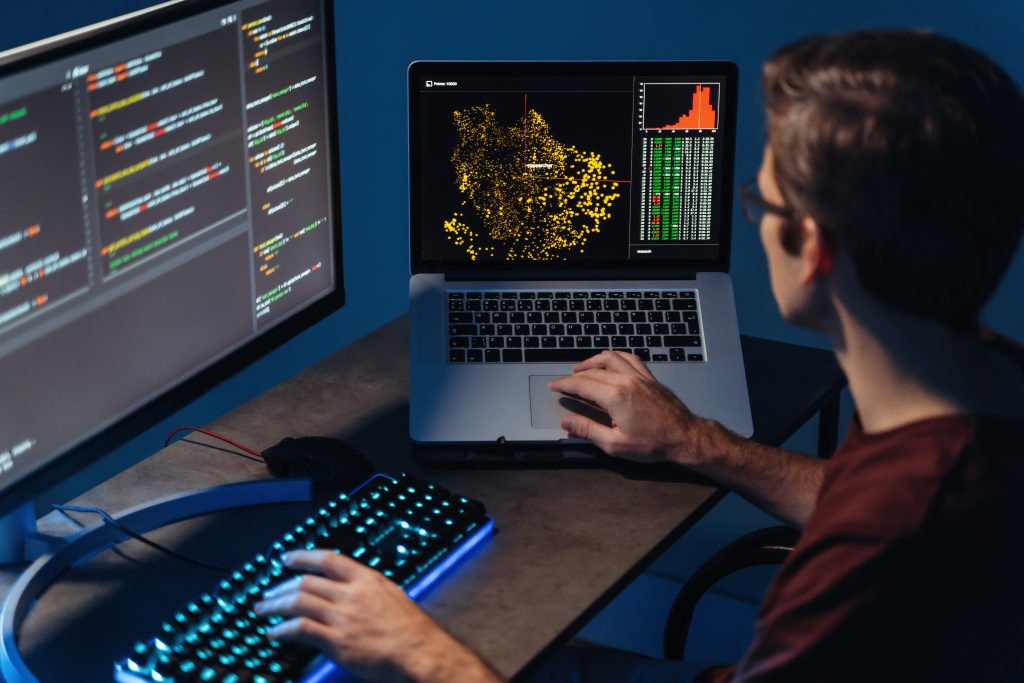
Python, an open source, general purpose, high level programming language and has been around for decades. In the past few years it has become popular language for many in the field of financial and economics, (including R), especially for data analytics and machine learning.
Software and Tools: For the purpose of this article, we are using Anaconda, with compiler and inbuilt libraries for Python and much more. It is free to download (https://www.continuum.io/downloads) and available for most popular operating systems. Anaconda is the leading open data science platform powered by Python. The open source version of Anaconda is a high performance distribution of Python and R and includes over 100 of the most popular Python, R and Scala packages for data science. We expect readers to have Anaconda installed and have basic familiarity with how to start and stop the python program. Reader must have basic programming background, and basic knowledge of Python is good, but not required. Once you have Anaconda installed, you can open up the Jupyter notebook app by clicking the launch button
Programming in Python:
In next few sections, we will explain some of the basic concept of programming paradigm, as related with Python programming language.
Hello World using Jupyter: Jupyter is basically a server-client application that runs on the default web browser (client) of your operating system. Jupyter comes with already installed Python kernel, and other language kernels may also be installed. For the purposes of these articles we are going to use Python 3+ as default interpreter. Using Jupyter, we work on the IPython notebook files (*.ipynb), which are easy to share and run. Since, Anaconda install provides us both Jupyter and Python, so we are good to go!
Create a new python notebook using Jupyter, and make sure your Python version is 3.6 +. The programs in this article are tested using Python 3+, but they should work fine with Python 2.7
You can click on the title (“Untitled”) and rename this notebook to something that you like; we are calling it, “HelloWorld”.
In the section where it says In [1]:, type of the following code in the cell and press shift+enter together or you can press the play button (run cell, 8th icon) in Jupyter.
Viola! You should see the hello message. As you notice, if fairly easy to use Jupyter dashboard. You can open and shut down files or folders, as well rename or duplicate them. You can store them anywhere you like your system or cloud directory.
Explore this dashboard how to group files into folder, create new folder, or create new python notebook and much more. They are mostly easy to understand and if you have experience with any IDE, this should pretty much self-explanatory.
Variables, Conditional Statements, and Data types in Python:
To create new cell, just press shift+enter, and new Jupyter cell will be created. This time enter the following code and run the cell, by pressing shift+enter.
In the above example, what we have is a Python list variable called x, with four values, when you run this cell, it shows output of 1, 2, 3, 4 exactly same as contents of the list x.
Variables in any programming are local storage of a data type. See the cells below:
As you see, mark is a variable, storing a temporary value of 90, an integer, and all that the cell does is prints it’s value. Perfect, but what if we request to print Mark?
We get an error, and that is because Python is case sensitive. Mark is not the same as variable mark. Now lets get into how we can build logical conditional statements.
Notice how we are created if-else statement, all the program doing is checking if the mark variable is more than 90, print A grade, else B grade. Quite simple to use, but notice how semicolon + tabs/white spaces are used in Python to distinguish various conditional statements. Other languages use parenthesis or some other mechanism; Python is unique to use tabs and white spaces. This is why it’s important to fragment and keep your code clean. All right, now what if we want to grade folks based upon various marks they get in a subject? Let’s modify our code:
Interesting, in Python you can use greater than or equal to compute a conditional statement. If you have programmed in any modern programming language it’s not much different. Single = is for assignment of a value to variable, while == is to compute if two values are equal (return true or false). In addition, >= means if greater than or equal to, and <= means if less than equal to. We will deal with more complex conditional statements and exception handling as we go along, but for now this is good. Lets change the marks to 59 and see what we get.
As expected last else statement is executed as none of if or elif (short of else if in Python) statement has come true.
You can assign multiple variable values in the same declaration statement.
As you notice above, variables a and b, wherein they are assigned different values in the same statement deceleration. Notice how both variables of are of different data types, we will cover them in coming section. For now let’s look at type feature of python. In the example below we have declared a variable called test1, and we have assigned it a value of 3, now if we just want to now what to know its data type, just write type(test1) and execute, we got an int.
Below you can notice few more basic data types in Python:
int (integer), floats (real numbers), booleans (bool), string (str), list, tuple, class, and dict (dictionary) are the most common data types in Python.
Here is few interesting Boolean assessment in Python, returning true or false:
String, sets of characters are easy to demonstrate in Python, everything in quotes is basically a string. You can output/print just by typing the variable name or using + sign, easy example:
Arithmetic Operations in Python (Operands and Operators): When you do calculation such as 2 + 3 =5, the numbers 2 and 3 are operands and + sign is the operator. Python has excellent built in support for mathematical functions and that’s’ why its very popular for financial modeling, we will get to that later, lets try following examples.
Now lets look at the interesting example of outcome from the knowledge we have acquired thus far:
We assign a squared value of 4 to variable a, and in the next line we are trying to evaluate if that value is 16? Of course, Python prints false, because squared value of 4 is 256 (4 x 4 x 4 x 4 = 256). Notice how in just two lines you could express so much with great level of clarity. The designers of the language had simplicity in the mind as prime requirement. One of the reason, Python is enjoying the popularity within programming community.
Reassignment of values: Lets’ say if we have a variable, x, and give it a value of 5, and in next statement, reassign a different value, say 6, and try to print the value. It will no longer be 5, it will become 6 – this is true practically for all programming languages, as you have changed the value stored in the variable.
Comparison Operator: Comparison operator in Python evaluates the left and right side of the equation. For example if we type 1 == 2 (with two equal sign we are not assigning but comparing left and right), the answer will be false because 1 is not equal to 2. Another example maybe if we have 3 > 2, this will evaluate to true because 3 is indeed larger than 2. Here are few examples you should try.
Logical and Identity Operators: Logical operators in Python are the words, NOT, AND, OR. They compare certain amount of statement and return a Boolean value, true or false, hence their second name, Boolean operators. AND operator checks if two statements around are true. Here are some examples.
Likewise, OR operator checks if any one of two statements is true.
NOT is an interesting one, because it returns the opposite of the given statement.
The fun starts when three of them are in the same statement, then they have there own order of precedence, not comes first, than and, last is or.
To fully understand the concept, consider following four interesting cases:
Similar to logical operators, in Python we have Identity operator – is and is not. They are actually quite easy to use and here are few examples.
Order of precedence: Lets take an example if you want to add and multiple in the same statement? Well remember order of precedence from you math classes? It’s the same here in Python.
Out of 4 + 3 * 4 is 16, while user may have been expecting 28? Well, the way Python read is what user want is: 4 + (3 * 4) and not (4 + 3) * 4. Order of precedence is higher with /, *, + and -. It is a good idea to use brackets, otherwise may cause confusion, here are few examples.
List and Indexing in Python: Now lets get to another important concept in Python about Indexing. As we discussed earlier, we can declare a variable that may store more than one value. For example, lets say we need a program for shopping list, and that list has fruits as variable with various fruit types. See below example:
Above, fruits declared as a variable that is holding multiple values in squared brackets. But when we try to access the first value, it gives us an apple? It should be banana? Well, indexing in Python starts with 0 and not one, see below:
So now we have our three types of fruits and if we try to give value of 3 or higher, the program will show error (out of bound) because we don’t have anything stored at index 3 or higher. This is important concept in Python and we will use extensively in coming examples. Also the variable fruit in our above example is known as a list or an array of things of same type in Python. You can add and remove things from the list. See below example to add or remove its from a Python list
Using inbuilt Python function of remove and append, we can easily remove items from a list or add new items. Another cool feature is you can take any object, say a string, and use square brackets to get a specific value at index location.
Functions and Indentation: In programming, you should organize your code into few lines with specific task and logic. For example, lets say we want to build our own calculator that does few simple tasks such as addition, multiplication, division, subtraction and power of operations. Instead of writing code in number of lines, we divide them into logical segments, also called as methods or functions. We use Python “def” as definition of a function (block of code), which may or may not take an argument and does something specific. The function may or may not return a value. In our example, we are taking two numbers as arguments, execute a mathematical calculation, and return a computed value. Notice how Python indentation is used for logically organizing the code. We covered a bit of this in our previous example, but here is a detailed example:
The important aspect here is you can actually call your functions anywhere in the program. This encourages reuse; in fact, most of the code written today is not from the scratch but built upon the existing functions. As we discussed above, we can call various operations on our list of fruits, such as remove or add or print. Those operations are nothing but functions written by someone else for us in Python. We just call them and use those functions. This is called code reuse.
Programmers usually organize their code in functions which maybe part of a library. In Python, there are many inbuilt libraries. Each one of them does something unique and are quite useful. Sometimes we have import these set of functions or libraries for a specific task. Let us show you buy playing a fun game.
Built-in Function in Python: The Python interpreter has a number of functions and types built into it that are always available. They are listed here in alphabetical order.
If you want to know all the built-in functions in your specific Python version, try following:
Game in Python: Heads or Tails? Let’s design and play a game wherein we want to randomly toss a coin and see if we get a head or tails? Count how many heads or tails did we get, and see who wins? Well how do we generate a random number? Well here come inbuilt libraries that we may just import a set of functions associated with random numbers and design our game.
We imported a library of random number generator, we declared a variable called toss, and we stored 0 or 1 randomly generated by computer. If the number generated is 0, well call it heads otherwise tails. Now what if we want this program to run 10 times?
For Loop in Python: Let’s modify above program to see if we can run a loop for say ten times to make the game more interesting. One easiest way to create a loop in Python by using for statements. You specify number of times you want loop to run:
Now if you run this program, you should randomly see heads and tails printed. Excellent, you may try to change the size of loop and see your output.
Lets’ count how many heads and tails are generated by the system:
We now have two more variables defined, count and MAX. Where count starts with 0 and every time have head count goes up by one. MAX is the size of the loop we want to run. In the end, we just print number of heads and tails computer generated. So lets’ see another way of running a while loop, say you want to out a message 5 times:
Yet another way to iterate through a list of items using a for loops is:
Tuple: An immutable sequence or list in Python: As we noticed in Python list data types, you may add or remove items from the list. Sometime programmers need a constant (immutable) list, called as Tuple in Python. For example, lets suppose our program needs all the state names in US, now this information will not change over the time. So actually it might be better to store such constant info in a tuple instead of a regular list data structure.
Now lets try to append another state:
The main difference between list and tuples is the mutability, because tuples are immutable, they may server as keys in dictionaries (coming next).
Dictionaries in Python: In common usage, a dictionary is a collection of words matched with their definitions. Given a word, you can look up its definition. Python has a built in dictionary type called dict which you can use to create dictionaries with arbitrary definitions for character strings. It can be used for the common usage, as in a simple English-Spanish dictionary.
To refer to the definition for a word, you use the dictionary name, follow it by the word inside square brackets. This notation can either be used on the left-hand side of an assignment to make (or remake) a definition, or it can be used in an expression (as in the print functions), where its earlier definition is retrieved. There are many more functions possible with dictionaries, here are few examples, and reader must try them and play with more:
Sets in Python: Another sequence type found in Python is a set. A set is an unordered sequence that cannot contain duplicates. Like a tuple it can instantiated with a list. There are few differences. First, unlike lists and tuples, a set does not care about order. Pass the set class a list, and it will create a sequence with any duplicates removed:
User Input with While loop: Thus far we have shown example of different data types and output print statements. Well how about taking user inputs? Lets’ design a new game. We want computer to generate a random number between 0 and 100, and user is supposed to guess what number it is. So long the answer is incorrect, the program keeps telling the user if there input is too high or too low, and the moment the condition is met i.e. secret and guess numbers are the same, the program stops and prints in how many attempts user found the number. Here goes the code:
Notice how input statement work above, and how we are casting the input as int type because program expects an integer input for the game. Well the program works fine till user inputs an integer, what happens if user erroneously inputs a wrong data type? Lets’ try:
Oops! The program bonged because it was expecting an integer while, we fed it a string type. This is where Python exception handling comes handy! We can use try and except block to warn user to only input data of type integer.
Well few lines of code take care of this situation as shown above. Notice how the code block gets indented by an extra tab to accommodate the try/except code segment. Fun fact, you can guess a number between 0 and 100 in no more than 7 tries. Think why so, and what will happen if we change the range between 0 and 1000?
Sample examples with Python: In this last section, let us walk you through few examples and try to cover the basics we have addressed in this article. We will go through code snippets to help you venture more.
Example 1: Lets’ suppose we want to reverse our list of fruits, and few lines of code and change our output.
If you see variable fruits[::-1] will print last value first. You should try more examples with what happens fruits[1::] or be more creative.
Example 2: Lets’ suppose we want to find IP address of a website, given the hostname. We are going to use socket library of Python and simply call library function called gethostbyname.
Conclusion: Well, this is basic article on Python language and we sincerely hope you enjoyed the journey. There is much more to Python and modules than this. Our intent is to familiarize you with the syntax and to get a start with Python and Jupyter. Stay tuned, more to come on Python from team IrisLogic.