way to handle multiple channel operations simultaneously, making it easy to manage complex concurrency scenarios.