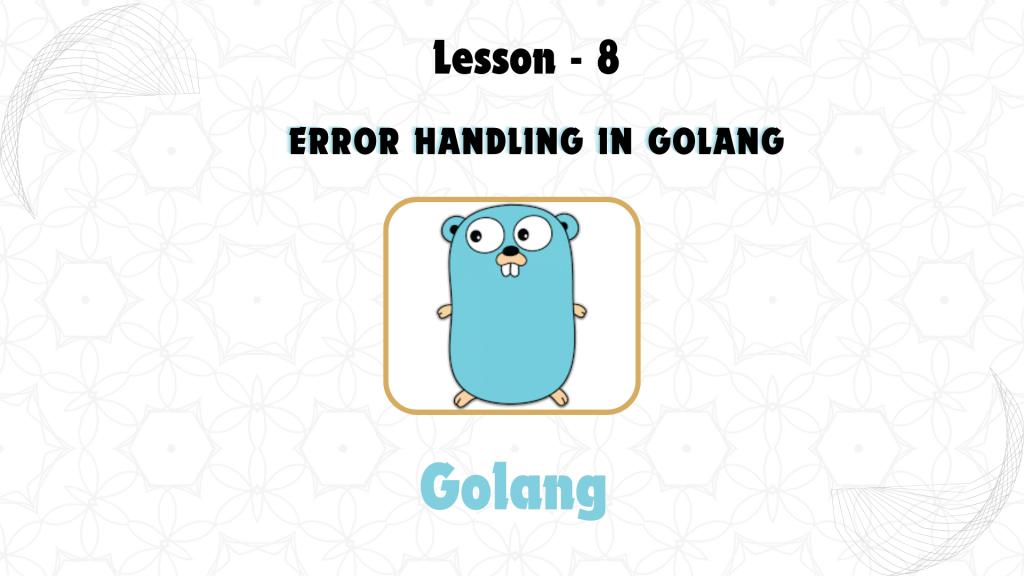
Error handling is a fundamental aspect of any programming language, and GoLang has a unique and efficient way of dealing with errors. Instead of using exceptions like in many other languages, GoLang emphasizes handling errors explicitly, making the code more predictable and easy to follow.
1. Error Values in Go
In Go, errors are handled using the error
type, which is a built-in interface. When a function encounters an issue, it returns an error as the last return value.
Example:
package main
import (
"errors"
"fmt"
)
func divide(a, b float64) (float64, error) {
if b == 0 {
return 0, errors.New("cannot divide by zero")
}
return a / b, nil
}
func main() {
result, err := divide(10, 0)
if err != nil {
fmt.Println("Error:", err)
} else {
fmt.Println("Result:", result)
}
}
In this example, the divide
function returns both a result and an error. If an error occurs (like dividing by zero), the function returns an error object which is checked in the main function.
2. Using defer
, panic
, and recover
GoLang offers additional tools for handling more complex error cases, such as using panic
for unexpected scenarios and recover
for regaining control after a panic.
defer
: Delays the execution of a function until the surrounding function returns.panic
: Stops the normal execution of the program and begins panicking.recover
: Regains control of a panicking goroutine.
Example with defer
, panic
, and recover
:
package main
import "fmt"
func main() {
defer func() {
if r := recover(); r != nil {
fmt.Println("Recovered from panic:", r)
}
}()
panic("A severe error occurred!")
fmt.Println("This will not be printed")
}
In this example, the program panics with an error message, but the recover
function allows it to regain control and handle the error gracefully.
3. Creating Custom Errors
Go allows developers to create custom error types to provide more context about the error.
Example:
package main
import (
"fmt"
)
type MyError struct {
When string
What string
}
func (e *MyError) Error() string {
return fmt.Sprintf("Error occurred at %s: %s", e.When, e.What)
}
func riskyOperation() error {
return &MyError{
When: "Operation 1",
What: "something went wrong",
}
}
func main() {
err := riskyOperation()
if err != nil {
fmt.Println(err)
}
}
In this example, we define a custom error type MyError
which includes additional context (when and what happened).
Key Takeaways:
- Error handling in Go is explicit and involves returning error values.
- Use
defer
,panic
, andrecover
for more complex error handling scenarios. - You can create custom error types to improve error messages and debugging.
Best Practices:
- Always handle errors in GoLang. Ignoring errors can lead to bugs and unexpected behavior.
- Prefer returning error values rather than panicking for routine errors.
- Use
panic
andrecover
only in exceptional situations or when handling unforeseen errors.
Error handling is crucial in making your GoLang applications robust and reliable. Handling errors properly ensures that your code behaves predictably and fails gracefully!