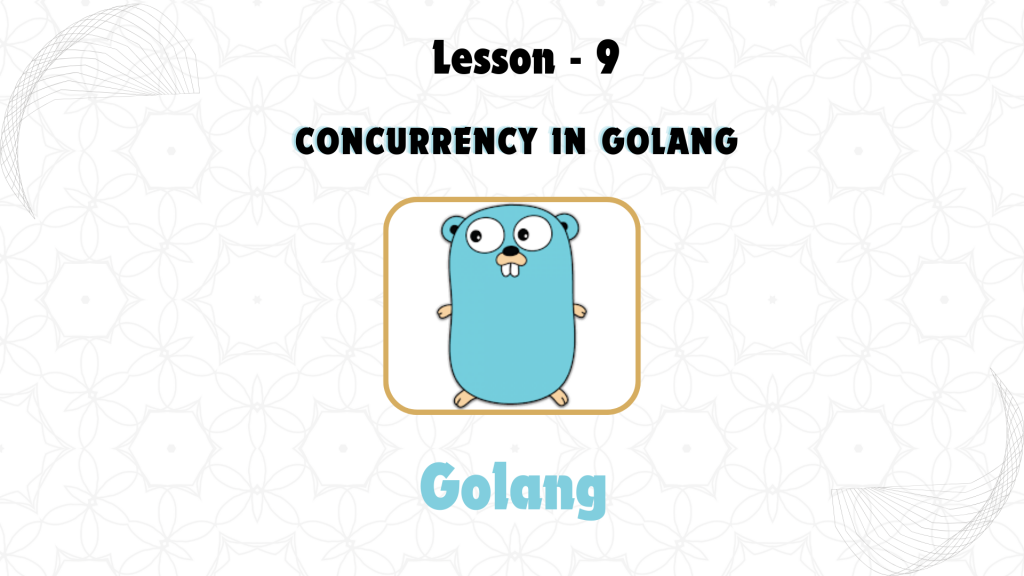
Concurrency is one of GoLang’s standout features, and it provides a highly efficient and simple way to manage multiple tasks simultaneously. In Go, concurrency is handled via goroutines and channels, which enable lightweight, scalable parallel processing.
1. Understanding Concurrency in GoLang
Concurrency allows multiple tasks to be executed at the same time, improving the efficiency and performance of applications. Go makes this easy to manage with goroutines, which are functions that run concurrently with other functions.
2. Goroutines
A goroutine is a lightweight thread of execution. They are more memory-efficient than traditional threads in other programming languages, making GoLang ideal for building high-concurrency applications.
To start a goroutine, simply add the go
keyword before a function call:
Example:
package main
import (
"fmt"
"time"
)
func printNumbers() {
for i := 1; i <= 5; i++ {
fmt.Println(i)
time.Sleep(1 * time.Second) // Simulate a time delay
}
}
func main() {
go printNumbers() // Start a goroutine
fmt.Println("Goroutine started")
time.Sleep(6 * time.Second) // Wait to allow goroutine to finish
fmt.Println("Main function ends")
}
In this example, printNumbers
runs as a separate goroutine, allowing the main function to continue without waiting for it to finish.
3. Channels: Communication Between Goroutines
Channels provide a way for goroutines to communicate and synchronize. They allow you to send and receive values between goroutines safely.
To create a channel in Go:
c := make(chan int)
Sending and receiving data via a channel:
c <- 42 // Send value to channel
x := <- c // Receive value from channel
Example:
package main
import (
"fmt"
)
func worker(c chan int) {
c <- 10 // Send value to channel
}
func main() {
channel := make(chan int)
go worker(channel) // Start worker goroutine
result := <-channel // Receive value from channel
fmt.Println("Received:", result)
}
Here, the worker goroutine sends a value to the main goroutine through a channel, allowing them to communicate safely.
4. Syncing Goroutines with WaitGroups
When you have multiple goroutines and need to ensure that all complete before the main function finishes, you can use a WaitGroup. It’s a synchronization mechanism to wait for goroutines to finish.
Example:
package main
import (
"fmt"
"sync"
)
func worker(id int, wg *sync.WaitGroup) {
defer wg.Done() // Notify WaitGroup that this goroutine is done
fmt.Printf("Worker %d started\n", id)
}
func main() {
var wg sync.WaitGroup
for i := 1; i <= 3; i++ {
wg.Add(1)
go worker(i, &wg) // Start 3 worker goroutines
}
wg.Wait() // Wait for all goroutines to finish
fmt.Println("All workers completed")
}
In this example, the WaitGroup waits for all worker goroutines to complete before the program exits.
5. Select Statement for Multiplexing Channels
The select statement is a powerful tool in GoLang that lets you handle multiple channel operations simultaneously. It’s similar to a switch
statement but works with channels.
Example:
package main
import "fmt"
func main() {
c1 := make(chan string)
c2 := make(chan string)
go func() {
c1 <- "Hello from channel 1"
}()
go func() {
c2 <- "Hello from channel 2"
}()
select {
case msg1 := <-c1:
fmt.Println(msg1)
case msg2 := <-c2:
fmt.Println(msg2)
}
}
Here, the select
statement listens to both channels and prints whichever message arrives first.
Key Takeaways:
- Goroutines: Lightweight, concurrent functions that allow you to perform tasks in parallel.
- Channels: Used for safe communication between goroutines.
- WaitGroups: Syncs goroutines to ensure all tasks are complete before proceeding.
- Select Statement: Handles multiple channels simultaneously, improving efficiency in concurrent programming.
Best Practices:
- Avoid shared state between goroutines; use channels for safe communication.
- Use goroutines for tasks that can be done in parallel to improve application performance.
- Use WaitGroups to ensure all goroutines complete before exiting the main program.
- Use the select statement to handle multiple channels efficiently.
Concurrency in GoLang is simple, efficient, and highly effective. By using goroutines and channels, you can create scalable applications that perform multiple tasks concurrently with ease.