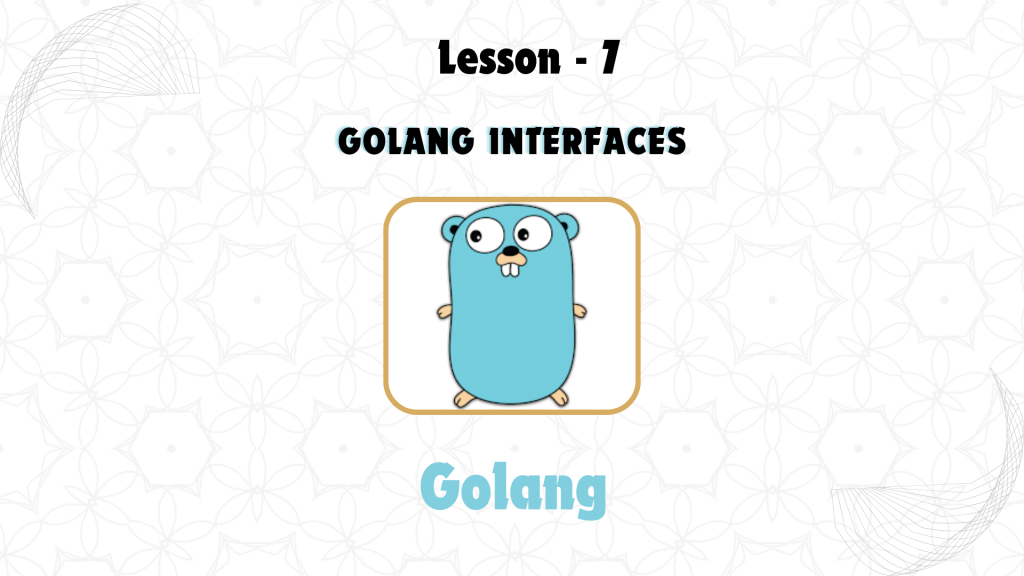
1. What are Interfaces in GoLang? Interfaces in GoLang define a set of method signatures (behaviors) that types must implement. An interface allows different types to be treated as one. Unlike other languages where you explicitly declare that a type implements an interface, in Go, if a type implements all the methods of an interface, it is implicitly considered as implementing that interface.
Here’s a basic example of an interface:
type Speaker interface {
Speak() string
}
Any type that implements the Speak
method can be considered a Speaker
.
2. Implementing Interfaces A type implements an interface simply by having methods that match the interface’s method signatures. There’s no need to explicitly declare that a type implements an interface.
For example:
type Dog struct{}
func (d Dog) Speak() string {
return "Woof!"
}
type Cat struct{}
func (c Cat) Speak() string {
return "Meow!"
}
Both Dog
and Cat
implement the Speak
method, so they implicitly satisfy the Speaker
interface.
You can now write a function that accepts the Speaker
interface as a parameter:
func MakeItSpeak(s Speaker) {
fmt.Println(s.Speak())
}
You can call this function with any type that implements the Speaker
interface:
dog := Dog{}
cat := Cat{}
MakeItSpeak(dog) // Output: Woof!
MakeItSpeak(cat) // Output: Meow!
3. Interface Practical Use Cases Interfaces in GoLang are widely used to make programs flexible and adaptable to different types without changing the code. This is especially useful for writing generic functions, allowing you to write code that can work with different types.
Here are a few practical use cases of interfaces:
- Polymorphism: Interfaces allow different types to be treated uniformly, so you can create functions that accept interface types and work with any data type that implements the required methods.
- Dependency Injection: Interfaces are often used to inject dependencies, making testing and decoupling of components easier.
4. Empty Interfaces An empty interface is declared using interface{}
and can hold values of any type. This is because every type implements at least zero methods, making them compatible with the empty interface.
Example:
func Describe(i interface{}) {
fmt.Printf("(%v, %T)\n", i, i)
}
Describe(42) // Output: (42, int)
Describe("hello") // Output: (hello, string)
While the empty interface is powerful and flexible, it’s generally better to use more specific interfaces to ensure type safety and readability.
5. Type Assertions and Type Switches When you work with an interface, you may need to retrieve the actual value or type. This is done using type assertions or type switches.
Here’s how to perform a type assertion:
var i interface{} = "hello"
s := i.(string) // Type assertion
fmt.Println(s) // Output: hello
To handle cases where the assertion may fail:
s, ok := i.(string)
if ok {
fmt.Println(s) // Output: hello
} else {
fmt.Println("Not a string")
}
Type switches allow you to handle multiple types in a single statement:
func CheckType(i interface{}) {
switch v := i.(type) {
case int:
fmt.Printf("Integer: %d\n", v)
case string:
fmt.Printf("String: %s\n", v)
default:
fmt.Printf("Unknown type\n")
}
}
Lesson Summary:
- Interfaces define a set of methods that types can implement, enabling polymorphism and flexibility in GoLang.
- You can implement interfaces implicitly by defining methods that match the interface.
- Empty interfaces can hold values of any type but should be used cautiously to avoid losing type safety.
- Use type assertions and type switches to extract concrete types from interfaces.
This concludes Lesson 7: GoLang Interfaces. Understanding and utilizing interfaces in GoLang is key to writing reusable, flexible, and decoupled code.