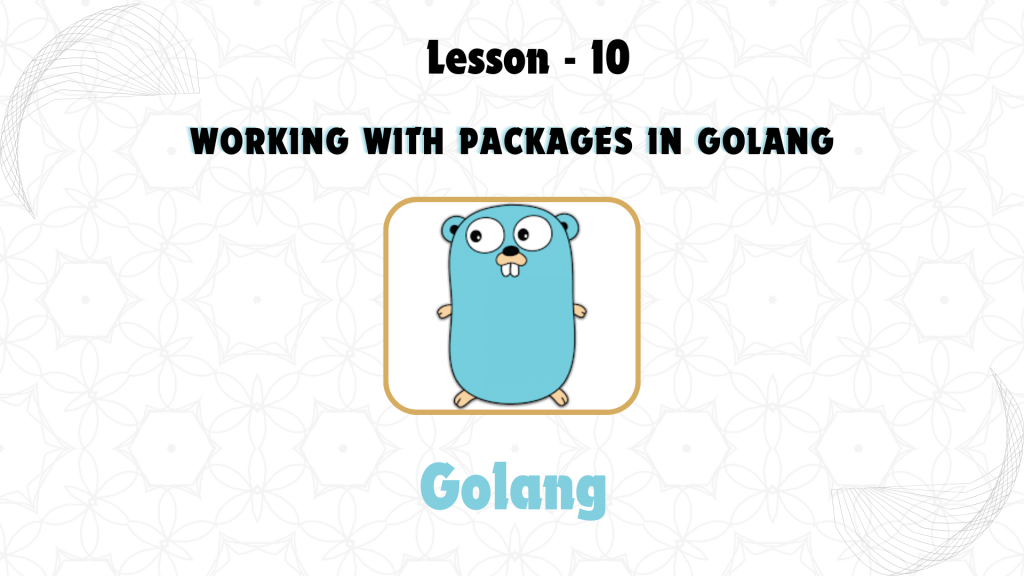
GoLang provides an extensive ecosystem of packages that allow you to structure your code efficiently and reuse functionality across multiple projects. Packages in GoLang are similar to libraries or modules in other programming languages, and they play a vital role in organizing and maintaining the scalability of codebases.
1. Understanding Packages in GoLang
A package is essentially a collection of related Go source files, and each Go program is made up of packages. The standard library offers a rich set of packages, but you can also create your own or import third-party ones.
- Main Package: The entry point for all Go programs is the
main
package. - Other Packages: Go standard library has packages for file handling, HTTP, JSON, and more.
Every Go source file must start with a package
declaration:
package main
2. Importing Packages
To use functions or types from other packages, you need to import them. GoLang provides a built-in mechanism to import both standard and custom packages using the import
keyword.
Example of Importing Standard Packages:
package main
import (
"fmt"
"time"
)
func main() {
fmt.Println("Hello, Go!")
fmt.Println("The current time is:", time.Now())
}
In this example, the fmt
package is used for formatted I/O, while the time
package provides functions to handle dates and times.
3. Creating and Importing Custom Packages
You can create your own packages by organizing Go files into directories. The directory name serves as the package name. Once you create a custom package, you can import it into your main application.
Steps to Create a Custom Package:
- Create a directory named after your package.
- Inside the directory, create Go source files starting with the package declaration.
- Functions and variables that you want to be accessible outside the package must start with an uppercase letter (this is GoLang’s convention for making things “exported” or public).
Example:
- Directory Structure:
myapp/
├── main.go
└── greetings/
└── greet.go
greet.go (Custom Package):
package greetings
import "fmt"
// Exported function (starts with uppercase)
func Hello() {
fmt.Println("Hello from the greetings package!")
}
main.go (Importing the Custom Package):
package main
import (
"myapp/greetings" // Importing the custom package
)
func main() {
greetings.Hello() // Calling a function from the custom package
}
When you run the main.go
file, it will print “Hello from the greetings package!” by using the custom package you created.
4. Package Visibility and Scope
GoLang uses capitalization to determine visibility. Identifiers (variables, constants, functions, etc.) that begin with a capital letter are exported from the package and can be accessed by other packages. If an identifier starts with a lowercase letter, it is unexported and can only be accessed within the package.
Example:
package greetings
// Exported function (public)
func Hello() {
fmt.Println("Hello, World!")
}
// Unexported function (private to the package)
func goodbye() {
fmt.Println("Goodbye!")
}
In this case, Hello
is accessible to other packages, but goodbye
is not.
5. Go Modules: Managing Dependencies
When working on large projects with multiple packages, it’s essential to manage dependencies. Go modules help you manage versions of dependencies and resolve package paths easily.
Creating a Go Module:
go mod init myapp
This command creates a go.mod
file, which tracks the project’s dependencies. When you import external packages, GoLang automatically adds them to the go.mod
file.
Example:
require github.com/sirupsen/logrus v1.8.1
This line in go.mod
indicates that your project depends on version 1.8.1 of the logrus
logging library.
Key Takeaways:
- Packages: Organize related code and improve reusability.
- Standard Packages: GoLang’s rich standard library helps handle common tasks.
- Custom Packages: Allow for cleaner code organization and separation of concerns.
- Visibility: Capitalized identifiers are exported (public), while lowercase identifiers are private to the package.
- Go Modules: Help manage dependencies and versioning.
By mastering GoLang’s package system, you can write modular, maintainable, and reusable code. Whether working with standard library packages or creating your own, packages are essential in scaling Go applications.