Microservices architecture is a design approach where an application is composed of small, independent services that work together to achieve the overall functionality of the system. Unlike monolithic architectures, where all components are tightly integrated, microservices break down the application into smaller, loosely coupled services, each responsible for a specific business function. This modular approach allows for more flexibility, scalability, and ease of maintenance.
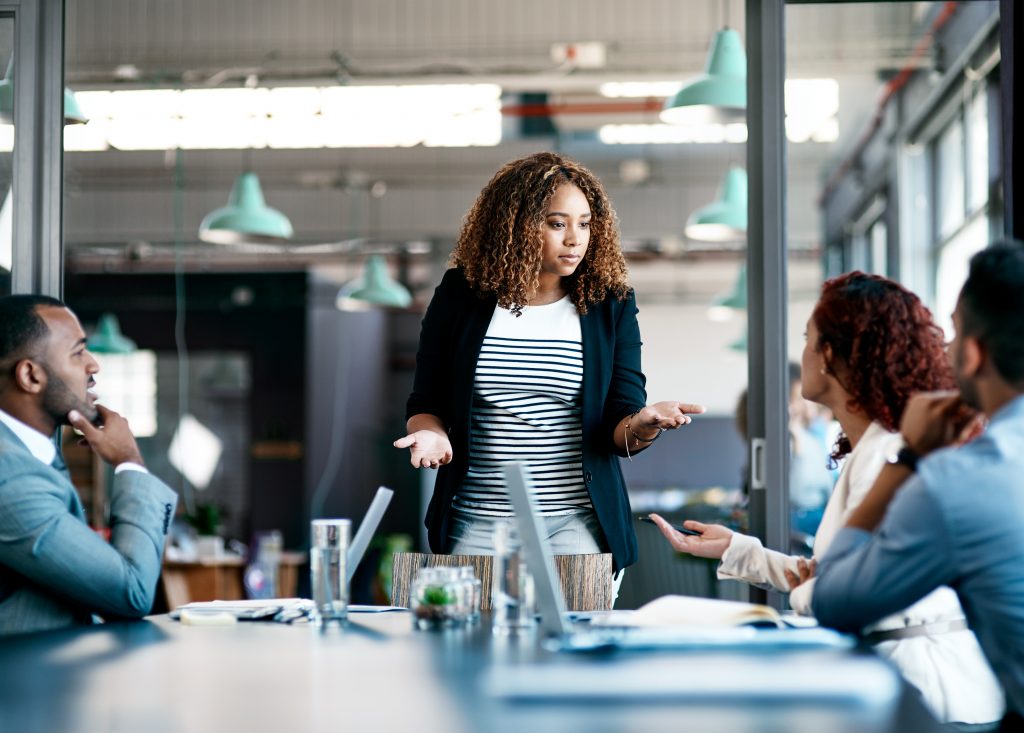
Overview of Microservices Architecture
In microservices architecture, each service operates independently, often with its own database and communication mechanism. These services interact with each other over network protocols like HTTP/REST, gRPC, or messaging queues. This decoupling of services provides several benefits, such as:
- Scalability: Individual services can be scaled independently based on demand, optimizing resource usage.
- Flexibility in Development: Different teams can work on different services, potentially using different programming languages or frameworks, allowing for faster development cycles.
- Resilience: Failure in one service doesn’t necessarily bring down the entire system, as services are isolated from one another.
However, this architectural style also introduces significant challenges, especially in testing.
Importance of Testing in Microservices Environments
Testing in a microservices environment is crucial due to the complexity and interdependence of services. Unlike monolithic applications where testing might focus on a single codebase, microservices require a more sophisticated approach to ensure that each service, as well as the interactions between services, functions correctly. Proper testing helps to:
- Ensure Service Integrity: Validate that each microservice performs its designated function correctly.
- Verify Inter-Service Communication: Ensure that data and requests are correctly exchanged between services.
- Maintain System Stability: Prevent cascading failures that could occur due to issues in a single service.
Challenges in Testing Microservices
Testing microservices introduces several unique challenges compared to traditional monolithic applications:
- Complexity Due to Distributed Nature:
- Each microservice operates independently, but they must work together as a cohesive system. Testing must account for the complexity of multiple services interacting over a network, often in unpredictable ways.
- Network latency, data consistency, and asynchronous communication add layers of complexity that must be thoroughly tested to avoid unexpected issues in production.
- Independent Deployment and Scalability:
- Microservices can be deployed and scaled independently, which means that the testing environment must be able to replicate different deployment scenarios, including various versions of services running simultaneously.
- This independence makes it challenging to ensure that updates to one service do not inadvertently break functionality in another.
- Communication Between Services:
- Services communicate over APIs, which introduces potential points of failure in the communication process, such as incorrect API responses, network issues, or mismatched data formats.
- Testing must cover these communication channels to ensure that services can reliably exchange data and handle failures gracefully.
Addressing these challenges requires a robust testing strategy that includes various testing levels, such as unit, integration, contract, and end-to-end testing. Each level plays a critical role in ensuring that the microservices architecture is resilient, scalable, and maintainable.
1. Unit Testing
Definition and Purpose
Unit testing involves writing test cases for individual components or services to ensure they perform as expected in isolation. In microservices architecture, this means testing each service’s functionality independently, without relying on external services or databases.
Tools and Best Practices
To effectively unit test microservices, developers commonly use tools like JUnit and Mockito in Java-based environments:
- JUnit: A testing framework that provides annotations and assertions to define and verify unit tests.
- Mockito: A mocking framework that allows you to create mock objects to simulate external dependencies in unit tests.
Example of a Unit Test Using JUnit and Mockito
Consider a simple microservice that manages user data. Here’s a basic example of how to unit test a service method that retrieves a user by ID:
// UserService.java
public class UserService {
private final UserRepository userRepository;
public UserService(UserRepository userRepository) {
this.userRepository = userRepository;
}
public User getUserById(Long id) {
return userRepository.findById(id)
.orElseThrow(() -> new UserNotFoundException("User not found"));
}
}
// UserServiceTest.java
@RunWith(MockitoJUnitRunner.class)
public class UserServiceTest {
@Mock
private UserRepository userRepository;
@InjectMocks
private UserService userService;
@Test
public void testGetUserById_UserExists() {
// Arrange
User mockUser = new User(1L, "John Doe", "johndoe@example.com");
Mockito.when(userRepository.findById(1L)).thenReturn(Optional.of(mockUser));
// Act
User user = userService.getUserById(1L);
// Assert
assertNotNull(user);
assertEquals("John Doe", user.getName());
assertEquals("johndoe@example.com", user.getEmail());
}
@Test(expected = UserNotFoundException.class)
public void testGetUserById_UserNotFound() {
// Arrange
Mockito.when(userRepository.findById(1L)).thenReturn(Optional.empty());
// Act
userService.getUserById(1L);
}
}
Explanation:
@RunWith(MockitoJUnitRunner.class)
: This tells JUnit to use the Mockito JUnit runner, which automatically initializes the mock objects.@Mock
: Marks theUserRepository
as a mock object, meaning it will simulate the behavior of the real repository.@InjectMocks
: Tells Mockito to inject the mock repository into theUserService
.Mockito.when()
: Defines the behavior of the mock object when a specific method is called.assertNotNull()
andassertEquals()
: JUnit assertions to verify the correctness of the method’s output.@Test(expected = UserNotFoundException.class)
: This test expects aUserNotFoundException
to be thrown, verifying how the service handles cases where the user is not found.
Importance of Mocking Dependencies
Mocking is crucial in microservices unit testing to isolate the service under test. By using mock objects, you can:
- Simulate Dependencies: As shown in the example, the
UserRepository
is mocked to simulate its behavior, allowing you to test theUserService
independently. - Control Scenarios: You can control what the mock returns, enabling you to test various scenarios, including edge cases and error conditions.
Challenges and Solutions
- Dealing with Dependencies Between Services: Microservices often rely on other services or databases. By mocking these dependencies, you can focus solely on the logic of the service under test.
- Solution: Use frameworks like Mockito to create mocks for any external dependencies.
- Ensuring Coverage: It’s essential to test all possible paths, including success and failure scenarios.
- Solution: Write comprehensive tests, covering all possible inputs and outputs.
- Maintaining Test Quality: As services evolve, test cases must be updated to reflect changes.
- Solution: Regularly refactor and review tests as part of the development cycle.
Strategies for Effective Unit Testing in Microservices
- Test Edge Cases: Ensure that your tests cover edge cases and unexpected inputs, such as null values or invalid IDs.
- Automate and Integrate: Use Continuous Integration (CI) tools like Jenkins to automate the execution of unit tests whenever code changes are made.
- Monitor Coverage: Use tools like JaCoCo to measure code coverage and ensure that all critical paths are tested.
By incorporating these practices, your unit tests will help ensure that your microservices are robust, reliable, and ready for production deployment.
2. Integration Testing
Definition and Importance
Integration testing is a crucial phase in microservices architecture where the focus shifts from testing individual components to testing the interactions between multiple services. Unlike unit tests that isolate a single service, integration tests ensure that services work together as intended, verifying that data flows correctly between them and that APIs communicate properly.
In a microservices environment, integration testing is essential because each service often depends on others to perform its role. These tests help identify issues that may not surface during unit testing, such as problems with data consistency, API mismatches, or network-related issues.
Approach and Tools
Integration testing for microservices requires a different set of tools and approaches compared to unit testing. Here’s how you can effectively perform integration testing:
- Spring Boot Test: If you’re using Spring Boot for your microservices, Spring Boot Test is a powerful tool that can help you run integration tests within a Spring application context. It allows you to load the full application context, including all beans and configuration, and test how different services interact.Example: A basic integration test for a Spring Boot microservice might look like this:
@RunWith(SpringRunner.class)
@SpringBootTest(webEnvironment = SpringBootTest.WebEnvironment.RANDOM_PORT)
public class UserServiceIntegrationTest {
@Autowired
private TestRestTemplate restTemplate;
@Test
public void testGetUserById() {
// Act
ResponseEntity<User> response = restTemplate.getForEntity("/users/1", User.class);
// Assert
assertEquals(HttpStatus.OK, response.getStatusCode());
assertNotNull(response.getBody());
assertEquals("John Doe", response.getBody().getName());
}
}
Explanation:
@SpringBootTest
: Loads the entire application context for testing, allowing you to test the service as it would run in production.TestRestTemplate
: A Spring-provided class that allows you to send HTTP requests to your service and verify the responses.- Integration Focus: This test ensures that the
UserService
correctly handles a REST API call to retrieve a user by ID.
TestContainers: TestContainers is a popular tool for running Docker containers in your tests. It allows you to spin up real instances of dependencies like databases, message brokers, or other microservices, ensuring that your tests are as close to a real environment as possible.
Example: Using TestContainers to test a microservice that interacts with a PostgreSQL database:
public class UserServiceIntegrationTest {
@Container
public static PostgreSQLContainer<?> postgreSQLContainer = new PostgreSQLContainer<>("postgres:latest")
.withDatabaseName("testdb")
.withUsername("user")
.withPassword("password");
@Autowired
private UserRepository userRepository;
@Test
public void testDatabaseIntegration() {
// Arrange
User user = new User("John Doe", "johndoe@example.com");
userRepository.save(user);
// Act
User foundUser = userRepository.findByName("John Doe");
// Assert
assertNotNull(foundUser);
assertEquals("johndoe@example.com", foundUser.getEmail());
}
}
- Explanation:
- TestContainers: Automatically starts a PostgreSQL database in a Docker container for the duration of the test.
- Realistic Environment: This approach tests the service against a real database instance, ensuring that database-related issues are caught early.
Testing Service Communication, Data Flow, and API Interactions
In microservices, services communicate with each other via APIs, passing data through HTTP requests, message queues, or other communication protocols. Integration tests should focus on:
- Service Communication: Ensure that services can correctly call each other’s APIs and handle responses appropriately.
- Data Flow: Verify that data is correctly passed between services and that transformations or mappings are accurate.
- API Interactions: Test the full lifecycle of an API call, including request validation, response generation, and error handling.
Example: Testing the interaction between two microservices (e.g., OrderService
and PaymentService
):
@RunWith(SpringRunner.class)
@SpringBootTest(webEnvironment = SpringBootTest.WebEnvironment.RANDOM_PORT)
public class OrderServiceIntegrationTest {
@MockBean
private PaymentService paymentService;
@Autowired
private TestRestTemplate restTemplate;
@Test
public void testOrderCreationWithPayment() {
// Arrange
Mockito.when(paymentService.processPayment(any(PaymentRequest.class)))
.thenReturn(new PaymentResponse("SUCCESS"));
// Act
ResponseEntity<OrderResponse> response = restTemplate.postForEntity("/orders", new OrderRequest(...), OrderResponse.class);
// Assert
assertEquals(HttpStatus.OK, response.getStatusCode());
assertEquals("SUCCESS", response.getBody().getPaymentStatus());
}
}
Handling Dependencies
In a microservices architecture, services often depend on others to complete their operations. Managing these dependencies during integration testing is critical:
- Use of Stubs and Mocks: For dependent services that are not under test, you can use stubs or mocks to simulate their behavior. This helps isolate the service you’re testing while still verifying that it interacts correctly with others.Example: In the above
OrderServiceIntegrationTest
,PaymentService
is mocked to simulate the payment process, allowing the test to focus on the order creation logic. - Ensuring Data Consistency Across Services: One of the challenges in integration testing is ensuring that data remains consistent across multiple services. This can be especially tricky when services are interacting with shared databases or need to maintain synchronized state.
- Solution: Use a combination of transactional tests and data validation checks to ensure that all services maintain consistent data throughout the test scenario.
Example: Using transactional tests to ensure consistency:
@Transactional
public void testOrderAndPaymentConsistency() {
// Arrange
Order order = new Order(...);
orderService.createOrder(order);
// Act
Payment payment = paymentService.processPayment(order.getId());
// Assert
assertEquals(order.getId(), payment.getOrderId());
assertTrue(orderRepository.existsById(order.getId()));
assertTrue(paymentRepository.existsById(payment.getId()));
}
Integration testing in microservices is vital for ensuring that different services can work together seamlessly. By using tools like Spring Boot Test and TestContainers, along with strategies for handling dependencies and ensuring data consistency, you can create robust integration tests that help maintain the reliability and stability of your microservices architecture.
3. Contract Testing
Overview of Contract Testing
In a microservices architecture, where services communicate with each other via APIs, it’s crucial to ensure that the contracts (the agreed-upon communication protocols and data formats) between these services are adhered to. Contract Testing is a testing approach that focuses on verifying that a service (the provider) meets the expectations of the services that consume it (the consumers). This ensures that both sides of the interaction agree on the data exchange, reducing the risk of integration issues when services evolve independently.
Contract testing ensures that:
- The provider service sends responses that match the expectations of the consumer.
- The consumer service makes requests that the provider can handle correctly.
Provider and Consumer Testing
Contract testing involves two main perspectives:
- Provider Testing:
- The provider is the service that offers an API for others to consume.
- The goal is to ensure that the provider’s API complies with the expectations documented in the contract.
- The provider tests its API against the contract to confirm that it returns the expected responses for given requests.
- Consumer Testing:
- The consumer is the service that calls the provider’s API.
- The goal is to ensure that the consumer sends requests that are valid according to the contract and that it can handle the responses it receives.
- The consumer tests its interaction with the API based on the contract, ensuring it only relies on the agreed-upon aspects of the API.
Tools and Implementation
To implement contract testing in microservices, several tools are available, with Pact being one of the most popular:
- Pact: A contract testing tool that helps automate the process of defining, verifying, and maintaining contracts between services. Pact works by allowing consumers to define their expectations in a contract, which the provider then verifies against its API.How Pact Works:
- The consumer service generates a contract (also known as a Pact) based on its expectations.
- The provider service then uses this contract to verify that it meets the consumer’s expectations.
- Pact provides tools for both generating and verifying these contracts, ensuring that any changes to the provider’s API are checked against consumer expectations.
Example: A simple contract test using Pact for a service that retrieves user data.
Consumer Side (Creating a Pact):
@ExtendWith(PactConsumerTestExt.class)
@PactTestFor(providerName = "UserService")
public class UserServiceConsumerTest {
@Pact(consumer = "OrderService")
public RequestResponsePact createPact(PactDslWithProvider builder) {
return builder
.given("User with ID 1 exists")
.uponReceiving("A request for User ID 1")
.path("/users/1")
.method("GET")
.willRespondWith()
.status(200)
.body(new PactDslJsonBody()
.stringType("name", "John Doe")
.stringType("email", "johndoe@example.com"))
.toPact();
}
@Test
@PactTestFor(pactMethod = "createPact")
public void testGetUser(MockServer mockServer) {
// Act
Response response = RestAssured.get(mockServer.getUrl() + "/users/1");
// Assert
assertEquals(200, response.getStatusCode());
assertEquals("John Doe", response.jsonPath().getString("name"));
}
}
Provider Side (Verifying the Pact):
@ExtendWith(PactProviderTestExt.class)
@Provider("UserService")
@PactFolder("pacts")
public class UserServiceProviderTest {
@TestTemplate
@ExtendWith(PactVerificationInvocationContextProvider.class)
public void verifyPact(PactVerificationContext context) {
context.verifyInteraction();
}
@State("User with ID 1 exists")
public void toUserExistsState() {
// Set up your service to return the expected user data.
}
}
Explanation:
- Consumer Test:
@Pact
: Defines the expected interaction, including the request path, method, and the expected response body.MockServer
: Simulates the provider service during the test.- The test ensures that the consumer can correctly handle the response it expects from the provider.
- Provider Test:
@Provider
: Specifies the service being tested.@PactFolder
: Indicates where the Pact files (contracts) are stored.- The provider test verifies that the service meets the expectations defined in the contract.
Benefits in Microservices
Contract testing offers several benefits in a microservices architecture:
- Reducing Integration Issues: By ensuring that contracts are adhered to, contract testing minimizes the risk of breaking changes that could disrupt communication between services. This is especially important when services are developed and deployed independently.
- Ensuring Smooth Communication Between Services: Contract tests ensure that both providers and consumers have a shared understanding of the API, leading to smoother interactions and fewer runtime errors.
- Early Detection of Issues: Contract tests can be run as part of the CI/CD pipeline, allowing teams to catch and address issues early in the development process before they reach production.
- Facilitating Independent Development: With contract testing, teams can confidently develop services in parallel, knowing that their interactions are validated through the contracts.
By incorporating contract testing into your microservices testing strategy, you can enhance the reliability and maintainability of your services, ensuring that they communicate effectively and consistently across the architecture.
4. End-to-End Testing
Definition and Scope
End-to-End (E2E) Testing is a testing strategy that focuses on validating the complete workflow of an application from start to finish. In the context of microservices architecture, this means testing the entire interaction between various services to ensure that they work together to deliver the expected functionality to the end user.
E2E testing goes beyond individual services and looks at the application as a whole, simulating real-world scenarios where multiple services interact with each other. The goal is to verify that all components of the system function correctly and that data flows seamlessly between services to deliver the desired outcome.
Example: An E2E test might involve a user placing an order on an e-commerce platform, which triggers interactions between services such as user authentication, order processing, payment, inventory management, and notification services.
Challenges in Microservices
E2E testing in a microservices architecture presents unique challenges due to the distributed nature of the system:
- Complexity: Microservices architectures often consist of numerous services, each with its own APIs, databases, and dependencies. Testing the entire workflow across these services can be complex and time-consuming.
- Data Flow Management: Ensuring that data flows correctly between services, particularly when there are asynchronous processes (e.g., message queues or event streams), can be difficult.
- Service Dependencies: Each service may depend on others, creating challenges in setting up and maintaining a consistent test environment that mimics production.
- Scalability: As the number of services grows, so does the complexity of E2E tests, which can lead to longer test execution times and more difficult troubleshooting when issues arise.
Best Practices
To effectively perform E2E testing in a microservices architecture, consider the following best practices:
- Prioritize Critical User Journeys:
- Focus E2E tests on the most critical user journeys that represent key business processes. This prioritization ensures that the most important workflows are thoroughly tested, even if not every possible interaction is covered.
- Example: For an online shopping platform, a critical user journey might include browsing products, adding items to the cart, and completing a purchase.
- Automate End-to-End Tests:
- Automating E2E tests helps ensure consistency and reliability. Automated tests can be integrated into the CI/CD pipeline, enabling continuous testing as new code is deployed.
- Automating tests also allows for more frequent execution, which helps catch issues early in the development process.
- Isolate Environments:
- Use dedicated test environments that closely mirror production to run E2E tests. This isolation prevents interference with production data and allows for controlled testing conditions.
- Test Resilience and Failure Scenarios:
- In a microservices architecture, services may fail independently. E2E tests should include scenarios that simulate service failures to ensure that the system can handle these situations gracefully.
- Example: Simulate a payment service outage and verify that the order service can handle the failure and provide appropriate feedback to the user.
Tools
Several tools can be used to automate and execute E2E tests in a microservices environment:
- Selenium:
- Selenium is a popular tool for automating web browsers, making it ideal for testing user interfaces in web applications.
- Example: Use Selenium to automate a user journey on an e-commerce site, verifying that the UI behaves correctly from login to checkout.
WebDriver driver = new ChromeDriver();
driver.get("https://www.example.com/login");
driver.findElement(By.id("username")).sendKeys("testuser");
driver.findElement(By.id("password")).sendKeys("password");
driver.findElement(By.id("loginButton")).click();
Assert.assertEquals(driver.getTitle(), "Dashboard");
Cypress:
- Cypress is a modern, JavaScript-based end-to-end testing framework that is particularly suited for testing modern web applications. It provides powerful features for writing, running, and debugging tests directly in the browser.
- Example: Write a Cypress test to verify that the order placement process works correctly
describe('Order Placement', () => {
it('should place an order successfully', () => {
cy.visit('/login');
cy.get('#username').type('testuser');
cy.get('#password').type('password');
cy.get('#loginButton').click();
cy.url().should('include', '/dashboard');
cy.visit('/products');
cy.get('.product').first().click();
cy.get('#addToCart').click();
cy.visit('/cart');
cy.get('#checkout').click();
cy.get('#paymentSuccess').should('exist');
});
});
3. Postman:
Postman is a versatile tool for API testing that can be used to test the interactions between services in a microservices architecture. It supports automated testing through its built-in scripting capabilities and can be integrated into CI/CD pipelines.
Example: Use Postman to create and run a collection of API requests that simulate an E2E workflow, such as creating a user, placing an order, and verifying payment status.
pm.test("Status code is 200", function () {
pm.response.to.have.status(200);
});
pm.test("Payment status is SUCCESS", function () {
var jsonData = pm.response.json();
pm.expect(jsonData.paymentStatus).to.eql("SUCCESS");
});
End-to-End testing is essential in microservices architectures to ensure that the entire system functions as expected from the user’s perspective. Despite the challenges of testing across multiple services, following best practices like prioritizing critical user journeys, automating tests, and using the right tools can help maintain the reliability and performance of your application. With tools like Selenium, Cypress, and Postman, you can create comprehensive E2E tests that verify not only the functionality but also the resilience of your microservices-based system.
5. Performance Testing
Importance in Microservices
Performance testing is crucial in a microservices architecture to ensure that each service performs optimally under various conditions, such as high user load, rapid scaling, or when subjected to stress. Unlike monolithic applications, where performance bottlenecks are often easier to identify, microservices architectures distribute functionality across multiple services, each with its own resource requirements and performance characteristics.
The importance of performance testing in microservices includes:
- Ensuring Scalability: Verifying that services can scale to handle increased load without degrading performance.
- Identifying Bottlenecks: Detecting service-level bottlenecks that could impact the overall system performance.
- Optimizing Resource Usage: Ensuring that each service uses system resources (CPU, memory, I/O) efficiently.
- Maintaining SLAs: Ensuring that services meet their performance-related Service Level Agreements (SLAs).
Approach to Performance Testing
Performance testing in microservices involves several types of tests, each addressing different aspects of performance:
- Load Testing:
- Load testing involves subjecting services to expected production loads to verify that they can handle the volume of traffic and data they will encounter in the real world.
- Example: Simulating 1000 users accessing a shopping cart service simultaneously to check response times and system behavior.
- Stress Testing:
- Stress testing goes beyond normal load conditions, pushing services to their limits to determine how they perform under extreme conditions, such as sudden spikes in traffic or resource exhaustion.
- Example: Gradually increasing the number of concurrent users on a payment processing service until it fails, to determine its breaking point.
- Scalability Testing:
- Scalability testing assesses how well a service scales in response to increasing demand. This involves testing how the service performs when additional resources (e.g., instances, CPU) are allocated.
- Example: Testing how quickly a microservice can scale from 2 instances to 10 instances during a flash sale event on an e-commerce platform.
Tools and Techniques
To effectively conduct performance testing in microservices, various tools and techniques can be used:
- JMeter:
- Apache JMeter is a widely-used open-source tool for load and performance testing. It allows you to simulate a large number of users and test how your services perform under different loads.
- Example: Creating a JMeter script to simulate thousands of requests per second to a microservice, measuring response times, and identifying potential bottlenecks.
<ThreadGroup>
<StringProp name="ThreadGroup.num_threads">100</StringProp>
<StringProp name="ThreadGroup.ramp_time">60</StringProp>
<HTTPSamplerProxy>
<stringProp name="HTTPSampler.domain">api.example.com</stringProp>
<stringProp name="HTTPSampler.path">/orders</stringProp>
<stringProp name="HTTPSampler.method">GET</stringProp>
</HTTPSamplerProxy>
</ThreadGroup>
Gatling:
- Gatling is another powerful tool for performance testing, particularly known for its high performance and ability to simulate complex user behaviors. It is written in Scala and provides a DSL for defining test scenarios.
- Example: Writing a Gatling script to test how well a search service handles 10,000 concurrent search requests.
val scn = scenario("Search Service Load Test")
.exec(http("Search Request")
.get("/search")
.queryParam("query", "test query")
.check(status.is(200)))
setUp(
scn.inject(atOnceUsers(10000))
).protocols(http.baseUrl("http://api.example.com"))
Strategies for Simulating Real-World Loads
When conducting performance testing, it’s important to simulate real-world loads as closely as possible to uncover potential issues before they affect users:
- Realistic Traffic Patterns:
- Use historical data or analytics to simulate realistic traffic patterns, such as peak usage times, geographic distribution of users, or seasonal traffic surges.
- Example: Simulating increased traffic during a Black Friday sale with a specific user distribution pattern.
- Data Variability:
- Simulate different types of data loads and variations in input sizes to test how well services handle diverse scenarios.
- Example: Testing how a microservice handles various sizes of payloads, such as small product catalogs versus large ones.
- Service Dependencies:
- Consider dependencies between services, simulating real-world conditions where one service’s load might impact another.
- Example: Testing an order service under high load while simultaneously stressing the inventory and payment services it depends on.
Challenges and Mitigation
Performance testing in a microservices environment comes with its own set of challenges:
- Distributed Environment:
- Microservices are often distributed across multiple servers or cloud instances, which can complicate performance testing. It’s essential to ensure that your test environment closely resembles your production environment.
- Mitigation: Use containerization tools like Docker and orchestration platforms like Kubernetes to replicate production-like environments for testing.
- Service-Level Bottlenecks:
- In a microservices architecture, a single underperforming service can create bottlenecks that affect the entire system.
- Mitigation: Identify and isolate bottlenecks by running targeted load tests on individual services, using monitoring and profiling tools to gather performance metrics.
- Data Consistency and Integrity:
- Ensuring data consistency across services during high-load scenarios can be challenging, particularly when dealing with distributed databases or eventual consistency models.
- Mitigation: Implement thorough validation checks and use distributed tracing to monitor data flow and consistency across services during performance tests.
Performance testing in a microservices architecture is essential to ensure that each service performs optimally under varying conditions, from normal loads to extreme stress scenarios. By employing tools like JMeter and Gatling, and following best practices for simulating real-world loads, you can identify and address performance bottlenecks before they impact your users. Despite the challenges posed by the distributed nature of microservices, careful planning, and the right strategies can help you maintain a high-performing, scalable, and resilient system.
6. Security Testing
Need for Security in Microservices
Security is paramount in a microservices architecture due to the distributed nature of the system. Each microservice may expose APIs, interact with other services, and manage sensitive data, making them potential targets for various vulnerabilities and attacks. Effective security testing is essential to protect microservices from threats such as:
- Unauthorized Access: Preventing unauthorized users from accessing or manipulating sensitive data.
- Data Breaches: Ensuring that data exchanged between services and stored within them is protected from unauthorized access.
- Injection Attacks: Protecting against attacks that exploit vulnerabilities in input handling.
- Denial of Service (DoS): Mitigating risks of services being overwhelmed by malicious traffic.
Approach and Tools
Security testing involves identifying and addressing potential vulnerabilities within each microservice and the interactions between them. Several tools and approaches can help with this process:
- OWASP ZAP (Zed Attack Proxy):
- Overview: OWASP ZAP is an open-source security tool designed for finding vulnerabilities in web applications. It is useful for both automated and manual security testing.
- Features: Automated scanners, passive scanning, and active scanning for various vulnerabilities.
- Example Usage:
- Run an automated scan on a microservice API to detect common vulnerabilities.
- Use the ZAP Proxy to intercept and analyze traffic between services to identify potential security issues.
zap-cli start
zap-cli quick-scan http://api.example.com
Burp Suite:
- Overview: Burp Suite is a comprehensive suite of tools for web application security testing. It includes features for scanning, crawling, and analyzing web applications.
- Features: Intruder for attack simulations, Scanner for vulnerability detection, and Repeater for manual testing.
- Example Usage:
- Configure Burp Suite to intercept requests and responses between microservices to identify vulnerabilities.
- Use the Scanner to perform automated vulnerability assessments on your APIs.
burpsuite -D
Testing for Common Vulnerabilities
In microservices architectures, several common vulnerabilities should be addressed through security testing:
Testing for Common Vulnerabilities
In microservices architectures, several common vulnerabilities should be addressed through security testing:
- 1. SQL Injection:
- Description: Occurs when an attacker can manipulate SQL queries by injecting malicious input, potentially compromising data integrity.
- Testing: Use automated tools or manual techniques to input SQL injection payloads into query parameters and validate if the service is vulnerable.
SELECT * FROM users WHERE username = 'admin' OR '1'='1';
2. Cross-Site Scripting (XSS):
- Description: XSS vulnerabilities allow attackers to inject malicious scripts into web pages, which can be executed in the context of other users.
- Testing: Inject JavaScript payloads into form fields or URL parameters and check if the script executes.
<script>alert('XSS');</script>
- 3. Cross-Site Request Forgery (CSRF):
- Description: CSRF attacks trick a user into performing actions on a web application without their consent.
- Testing: Verify that your microservices implement CSRF protection mechanisms, such as tokens or headers.
- 4. Broken Authentication:
- Description: Vulnerabilities in authentication mechanisms can allow unauthorized access to services.
- Testing: Check for weaknesses in login processes, password management, and session handling.
Best Practices
To ensure robust security in a microservices environment, incorporate these best practices into your security testing strategy:
- Incorporating Security Testing into CI/CD Pipelines:
- Continuous Integration/Continuous Deployment (CI/CD) pipelines should include security testing steps to identify vulnerabilities early in the development cycle.
- Integrate security testing tools into your CI/CD pipeline to automate vulnerability assessments and ensure that security issues are addressed before deployment.
- Use Jenkins or GitHub Actions to run security scans with OWASP ZAP or Burp Suite during the build process.
- Regularly Updating Security Measures:
- Stay Current: Regularly update your security tools and libraries to protect against new vulnerabilities and threats.
- Patch Management: Apply security patches and updates to your services, dependencies, and infrastructure promptly.
- Vulnerability Management: Continuously monitor and address vulnerabilities as they are discovered, using threat intelligence and security advisories.
- Implementing Secure Coding Practices:
- Validation and Sanitization: Ensure that all inputs are validated and sanitized to prevent injection attacks.
- Authentication and Authorization: Implement strong authentication mechanisms and enforce least privilege principles for access control.
- Conducting Regular Security Audits:
- Periodic Reviews: Perform regular security audits and penetration tests to identify and address potential vulnerabilities in your microservices.
- Compliance: Ensure that your security practices comply with relevant regulations and standards (e.g., GDPR, HIPAA).
Security testing is a critical component of maintaining a secure microservices architecture. By utilizing tools like OWASP ZAP and Burp Suite, testing for common vulnerabilities, and following best practices such as integrating security testing into CI/CD pipelines and regularly updating security measures, you can effectively protect your services from threats and ensure that your microservices ecosystem remains robust and resilient.
7. Monitoring and Observability
Importance in Microservices
In a microservices architecture, continuous monitoring and observability are critical for maintaining the health and performance of the system. Given the distributed nature of microservices, it can be challenging to identify and diagnose issues that span multiple services. Effective monitoring and observability help ensure that:
- Issues Are Detected Early: Continuous monitoring allows you to detect and address issues in real time before they impact end users.
- Performance Is Optimized: Observability helps identify performance bottlenecks and inefficiencies, enabling optimization efforts.
- System Health Is Maintained: Regular monitoring of key metrics ensures that the overall health of the system is maintained and any deviations are addressed promptly.
Key Metrics to Monitor
To effectively monitor a microservices environment, focus on the following key metrics:
- Latency:
- Definition: The time taken for a request to be processed by a service. High latency can indicate performance issues or bottlenecks.
- Example: Track request and response times for each microservice to ensure they meet performance benchmarks.
- Error Rates:
- Definition: The frequency of errors occurring in a service, such as HTTP 5xx errors or exceptions.
- Example: Monitor error rates to identify services experiencing failures or misconfigurations.
- Service Health:
- Definition: The overall health and availability of a service, including uptime and resource usage.
- Example: Use health checks and status endpoints to track whether services are running and responding as expected.
Tools and Strategies
Several tools and strategies can be employed to achieve effective monitoring and observability in a microservices architecture:
- Prometheus:
- Overview: Prometheus is an open-source monitoring and alerting toolkit designed for reliability and scalability. It collects metrics from configured endpoints at specified intervals and stores them in a time-series database.
- Usage:
- Set up Prometheus to scrape metrics from your microservices and store them for analysis.
- Use Prometheus queries to create dashboards and alerts based on your metrics.
scrape_configs:
- job_name: 'microservices'
static_configs:
- targets: ['service1:9090', 'service2:9090']
Grafana:
- Overview: Grafana is a powerful open-source analytics and monitoring platform that integrates with Prometheus and other data sources to create dashboards and visualizations.
- Usage:
- Use Grafana to visualize metrics collected by Prometheus, creating dashboards that provide insights into system performance and health.
Example Dashboard Setup:
- Create panels to display metrics such as request latency, error rates, and system resource usage.
- Set up alerts in Grafana based on thresholds for key metrics.
ELK Stack (Elasticsearch, Logstash, Kibana):
- Overview: The ELK Stack is a popular set of tools for log management and analysis. Elasticsearch is a search and analytics engine, Logstash is a log pipeline tool, and Kibana is a visualization and dashboard tool.
- Usage:
- Collect logs from your microservices using Logstash, index them in Elasticsearch, and visualize them in Kibana.
- Use Kibana to search, analyze, and visualize logs to troubleshoot issues and gain insights.
Example Logstash Configuration:
input {
file {
path => "/var/log/microservices/*.log"
start_position => "beginning"
}
}
filter {
json {
source => "message"
}
}
output {
elasticsearch {
hosts => ["http://localhost:9200"]
index => "microservices-logs"
}
}
Distributed Tracing:
- Overview: Distributed tracing helps track the flow of requests across multiple services, providing insights into how different parts of the system interact and where delays or failures occur.
- Tools: Use tools like Jaeger or Zipkin for distributed tracing.
- Usage:
- Implement tracing in your microservices to capture and visualize end-to-end request flows.
- Analyze traces to identify performance bottlenecks and troubleshoot issues.
Example Jaeger Configuration:
service:
name: my-service
tags:
- key: "env"
value: "production"
Reactive Testing
Reactive testing involves adapting your testing strategies based on insights gained from monitoring and observability tools. This approach helps ensure that testing remains relevant and effective as your system evolves. Key aspects include:
- Adjusting Test Scenarios:
- Use data from monitoring tools to identify new test scenarios or adjust existing ones based on real-world usage patterns and observed issues.
- Example: If monitoring shows high latency in a particular service, create performance tests specifically targeting that service.
- Triggering Tests Based on Alerts:
- Integrate monitoring alerts with your testing processes to trigger additional tests when specific conditions are met.
- Example: If an alert is triggered due to increased error rates, run a series of integration and load tests to diagnose and address the issue.
- Continuous Improvement:
- Continuously review monitoring data and performance metrics to refine and improve testing strategies, ensuring they align with the latest insights and system changes.
Effective monitoring and observability are crucial for maintaining the health and performance of a microservices architecture. By focusing on key metrics such as latency, error rates, and service health, and utilizing tools like Prometheus, Grafana, and the ELK Stack, you can gain valuable insights into your system. Implementing distributed tracing further enhances your ability to diagnose and resolve issues. Reactive testing based on monitoring insights ensures that your testing strategies evolve in response to real-world conditions, contributing to a more robust and reliable microservices ecosystem.
Conclusion
In a microservices architecture, robust monitoring and observability are essential for maintaining system reliability and performance. The distributed nature of microservices introduces complexities that necessitate continuous oversight of key metrics such as latency, error rates, and service health. By leveraging tools like Prometheus, Grafana, and the ELK Stack, you can effectively collect, visualize, and analyze metrics and logs, enabling you to detect and address issues in real time.
Implementing distributed tracing provides deeper insights into the flow of requests across services, helping you pinpoint performance bottlenecks and troubleshoot complex issues. Reactive testing, driven by the insights gained from monitoring and observability tools, ensures that your testing strategies are responsive to real-world conditions and evolving system requirements.
By integrating comprehensive monitoring and observability practices into your microservices architecture, you enhance your ability to maintain system health, optimize performance, and deliver a reliable user experience. This proactive approach not only aids in identifying and resolving issues swiftly but also supports continuous improvement and adaptability in your microservices ecosystem.