In today’s fast-paced software development landscape, enterprises demand agility, scalability, and robustness in their applications. Spring Framework has emerged as a vital tool in meeting these demands. Its comprehensive ecosystem offers a one-stop solution for building enterprise applications, enabling developers to manage everything from web development and security to data access and transaction management.
One of the key reasons Spring remains crucial in modern Java development is its ability to integrate seamlessly with various technologies and frameworks. Whether you’re working with Hibernate for ORM, Apache Kafka for messaging, or Angular for frontend development, Spring’s integration capabilities ensure that all these technologies work harmoniously together.
Moreover, Spring’s focus on best practices, such as dependency injection and aspect-oriented programming, encourages developers to write clean, maintainable, and testable code. This not only reduces technical debt but also accelerates the development process, making it easier to meet tight deadlines without compromising on quality.
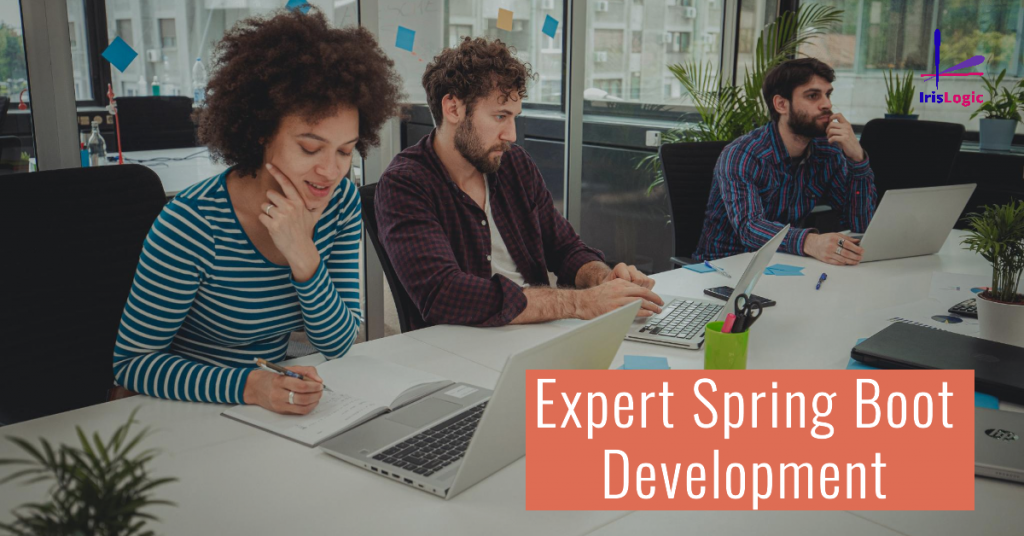
Simplifying Spring with Spring Boot
While Spring offered unparalleled flexibility and power, it also came with a steep learning curve, particularly for newcomers. Configuring Spring applications often required extensive XML configurations, which could be cumbersome and error-prone. Enter Spring Boot.
Spring Boot, introduced in 2014, was designed to simplify the development of Spring applications by offering a convention-over-configuration approach. With Spring Boot, developers can get a Spring application up and running with minimal configuration, thanks to its opinionated defaults and extensive use of annotations.
One of the standout features of Spring Boot is its ability to embed application servers like Tomcat, Jetty, or Undertow, allowing developers to package and deploy their applications as standalone JAR files. This eliminates the need for complex deployment processes, making it easier to build and deploy microservices.
Furthermore, Spring Boot’s auto-configuration feature intelligently configures the application based on the dependencies present in the classpath, reducing the need for manual setup. This, coupled with a vast ecosystem of “starters,” enables developers to integrate various technologies quickly and efficiently, from databases and messaging systems to cloud platforms.
In essence, Spring Boot has democratized Spring development, making it accessible to a broader range of developers while retaining the power and flexibility that made Spring a favorite among enterprise developers. It has become the de facto standard for modern Spring-based applications, enabling rapid development, streamlined deployment, and easier maintenance.
1. Core Concepts of Spring Framework
Inversion of Control (IoC)
Inversion of Control (IoC) is a foundational principle of the Spring Framework, representing a paradigm shift from traditional procedural programming. In a typical application, the flow of control is dictated by the code, with objects creating and managing their dependencies. However, in IoC, this control is inverted, meaning the framework takes over the responsibility of managing object lifecycles and their dependencies.
IoC allows developers to focus on writing business logic without worrying about the instantiation and management of dependencies. In Spring, this is primarily achieved through Dependency Injection (DI), where objects are provided with their dependencies by an external entity, typically the Spring container.
Dependency Injection (DI)
Dependency Injection (DI) is the mechanism through which IoC is implemented in Spring. DI allows the injection of dependencies into an object at runtime, rather than at compile-time, enabling greater flexibility and decoupling of components.
Spring supports three types of DI:
- Constructor Injection: Dependencies are provided through a class constructor.
- Setter Injection: Dependencies are provided through setter methods.
- Field Injection: Dependencies are injected directly into fields using annotations like
@Autowired
.
Constructor and setter injection are generally preferred due to their testability and clarity, while field injection is often discouraged in favor of the other two approaches.
Example:
@Component
public class MyService {
private final MyRepository repository;
@Autowired
public MyService(MyRepository repository) {
this.repository = repository;
}
}
Here, MyRepository
is injected into MyService
via constructor injection.
ApplicationContext vs BeanFactory
In Spring, the ApplicationContext and BeanFactory are the two main interfaces for accessing the Spring IoC container. While both can manage and configure beans, ApplicationContext is a superset of BeanFactory, offering additional features and capabilities.
BeanFactory is the most basic container, providing fundamental DI capabilities. It lazily loads beans, meaning they are instantiated only when needed.
ApplicationContext, on the other hand, eagerly loads all singleton beans during startup, providing advanced features such as:
- Event propagation
- Declarative mechanisms to create a bean
- AOP integration
- Internationalization (i18n) support
In most cases, developers prefer using ApplicationContext
because of these additional capabilities, which are critical in enterprise applications.
Aspect-Oriented Programming (AOP)
Aspect-Oriented Programming (AOP) is another core concept in Spring, allowing developers to separate cross-cutting concerns from the business logic. Cross-cutting concerns like logging, security, and transaction management are aspects of the application that often cut across multiple modules, leading to code tangling and scattering.
With AOP, these concerns are modularized into separate aspects, making the core business logic cleaner and more maintainable.
Cross-Cutting Concerns
Cross-cutting concerns refer to functionalities that affect multiple parts of an application and cannot be cleanly encapsulated within a single module. Typical examples include:
- Logging: Capturing logs across various modules.
- Security: Implementing consistent security checks across the application.
- Transaction Management: Ensuring consistent transaction handling across data operations.
Implementing AOP in Spring with Practical Examples
Spring provides robust support for AOP through its @AspectJ
annotation-driven approach. Here’s a basic example:
@Aspect
@Component
public class LoggingAspect {
@Before("execution(* com.example.service.*.*(..))")
public void logBeforeMethod(JoinPoint joinPoint) {
System.out.println("Executing method: " + joinPoint.getSignature().getName());
}
}
In this example, @Before
advice is used to log the execution of any method within the com.example.service
package.
Spring MVC Architecture
Spring MVC (Model-View-Controller) is a framework within Spring for building web applications. It follows the traditional MVC pattern, which divides the application into three interconnected components:
- Model: Represents the application’s data and business logic.
- View: Handles the presentation layer, rendering the UI.
- Controller: Manages the flow of the application, handling user input and updating the model.
Front Controller Design Pattern
Spring MVC uses the Front Controller design pattern, which centralizes request handling. The DispatcherServlet
acts as the front controller in Spring MVC, intercepting all incoming HTTP requests and routing them to appropriate handlers/controllers.
Handler Mapping, Controller, View Resolver
- Handler Mapping: Maps incoming requests to corresponding controllers based on URL patterns or annotations.
- Controller: Handles the request, processes it, and returns a
ModelAndView
object. - View Resolver: Resolves the view (e.g., JSP, Thymeleaf) to be rendered, based on the logical view name returned by the controller.
Example:
@Controller
public class MyController {
@RequestMapping("/greet")
public String greet(Model model) {
model.addAttribute("message", "Hello, World!");
return "greeting";
}
}
In this example, the greet
method handles requests to /greet
, adding a message to the model and returning the logical view name greeting
.
Spring Security
Spring Security is a powerful and highly customizable authentication and access-control framework. It provides comprehensive security services for Java applications, including web security, method-level security, and even integration with OAuth2 for securing REST APIs.
Authentication and Authorization Mechanisms
- Authentication: Verifies the identity of a user. Spring Security provides several options, including basic authentication, form-based login, and integration with third-party authentication providers.
- Authorization: Determines whether an authenticated user has access to specific resources. Spring Security uses roles and authorities to manage this, where users are granted roles, and these roles define their authorities.
Customizing Spring Security
Spring Security can be customized extensively using Java-based configuration. Here’s a simple example:
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth.inMemoryAuthentication()
.withUser("user").password("{noop}password").roles("USER")
.and()
.withUser("admin").password("{noop}admin").roles("ADMIN");
}
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.antMatchers("/admin/**").hasRole("ADMIN")
.antMatchers("/**").permitAll()
.and()
.formLogin();
}
}
This configuration defines two in-memory users and secures the application, restricting access to /admin/**
URLs to users with the ADMIN
role.
Spring Data Access
Spring simplifies data access in Java applications through various abstractions and integrations, ensuring a consistent approach regardless of the underlying data source.
Spring JDBC, Hibernate with Spring, and JPA Integration
- Spring JDBC: Provides a simpler approach to working with relational databases using JDBC, offering utility classes to avoid boilerplate code.
- Hibernate with Spring: Integrates Hibernate, a popular ORM framework, with Spring, managing the session factory and transaction management.
- JPA (Java Persistence API): Spring provides support for JPA, allowing developers to use any JPA-compliant ORM tool like Hibernate, EclipseLink, or OpenJPA.
Example using Spring Data JPA:
public interface MyRepository extends JpaRepository<MyEntity, Long> {
List<MyEntity> findByName(String name);
}
This MyRepository
interface automatically provides CRUD operations and custom query methods without requiring implementation.
Transaction Management
Transaction Management in Spring ensures data integrity and consistency across multiple operations. Spring supports both declarative and programmatic transaction management.
- Declarative Transactions: Managed through annotations like
@Transactional
, providing a clean and readable approach. - Programmatic Transactions: Managed explicitly in the code, offering more control but at the cost of additional complexity.
Example:
@Service
public class MyService {
@Transactional
public void performTransactionalOperation() {
// Perform operations here
}
}
In this example, the performTransactionalOperation
method is automatically wrapped in a transaction, ensuring that all operations within it either complete successfully or are rolled back in case of an error.
This technical content provides an in-depth look at the core concepts of the Spring Framework, offering practical examples to demonstrate the power and flexibility of Spring in modern Java development.
2. Spring Boot Overview
Convention over Configuration
One of the defining philosophies of Spring Boot is “Convention over Configuration.” This approach simplifies the development process by reducing the need for extensive manual configuration. Traditionally, setting up a Spring application required a significant amount of XML or Java-based configuration to wire up components. With Spring Boot, many of these configurations are handled automatically, allowing developers to focus more on building features and less on setup.
Spring Boot achieves this through sensible defaults, meaning it provides pre-configured setups based on common use cases. For instance, if you’re building a web application, Spring Boot will automatically configure a web server, a dispatcher servlet, and other necessary components. You can override these defaults when needed, but in many cases, the out-of-the-box configuration is sufficient to get your application up and running quickly.
Auto-configuration and Spring Boot Starters
Auto-configuration is a key feature of Spring Boot that further reduces the need for manual setup. Spring Boot’s auto-configuration mechanism automatically configures your application based on the dependencies present in your project’s classpath. This means that when you add a dependency (e.g., for a database or web server), Spring Boot automatically configures the necessary beans and components for you.
For example, if you include the H2 database dependency in your project, Spring Boot will auto-configure a connection to an in-memory H2 database, along with a DataSource
and JdbcTemplate
bean, without any additional setup required from you.
Complementing auto-configuration are Spring Boot Starters. Starters are a set of pre-configured dependencies that you can include in your project to quickly get started with a specific functionality. For instance:
spring-boot-starter-web
sets up a Spring MVC application with a web server.spring-boot-starter-data-jpa
configures JPA and Hibernate with a default database.spring-boot-starter-security
sets up Spring Security for your application.
These starters simplify dependency management by bundling the necessary libraries and configurations for common tasks, making it easier to get started and reducing the likelihood of configuration errors.
Embedded Servers
Spring Boot simplifies the process of deploying applications by providing embedded servers. Unlike traditional Java web applications that require a separate application server like Tomcat or Jetty, Spring Boot allows you to embed these servers directly into your application. This means you can package your entire application, along with the server, into a single executable JAR file.
The most commonly used embedded servers with Spring Boot are:
- Tomcat: The default server in Spring Boot, widely used and robust.
- Jetty: Known for its small footprint and flexibility.
- Undertow: A lightweight and high-performance server.
Pros and Cons of Using Embedded Servers
Pros:
- Simplified Deployment: Embedding the server within the application eliminates the need for external application servers, streamlining the deployment process.
- Consistency: Ensures that the application behaves the same way in different environments (e.g., development, testing, production) since the server configuration is bundled with the application.
- Portability: The entire application can be run on any system with Java installed, as the server is included in the package.
Cons:
- Increased Application Size: Bundling the server with the application increases the overall size of the application artifact.
- Limited Server Features: While embedded servers are sufficient for most use cases, they might not support some advanced features or configurations available in a full-fledged application server.
- Single Point of Failure: Embedding the server within the application means that a server failure could potentially bring down the entire application.
Spring Boot Annotations
Spring Boot makes extensive use of annotations to simplify development and configuration. Some of the most commonly used annotations include:
@SpringBootApplication
: This is a convenience annotation that combines@Configuration
,@EnableAutoConfiguration
, and@ComponentScan
. It marks the main class of a Spring Boot application, enabling auto-configuration and component scanning.- Example:
@SpringBootApplication
public class MyApplication {
public static void main(String[] args) {
SpringApplication.run(MyApplication.class, args);
}
}
@Component
: Marks a Java class as a Spring component, making it a candidate for auto-detection and dependency injection.
@Service
: A specialization of @Component
, used to annotate service classes that contain business logic.
@Repository
: Another specialization of @Component
, used to annotate classes that interact with the database, particularly DAOs (Data Access Objects).
@RestController
: Combines @Controller
and @ResponseBody
, used to create RESTful web services. It indicates that the class handles web requests and the returned value is bound to the web response body.
Example:
@RestController
public class MyController {
@GetMapping("/greet")
public String greet() {
return "Hello, World!";
}
}
These annotations, along with many others, provide a declarative way to configure and manage various components in a Spring Boot application, leading to cleaner, more readable code.
Profiles and Properties
Spring Boot allows you to configure your application for different environments using profiles and properties. This feature makes it easy to define environment-specific settings, such as database connections, API keys, or logging levels.
Environment-Specific Configurations
Profiles in Spring Boot enable you to define different configurations for different environments (e.g., development, testing, production). By activating a specific profile, you can ensure that only the relevant configuration is used in that environment.
Profiles can be activated via:
- Command Line: Using the
--spring.profiles.active
parameter when starting the application. - Application Properties/YAML: Defining the profile in the configuration file.
Example of profile-specific configurations in a properties file:
# application.properties
spring.datasource.url=jdbc:h2:mem:testdb
# application-dev.properties
spring.datasource.url=jdbc:mysql://localhost:3306/devdb
# application-prod.properties
spring.datasource.url=jdbc:mysql://localhost:3306/proddb
In this example, the appropriate database URL is selected based on the active profile.
Using YAML and Properties Files
Spring Boot supports configuration through both properties files (application.properties
) and YAML files (application.yml
). YAML offers a more hierarchical structure, making it easier to manage complex configurations.
Example using a YAML file:
spring:
datasource:
url: jdbc:mysql://localhost:3306/mydb
username: user
password: pass
server:
port: 8080
YAML files are particularly useful for configuring nested properties, which can be cumbersome in traditional properties files. Both formats can coexist in a Spring Boot project, with YAML often being preferred for more complex configurations.
3. Advanced Spring Boot Features
Customizing Auto-Configuration
While Spring Boot’s auto-configuration is incredibly powerful, there are scenarios where the default configurations may not suit your specific needs. In such cases, you can customize auto-configuration to tailor the application to your requirements.
How to Create Custom Auto-Configurations
Creating custom auto-configurations involves defining your own configuration classes that will be picked up and used by Spring Boot instead of, or in addition to, the default configurations.
- 1. Create a Configuration Class: Annotate your class with
@Configuration
to indicate that it’s a source of bean definitions. - Example:
@Configuration
public class MyCustomConfig {
@Bean
public MyService myService() {
return new MyServiceImpl();
}
}
2. Conditionally Enable Configurations: Use conditional annotations like @ConditionalOnMissingBean
, @ConditionalOnProperty
, or @ConditionalOnClass
to control when your configuration should be applied.
Example:
@Configuration
@ConditionalOnClass(MyService.class)
public class MyConditionalConfig {
@Bean
public MyService anotherService() {
return new AnotherServiceImpl();
}
}
3. Exclude Auto-Configurations: You can also exclude specific auto-configurations provided by Spring Boot if they conflict with your custom setup. This can be done using the @SpringBootApplication
annotation.
Example:
@SpringBootApplication(exclude = {DataSourceAutoConfiguration.class})
public class MyApplication {
public static void main(String[] args) {
SpringApplication.run(MyApplication.class, args);
}
}
Custom auto-configurations give you granular control over the behavior of your Spring Boot application, ensuring that it aligns perfectly with your project’s needs.
Spring Boot Actuator
Spring Boot Actuator provides a set of production-ready features that help you monitor and manage your Spring Boot application. Actuator includes several built-in endpoints that expose information about your application’s health, metrics, and configuration.
Monitoring and Managing Spring Boot Applications
Actuator endpoints can be accessed via HTTP, JMX, or even SSH, providing a flexible way to interact with your running application. Some of the most commonly used endpoints include:
/actuator/health
: Provides a health status of the application. This can include database connectivity, disk space, and custom health indicators.- Example:
{
"status": "UP",
"components": {
"db": {
"status": "UP",
"details": {
"database": "H2",
"validationQuery": "isValid()"
}
},
"diskSpace": {
"status": "UP",
"details": {
"total": 499963174912,
"free": 104353050624,
"threshold": 10485760
}
}
}
}
/actuator/metrics
: Exposes various metrics related to the JVM, system, and application (e.g., memory usage, request counts, and custom application metrics).
Example:
{
"names": [
"jvm.memory.used",
"jvm.memory.max",
"http.server.requests",
"process.uptime"
]
}
Exposing Metrics, Health Checks, and Custom Endpoints
You can customize which Actuator endpoints are enabled or disabled via your application.properties
or application.yml
file. You can also create custom endpoints by implementing the Endpoint
interface, allowing you to expose additional application-specific information.
Example of enabling specific endpoints:
management.endpoints.web.exposure.include=health,info,metrics
Creating a custom endpoint:
@Component
@Endpoint(id = "customEndpoint")
public class CustomEndpoint {
@ReadOperation
public String customOperation() {
return "Custom endpoint response";
}
}
Spring Boot Actuator is a powerful tool for keeping your application healthy and performing well in production, offering insights and control over the application’s runtime behavior.
Spring Boot Testing
Testing is an integral part of any software development process, and Spring Boot provides several utilities to make testing easier and more efficient.
Testing Strategies in Spring Boot
- 1. Unit Testing: Focuses on testing individual components in isolation. Spring Boot supports unit testing with popular testing frameworks like JUnit and TestNG.Example of a simple unit test:
@SpringBootTest
public class MyServiceTest {
@Autowired
private MyService myService;
@Test
public void testServiceMethod() {
assertEquals("expectedValue", myService.someMethod());
}
}
2. Integration Testing: Spring Boot simplifies integration testing by providing @SpringBootTest
, which starts the full application context, allowing you to test how various components work together.
Example of an integration test:
@RunWith(SpringRunner.class)
@SpringBootTest(webEnvironment = SpringBootTest.WebEnvironment.RANDOM_PORT)
public class MyControllerIntegrationTest {
@Autowired
private TestRestTemplate restTemplate;
@Test
public void testControllerEndpoint() {
ResponseEntity<String> response = restTemplate.getForEntity("/endpoint", String.class);
assertEquals(HttpStatus.OK, response.getStatusCode());
assertEquals("expectedResponse", response.getBody());
}
}
3. Mocking: You can mock dependencies using libraries like Mockito, allowing you to isolate the component under test without relying on the actual implementations of its dependencies.
Example of using Mockito:
@RunWith(MockitoJUnitRunner.class)
public class MyServiceTest {
@Mock
private Dependency dependency;
@InjectMocks
private MyService myService;
@Test
public void testWithMockedDependency() {
when(dependency.someMethod()).thenReturn("mockedValue");
assertEquals("mockedValue", myService.callDependency());
}
}
4. TestRestTemplate: Specifically designed for testing RESTful services, TestRestTemplate
provides an easy way to make HTTP requests and verify responses in your tests.
Example:
@Autowired
private TestRestTemplate restTemplate;
@Test
public void testRestEndpoint() {
ResponseEntity<String> response = restTemplate.getForEntity("/api/test", String.class);
assertEquals(HttpStatus.OK, response.getStatusCode());
assertEquals("Hello, World!", response.getBody());
}
Spring Boot’s testing support ensures that your application is robust, reliable, and free from regression issues.
Spring Boot with Docker
As applications are increasingly being deployed in containerized environments, understanding how to containerize a Spring Boot application with Docker is essential.
Containerizing Spring Boot Applications
To Dockerize a Spring Boot application, you’ll typically follow these steps:
- 1. Create a Dockerfile: This file contains instructions for packaging your application into a Docker container.
- Example Dockerfile:
FROM openjdk:17-jdk-alpine
VOLUME /tmp
ARG JAR_FILE=target/myapp.jar
COPY ${JAR_FILE} app.jar
ENTRYPOINT ["java","-jar","/app.jar"]
2. Build the Docker Image: Use the Docker CLI to build the image from your Dockerfile.
Command:
docker build -t myapp .
3. Run the Docker Container: Start your application in a Docker container.
Command:
docker run -p 8080:8080 myapp
Best Practices for Dockerizing Spring Boot Apps
- Use a Multi-Stage Build: Reduce the size of your Docker image by using a multi-stage build process, where the first stage compiles the application, and the second stage packages only the necessary files.
- Example:
# Stage 1: Build
FROM maven:3.8.1-jdk-11 AS build
COPY src /usr/src/app/src
COPY pom.xml /usr/src/app
RUN mvn -f /usr/src/app/pom.xml clean package
# Stage 2: Package
FROM openjdk:11-jre-slim
COPY --from=build /usr/src/app/target/myapp.jar /usr/app/myapp.jar
ENTRYPOINT ["java","-jar","/usr/app/myapp.jar"]
- Use Environment Variables for Configuration: Instead of hardcoding configurations, use environment variables to pass settings to your Dockerized application.
- Keep Your Image Small: Use a minimal base image like
alpine
to reduce the image size and improve startup times. - Leverage Docker Compose: For multi-container applications, use Docker Compose to define and run your entire stack (e.g., Spring Boot app, database, etc.) as a single service.
Microservices with Spring Boot
Spring Boot is widely used for building microservices, thanks to its lightweight nature and ease of configuration.
Building Microservices with Spring Boot
A typical microservices architecture involves breaking down an application into smaller, loosely coupled services that can be developed, deployed, and scaled independently. Spring Boot simplifies the creation of microservices with its comprehensive set of tools and frameworks.
Key elements in building microservices with Spring Boot include:
- **REST
ful APIs**: Implementing RESTful services using Spring MVC’s @RestController
.
Example:
@RestController
@RequestMapping("/api")
public class MyMicroserviceController {
@GetMapping("/data")
public ResponseEntity<String> getData() {
return ResponseEntity.ok("Data from microservice");
}
}
- Service Discovery: Use Spring Cloud’s Eureka for service registration and discovery, enabling microservices to find and communicate with each other.
- API Gateway: Use Spring Cloud’s Zuul or Spring Cloud Gateway to route requests to the appropriate microservices, providing a unified entry point.
- Load Balancing: Use Spring Cloud’s Ribbon or Spring Cloud LoadBalancer to distribute requests across multiple instances of a microservice.
Spring Cloud for Microservices Architecture
Spring Cloud provides a suite of tools for building robust microservices architectures:
- Eureka: A service registry and discovery service that allows microservices to register themselves and discover other services.
- Example configuration:
eureka.client.service-url.defaultZone=http://localhost:8761/eureka/
Zuul: An API gateway that provides dynamic routing, monitoring, resiliency, and security for your microservices.
Example configuration:
zuul.routes.myservice.path=/myservice/**
zuul.routes.myservice.service-id=myservice
Ribbon: A client-side load balancer that provides control over the behavior of HTTP requests, helping distribute load across service instances.
Example:
ribbon.eureka.enabled=true
Hystrix: A library for handling latency and fault tolerance, ensuring that your microservices architecture remains resilient in the face of failures.
Example configuration:
hystrix.command.default.execution.isolation.thread.timeoutInMilliseconds=1000
Spring Boot and Spring Cloud together offer a comprehensive solution for building, deploying, and managing microservices, allowing for scalable, maintainable, and resilient applications.
4. Performance Optimization in Spring and Spring Boot
Performance optimization is crucial for any application to ensure responsiveness, scalability, and efficient resource utilization. In Spring and Spring Boot, several strategies and tools are available to enhance application performance. This section covers caching, asynchronous processing, and reactive programming to help you optimize your Spring-based applications.
Caching with Spring
Caching can significantly improve the performance of your application by storing frequently accessed data in memory, reducing the need for repeated computations or database queries. Spring provides a comprehensive caching abstraction that allows you to easily integrate various caching solutions.
Caching Strategies and Implementation
- 1. Simple Caching: Spring offers a straightforward caching abstraction using the
@Cacheable
annotation. This annotation tells Spring to cache the result of a method call based on its parameters. - Example:
@Service
public class MyService {
@Cacheable("items")
public Item getItemById(Long id) {
// Expensive operation to fetch item
return itemRepository.findById(id).orElse(null);
}
}
In this example, the result of getItemById
will be cached with the cache name “items”. If the same id
is requested again, the result will be retrieved from the cache instead of querying the database.
2. Cache Configuration: You can configure various cache providers such as EhCache, Redis, or Caffeine in your application.properties
or application.yml
file.
Example configuration for EhCache:
spring.cache.ehcache.config=classpath:ehcache.xml
Example EhCache configuration file (ehcache.xml
):
<ehcache xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.ehcache.org/v3 http://www.ehcache.org/v3/xml/ehcache-v3.xsd"
xmlns="http://www.ehcache.org/v3">
<cache alias="items">
<key-type>java.lang.Long</key-type>
<value-type>com.example.Item</value-type>
<expiry>
<ttl unit="minutes">10</ttl>
</expiry>
<resources>
<heap unit="MB">100</heap>
</resources>
</cache>
</ehcache>
3. Cache Eviction: Use @CacheEvict
to remove entries from the cache when they become stale. This annotation can be used to evict cache entries or clear the entire cache.
Example:
@CacheEvict(value = "items", allEntries = true)
public void clearCache() {
// Method to clear cache
}
4. Cache Put: Use @CachePut
to update the cache without interfering with the method execution.
Example:
@CachePut(value = "items", key = "#item.id")
public Item updateItem(Item item) {
return itemRepository.save(item);
}
Caching can greatly enhance performance, especially in applications with expensive data retrieval operations or frequent reads.
Asynchronous Processing
Asynchronous Processing allows your application to perform tasks in the background, improving responsiveness and throughput. Spring simplifies asynchronous programming with the @Async
annotation.
@Async
Annotation and Its Use Cases
- 1. Enabling Asynchronous Processing: To use the
@Async
annotation, you need to enable asynchronous processing in your configuration class using@EnableAsync
. - Example:
@Configuration
@EnableAsync
public class AppConfig {
}
2. Using @Async
: Annotate methods with @Async
to execute them asynchronously. The method will return a Future
, CompletableFuture
, or ListenableFuture
, which can be used to handle results or exceptions.
Example:
@Service
public class MyService {
@Async
public CompletableFuture<String> processAsync() {
// Simulate a long-running task
Thread.sleep(2000);
return CompletableFuture.completedFuture("Processed");
}
}
You can then call this method asynchronously:
@Autowired
private MyService myService;
public void execute() {
CompletableFuture<String> future = myService.processAsync();
future.thenAccept(result -> System.out.println("Result: " + result));
}
3. Use Cases: Asynchronous processing is useful for tasks such as sending emails, processing data, or making non-blocking HTTP requests, where you don’t want to block the main thread.
Reactive Programming
Reactive Programming is a paradigm focused on handling asynchronous data streams and backpressure, making it ideal for building responsive and scalable applications. Spring WebFlux is a reactive framework that supports reactive programming.
Introduction to Spring WebFlux
Spring WebFlux: Spring WebFlux is a reactive framework built on Project Reactor, providing support for reactive programming in Spring applications. It allows you to build non-blocking, event-driven web applications.Key components:
Flux: Represents a stream of 0 to N elements.
Mono: Represents a stream of 0 to 1 element.
Setting Up WebFlux: Include the spring-boot-starter-webflux
dependency in your project to start using Spring WebFlux.
Example dependency:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-webflux</artifactId>
</dependency>
Implementing Non-Blocking Services with Spring Boot
- 1. Creating Reactive Controllers: Use
@RestController
with reactive types such asMono
andFlux
to create non-blocking endpoints. - Example:
@RestController
public class ReactiveController {
@GetMapping("/flux")
public Flux<String> getFlux() {
return Flux.just("A", "B", "C")
.delayElements(Duration.ofSeconds(1));
}
@GetMapping("/mono")
public Mono<String> getMono() {
return Mono.just("Hello, Reactive World!");
}
}
2. Reactive Data Access: Use Spring Data R2DBC or Spring Data MongoDB for reactive data access, enabling non-blocking database interactions.
Example with R2DBC:
@Repository
public interface MyReactiveRepository extends ReactiveCrudRepository<MyEntity, Long> {
}
3. Handling Backpressure: Reactive streams handle backpressure to ensure that data is processed at a rate the consumer can handle, preventing overload.
Example of backpressure handling:
Flux.range(1, 100)
.onBackpressureBuffer(10) // Buffer up to 10 elements
.subscribe(System.out::println);
By incorporating caching, asynchronous processing, and reactive programming into your Spring and Spring Boot applications, you can significantly improve performance and scalability. Each approach offers unique benefits and can be used individually or in combination to achieve optimal results.
Conclusion
The Future of Spring and Spring Boot
As technology continues to evolve, so does the Spring ecosystem. The future of Spring and Spring Boot is promising, with ongoing developments aimed at making Java development even more efficient, scalable, and developer-friendly. Here are some of the key trends and upcoming features to watch out for:
- Continued Emphasis on Cloud-Native Development: As cloud computing becomes more prevalent, Spring is increasingly focusing on providing tools and features for cloud-native development. Spring Cloud continues to grow, offering even more robust support for microservices, distributed systems, and cloud platforms like Kubernetes.
- Reactive Programming and WebFlux: With the rise of non-blocking, reactive systems, Spring WebFlux and reactive programming models are becoming more important. The future will likely see deeper integration of reactive programming across the Spring ecosystem, making it easier to build high-performance, responsive applications.
- Enhanced Developer Experience: Spring Boot has always prioritized convention over configuration to simplify development. Future updates will likely focus on further streamlining the development process, possibly introducing more intelligent defaults, improved tooling, and greater support for modern development practices like CI/CD and DevOps.
- Native Java Support: Projects like GraalVM and Spring Native are working towards providing native image support, enabling Spring applications to start up faster and consume less memory. This is particularly important for microservices and serverless architectures, where resource efficiency is crucial.
- Security Enhancements: With the growing emphasis on cybersecurity, future releases of Spring Security are expected to introduce more sophisticated authentication, authorization, and encryption mechanisms, along with enhanced support for industry standards like OAuth 2.0 and OpenID Connect.
Final Thoughts
Mastering Spring and Spring Boot is more than just a technical achievement; it’s a gateway to building robust, scalable, and modern applications that can thrive in today’s dynamic IT landscape. As we’ve explored, the Spring ecosystem provides a rich set of tools and frameworks that simplify complex enterprise application development, making it accessible to both seasoned developers and newcomers alike.
The importance of understanding core Spring concepts like IoC, AOP, and MVC, combined with the simplicity and power of Spring Boot, cannot be overstated. By leveraging advanced features such as caching, asynchronous processing, and reactive programming, developers can optimize their applications for performance and scalability.
Looking ahead, staying up-to-date with the latest trends and features in the Spring ecosystem will ensure that you remain at the forefront of Java development. Whether you’re building monolithic applications, microservices, or cloud-native solutions, Spring and Spring Boot will continue to be invaluable tools in your software development arsenal.
Embrace the evolution of Spring, and you’ll be well-equipped to tackle the challenges and opportunities that lie ahead in the ever-changing world of software development.