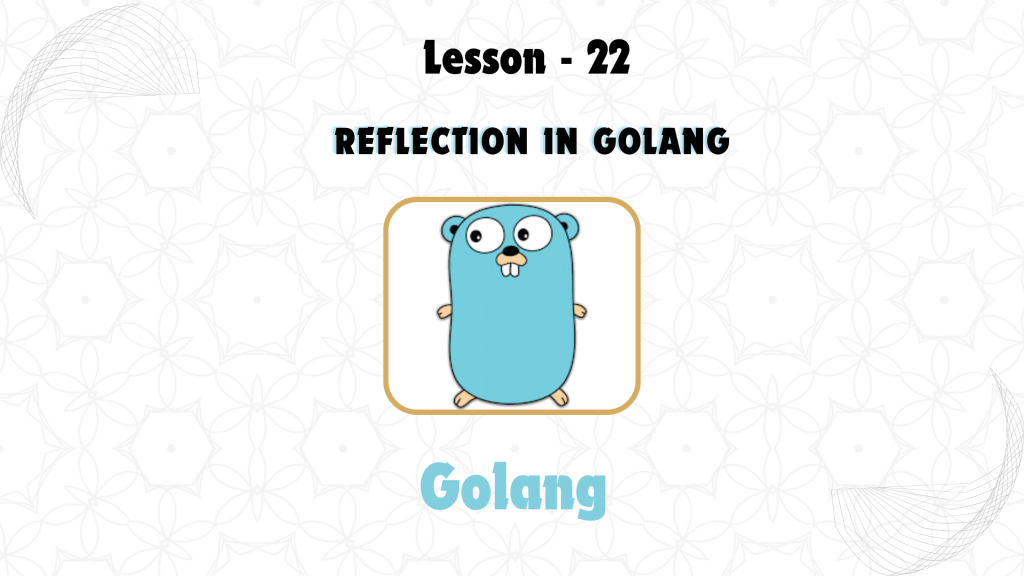
In Lesson 22, we dive into reflection, a powerful feature in GoLang that allows us to inspect and manipulate variables, types, and functions at runtime. Reflection is particularly useful for creating generic libraries, testing frameworks, and handling dynamic data types.
1. Introduction to Reflection
Reflection is facilitated by the reflect
package in GoLang. This package provides utilities to access the types and values of variables at runtime, making it possible to inspect their properties and even modify them.
Basic Concepts
reflect.Type
: Represents the type of a variable.reflect.Value
: Represents the value of a variable.interface{}
: Reflection works with empty interfaces (interface{}
), which can store any value.
Example:
package main
import (
"fmt"
"reflect"
)
func main() {
var x float64 = 3.14
fmt.Println("Type:", reflect.TypeOf(x))
fmt.Println("Value:", reflect.ValueOf(x))
}
Explanation:
reflect.TypeOf(x)
: Returns the type of the variablex
(e.g.,float64
).reflect.ValueOf(x)
: Returns the value of the variablex
.
2. Inspecting Types with Reflection
GoLang’s reflection allows you to dynamically inspect the structure of variables, including fields in structs and function signatures.
Getting the Kind of a Type
You can use the Kind()
method to determine the kind of a type, such as whether it is an integer, string, struct, etc.
Example:
package main
import (
"fmt"
"reflect"
)
func main() {
x := 42
fmt.Println("Type:", reflect.TypeOf(x))
fmt.Println("Kind:", reflect.TypeOf(x).Kind())
}
Explanation:
reflect.TypeOf(x).Kind()
: Returns the kind of the type, which will beint
in this case.
Inspecting Struct Fields
You can use reflection to access and modify fields of a struct.
Example:
package main
import (
"fmt"
"reflect"
)
type Person struct {
Name string
Age int
}
func main() {
p := Person{Name: "John", Age: 30}
value := reflect.ValueOf(p)
for i := 0; i < value.NumField(); i++ {
fmt.Println("Field:", value.Field(i))
}
}
Explanation:
reflect.ValueOf(p)
: Converts the structp
to a reflect value.value.NumField()
: Returns the number of fields in the struct.value.Field(i)
: Accesses each field’s value.
3. Modifying Values with Reflection
Reflection allows you to modify the value of a variable, but only if the variable is passed by reference (a pointer).
Example:
package main
import (
"fmt"
"reflect"
)
func main() {
x := 10
value := reflect.ValueOf(&x).Elem()
value.SetInt(42)
fmt.Println("Updated Value:", x)
}
Explanation:
reflect.ValueOf(&x).Elem()
: Gets the value of the pointerx
and allows modification.value.SetInt(42)
: Sets the new integer value to42
.
4. Reflection with Functions
You can also use reflection to inspect and invoke functions dynamically.
Inspecting Function Signatures
You can retrieve information about the arguments and return types of a function.
Example:
package main
import (
"fmt"
"reflect"
)
func add(a, b int) int {
return a + b
}
func main() {
funcType := reflect.TypeOf(add)
fmt.Println("Number of arguments:", funcType.NumIn())
fmt.Println("Return type:", funcType.Out(0))
}
Explanation:
reflect.TypeOf(add)
: Gets the reflection type of the functionadd
.funcType.NumIn()
: Returns the number of input parameters.funcType.Out(0)
: Returns the type of the return value.
Invoking Functions with Reflection
You can use reflection to call functions dynamically, which is useful in certain testing and dynamic applications.
Example:
package main
import (
"fmt"
"reflect"
)
func multiply(a, b int) int {
return a * b
}
func main() {
funcValue := reflect.ValueOf(multiply)
args := []reflect.Value{reflect.ValueOf(3), reflect.ValueOf(4)}
result := funcValue.Call(args)
fmt.Println("Result:", result[0].Int())
}
Explanation:
reflect.ValueOf(multiply)
: Gets the reflection value of the functionmultiply
.funcValue.Call(args)
: Invokes the function with the provided arguments.
5. Limitations and Performance Considerations
While reflection is a powerful tool, it should be used judiciously due to its runtime cost. Reflective operations are slower than direct operations because GoLang has to perform dynamic type checks and conversions. For most performance-critical applications, it’s better to avoid reflection unless absolutely necessary.
Key Takeaways:
- Reflection allows you to inspect and manipulate types, values, and functions at runtime in GoLang.
- It is particularly useful in dynamic applications, testing, and frameworks, but it should be used cautiously due to its performance impact.
- The
reflect
package provides a wide range of tools for working with types, values, and function signatures.