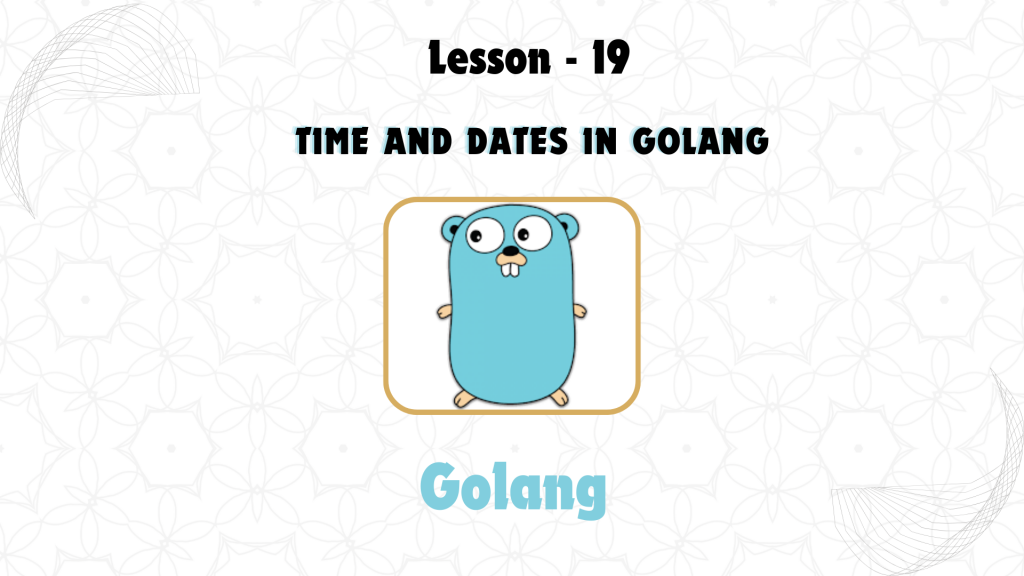
In Lesson 19, we’ll explore how GoLang manages time and dates. Time manipulation is a critical aspect of many applications, whether you’re dealing with timestamps, scheduling, or formatting time for display. GoLang’s time
package provides powerful tools for managing time, dates, and durations.
1. Working with Time in GoLang
GoLang’s time
package provides the Time
type to represent an instance in time. The package includes functions to get the current time, perform calculations with time, and format it.
Getting the Current Time
You can get the current local time using time.Now()
.
Example:
package main
import (
"fmt"
"time"
)
func main() {
currentTime := time.Now()
fmt.Println("Current Time: ", currentTime)
}
Output:
Current Time: 2024-10-10 12:34:56.789123456 +0530 IST
Creating a Specific Time
You can also create a specific date and time using time.Date()
.
Example:
package main
import (
"fmt"
"time"
)
func main() {
specificTime := time.Date(2023, time.September, 15, 10, 30, 0, 0, time.UTC)
fmt.Println("Specific Time: ", specificTime)
}
Explanation:
- This creates a
Time
instance for September 15, 2023, at 10:30:00 AM UTC.
2. Working with Epoch Time
Epoch (also known as Unix time) represents the number of seconds that have elapsed since January 1, 1970 (midnight UTC). Go provides support for working with epoch timestamps.
Getting the Epoch Timestamp
Use time.Now().Unix()
to get the current time in Unix timestamp format (seconds since the epoch).
Example:
package main
import (
"fmt"
"time"
)
func main() {
currentEpoch := time.Now().Unix()
fmt.Println("Current Epoch Time: ", currentEpoch)
}
Output:
Current Epoch Time: 1700000000
Converting Epoch to Time
You can convert a Unix timestamp to a Time
object using time.Unix()
.
Example:
package main
import (
"fmt"
"time"
)
func main() {
epoch := int64(1700000000)
timeObject := time.Unix(epoch, 0)
fmt.Println("Time from Epoch: ", timeObject)
}
3. Time Formatting and Parsing
GoLang provides a unique way to format and parse time using reference time: Mon Jan 2 15:04:05 MST 2006
. This specific date and time are used as the reference for formatting.
Formatting Time
To format a Time
object into a specific string, use time.Format()
.
Example:
package main
import (
"fmt"
"time"
)
func main() {
currentTime := time.Now()
formattedTime := currentTime.Format("2006-01-02 15:04:05")
fmt.Println("Formatted Time: ", formattedTime)
}
Output:
Formatted Time: 2024-10-10 12:34:56
Parsing Time
To convert a string representation of time into a Time
object, use time.Parse()
.
Example:
package main
import (
"fmt"
"time"
)
func main() {
timeString := "2024-10-10 12:34:56"
parsedTime, err := time.Parse("2006-01-02 15:04:05", timeString)
if err != nil {
fmt.Println("Error parsing time: ", err)
}
fmt.Println("Parsed Time: ", parsedTime)
}
4. Time Durations in GoLang
Durations in GoLang represent the difference between two times. They can be added, subtracted, or used in sleep functions.
Using Durations
Use time.Duration
to represent time intervals, such as hours, minutes, and seconds.
Example:
package main
import (
"fmt"
"time"
)
func main() {
start := time.Now()
time.Sleep(2 * time.Second)
end := time.Now()
duration := end.Sub(start)
fmt.Println("Duration: ", duration)
}
Explanation:
- This code measures the time duration between two
Time
instances.
Key Takeaways:
- GoLang’s
time
package provides a wide range of functionalities for working with time and dates, including getting the current time, working with epoch, and formatting/parsing time strings. - Time durations can be used to calculate differences between times or to perform operations after a delay.
- Understanding time and date manipulation is crucial for building systems that require accurate timestamps, scheduling, or time-sensitive operations.
By mastering GoLang’s time manipulation capabilities, you can handle all time-related needs in your applications.