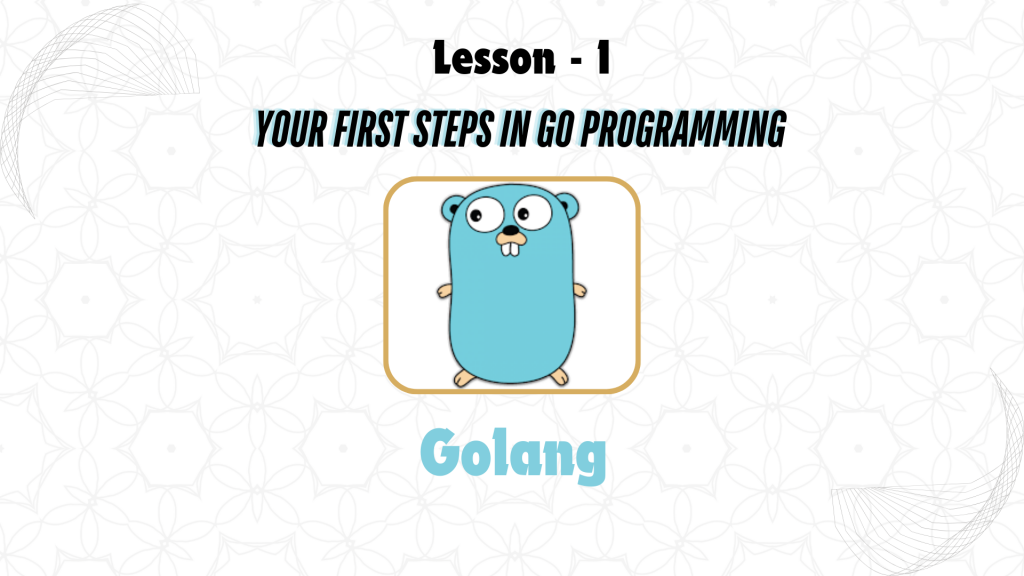
What is GoLang?
GoLang, commonly known as Go, is an open-source programming language designed by Google. It was created by Robert Griesemer, Rob Pike, and Ken Thompson and officially announced in 2009. Go is known for its simplicity, efficiency, and ease of use, making it an attractive choice for a wide range of applications from web servers and networking tools to data processing and cloud services.
Why Learn GoLang?
GoLang offers several compelling reasons for developers to consider it:
- Simplicity and Ease of Use: GoLang’s syntax is clean and minimalistic, which simplifies the learning curve for new developers. Its design philosophy emphasizes simplicity and clarity, avoiding complex features that could lead to confusion.
- Performance: GoLang is a statically typed, compiled language, meaning that Go code is compiled directly into machine code, resulting in high performance and efficiency. This makes Go suitable for performance-critical applications.
- Strong Standard Library: Go comes with a rich standard library that includes packages for handling I/O, string manipulation, networking, and more, allowing developers to build robust applications with less reliance on third-party libraries.
Key Features
- Goroutines and Concurrency: One of Go’s standout features is its built-in support for concurrent programming. Goroutines are lightweight threads managed by the Go runtime, allowing developers to perform multiple tasks simultaneously without the overhead of traditional threading models. This makes Go particularly effective for building scalable and responsive applications.
- Garbage Collection: Go includes an efficient garbage collector that automatically manages memory allocation and deallocation, reducing the complexity and potential errors associated with manual memory management.
- Simplicity and Readability: Go’s syntax is designed to be simple and readable, which improves code maintainability. Features like consistent formatting and straightforward error handling contribute to clean and easy-to-understand code.
- Static Typing and Efficiency: Go is a statically typed language, which means that types are checked at compile-time. This helps catch errors early in the development process and contributes to more efficient and predictable code execution.
- Built-in Tools: Go provides a suite of built-in tools for tasks such as formatting code (
go fmt
), managing dependencies (go mod
), and running tests (go test
). These tools are integrated into the Go toolchain, enhancing developer productivity.
Benefits of Using Go (Efficiency, Concurrency, Scalability)
- Efficiency: GoLang’s design ensures high performance and efficient execution of programs. Its compilation process produces optimized machine code, which translates to faster runtime performance. The language’s minimalistic syntax also reduces the complexity of code, further enhancing efficiency.
- Concurrency: Go’s concurrency model, based on goroutines and channels, allows developers to write highly concurrent programs with ease. This makes it ideal for applications that require handling multiple tasks simultaneously, such as web servers and real-time systems.
- Scalability: Go’s efficient handling of concurrency and memory management supports the development of scalable systems. The language’s design allows it to handle large-scale applications and high loads gracefully, making it a preferred choice for modern cloud-based and distributed systems.
2. Setting Up the GoLang Environment
Setting up the GoLang environment involves installing the Go programming language, configuring your workspace, and verifying the installation. This guide will walk you through the process for different platforms and ensure you’re ready to start coding in Go.
Installing GoLang on Different Platforms
Windows:
- Download the Go Installer:
- Visit the official GoLang website at golang.org/dl.
- Download the Windows installer (
.msi
file) for the latest version of GoLang.
- Run the Installer:
- Double-click the downloaded
.msi
file to start the installation process. - Follow the on-screen instructions, and accept the default settings.
- Double-click the downloaded
- Add Go to System PATH:
- The installer should automatically configure the PATH environment variable. To verify, open Command Prompt and type
go version
.
- The installer should automatically configure the PATH environment variable. To verify, open Command Prompt and type
macOS:
- Download the Go Package:
- Go to golang.org/dl and download the macOS package (
.pkg
file).
- Go to golang.org/dl and download the macOS package (
- Run the Installer:
- Double-click the
.pkg
file and follow the installation instructions.
- Double-click the
- Verify the Installation:
- Open Terminal and type
go version
to check if Go is installed correctly.
- Open Terminal and type
Linux:
- 1. Download the Go Binary:
- Visit golang.org/dl and download the appropriate tarball (
.tar.gz
file) for your system architecture (e.g.,go1.XX.linux-amd64.tar.gz
).
- Visit golang.org/dl and download the appropriate tarball (
- 2. Extract the Tarball:
- Open Terminal and navigate to the directory containing the downloaded tarball.
- Run the command:
tar -C /usr/local -xzf go1.XX.linux-amd64.tar.gz
3.Add Go to PATH:
- Open your shell profile (e.g.,
~/.bashrc
,~/.zshrc
) and add the following line:
export PATH=$PATH:/usr/local/go/bin
Apply the changes with:
source ~/.bashrc
-
- Verify the installation by typing
go version
in the terminal.
- Verify the installation by typing
Setting Up the Go Workspace
- Create a Go Workspace Directory:
- By default, Go projects are managed within a workspace directory. Create a directory to hold your Go projects, such as
~/go_projects
.
- By default, Go projects are managed within a workspace directory. Create a directory to hold your Go projects, such as
- Organize Your Workspace:
- Inside your workspace directory, you’ll typically create the following structure:
~/go_projects/
└── src/
└── bin/
└── pkg/
- The
src
directory holds your Go source code. Thebin
directory will contain compiled binaries, andpkg
will store package objects.
Set Up the GOPATH:
- The
GOPATH
environment variable specifies the root of your workspace. By default, Go uses~/go
as theGOPATH
, but you can set it to any directory. - To set
GOPATH
, add the following line to your shell profile:
export GOPATH=$HOME/go_projects
Apply the changes with:
source ~/.bashrc
Configuring the GOPATH
- Verify GOPATH Configuration:
- To check if
GOPATH
is set correctly, run
- To check if
go env GOPATH
- It should display the path to your Go workspace directory.
Update Go Modules (Optional):
- For Go versions 1.11 and later, Go modules are used for dependency management, which allows projects to be outside the
GOPATH
directory. You can enable modules by setting the environment variable:
export GO111MODULE=on
-
- Modules simplify dependency management and are recommended for new projects.
Verifying the Installation
- Check Go Version:
- Open a terminal or command prompt and run:
go version
- This command should display the installed Go version, confirming that GoLang is properly installed.
Run a Sample Program:
- Create a simple Go file to test your setup. In your
src
directory, create a file namedhello.go
with the following content:
package main
import "fmt"
func main() {
fmt.Println("Hello, GoLang!")
}
Navigate to the directory containing hello.go
and run:
go run hello.go
-
- If everything is set up correctly, you should see “Hello, GoLang!” printed to the terminal.
By following these steps, you’ll have a fully functional GoLang development environment, ready for building and running Go applications.
3. Writing Your First Go Program
Creating and running your first Go program is a great way to familiarize yourself with the language’s syntax and development workflow. In this section, we’ll cover the basic structure of a Go program, explain the main
function, and demonstrate how to print “Hello, World!” to the console. We will also show you how to run your program using go run
and go build
.
Basic Structure of a GoLang Program
A GoLang program consists of several key components:
- Package Declaration: Every Go program starts with a
package
declaration. Themain
package is special because it tells the Go compiler that this package should be compiled into an executable program. - Import Statement: This section allows you to include external packages into your program. For basic functionality, you will commonly import the
fmt
package, which provides formatted I/O functions. - Main Function: The
main
function is the entry point of the program. It is where the execution starts.
Here’s a basic template for a Go program:
package main
import "fmt"
func main() {
// Code goes here
}
Explanation of main
Function
The main
function is a special function in Go. It is the entry point for the program execution. When you run a Go program, the Go runtime looks for the main
package and then executes the main
function. Here’s a breakdown:
func main()
declares themain
function. In Go,func
is used to define a function.- The code inside the curly braces
{}
is the body of themain
function and contains the logic to be executed.
Printing “Hello, World!”
To write a program that prints “Hello, World!” to the console, follow these steps:
- Create a File: In your workspace directory, create a file named
hello.go
. - Write the Code: Open
hello.go
and add the following code:
package main
import "fmt"
func main() {
fmt.Println("Hello, World!")
}
-
package main
declares that this file is part of themain
package.import "fmt"
imports thefmt
package, which provides functions for formatted I/O.func main()
defines themain
function.fmt.Println("Hello, World!")
prints the string “Hello, World!” to the console.
Running the Program
You can run your Go program in two ways: using go run
or go build
.
1. Using go run
:
- The
go run
command compiles and runs the Go program in a single step. This is useful for quick testing and development.
go run hello.go
You should see the output:
Hello, World!
2. Using go build
:
- The
go build
command compiles the Go source code into an executable binary. This is useful for creating a standalone executable file.
go build hello.go
This command creates an executable file named hello
(or hello.exe
on Windows) in the current directory.
To run the compiled program, simply execute the binary:
./hello
You should see the output:
Hello, World!
By following these steps, you’ve successfully created, executed, and built your first GoLang program. This simple exercise introduces you to the core components of GoLang and sets the stage for more complex programming tasks.
Conclusion
Congratulations! You’ve successfully written and executed your first GoLang program. In this lesson, you learned the fundamental structure of a GoLang program, including the package declaration, the import statement, and the main
function. You also saw how to use the fmt
package to print “Hello, World!” to the console and explored how to run your program using both go run
and go build
.
With these basics under your belt, you’re now ready to dive deeper into GoLang programming. In the next lesson, we’ll explore more advanced topics such as variables, constants, and operators. These concepts are essential for building more complex and functional Go applications.
Stay tuned for Lesson 2, where we’ll continue to expand your GoLang knowledge and help you build a solid foundation for your programming journey.