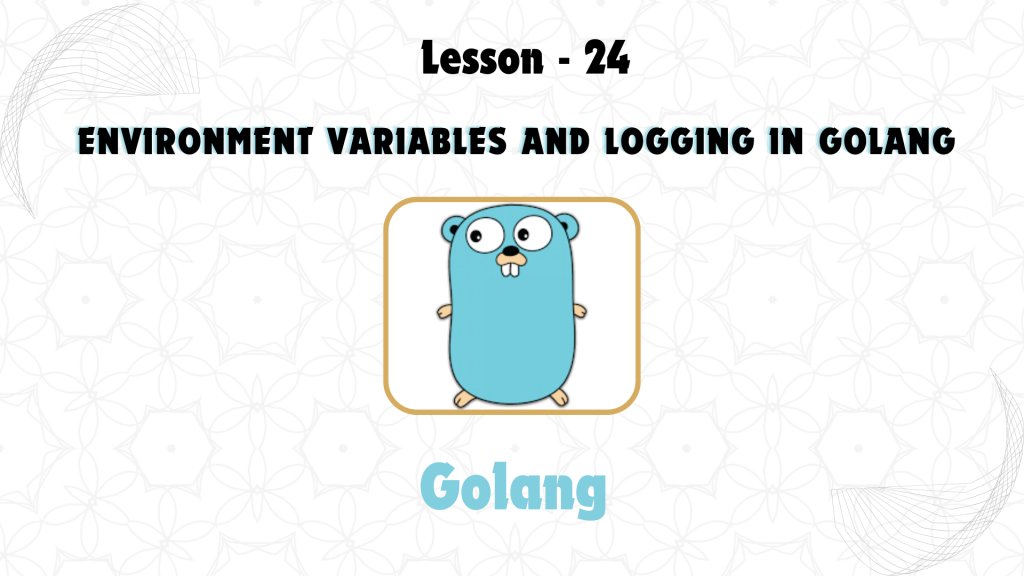
GoLang provides a simple and efficient way to work with environment variables and logging, which are critical components for configuring applications and keeping track of program behavior. This lesson covers how to manage environment variables and how to implement logging in GoLang applications.
1. Working with Environment Variables
Environment variables are key-value pairs that provide information about the environment in which a program is running. They are often used to configure settings without hardcoding values into the application.
Accessing Environment Variables
GoLang provides the os
package, which allows you to retrieve, set, and manage environment variables.
Retrieving Environment Variables:
package main
import (
"fmt"
"os"
)
func main() {
// Retrieving the value of the environment variable
dbHost := os.Getenv("DB_HOST")
if dbHost == "" {
fmt.Println("DB_HOST is not set")
} else {
fmt.Println("DB_HOST:", dbHost)
}
}
Explanation:
os.Getenv("DB_HOST")
: Retrieves the value of theDB_HOST
environment variable. If the variable is not set, it returns an empty string.
Setting Environment Variables:
You can set environment variables in your terminal before running the Go program.
export DB_HOST=localhost
go run envvar.go
Setting and Unsetting Environment Variables in GoLang:
package main
import (
"fmt"
"os"
)
func main() {
os.Setenv("MY_VAR", "myValue") // Setting an environment variable
myVar := os.Getenv("MY_VAR")
fmt.Println("MY_VAR:", myVar)
os.Unsetenv("MY_VAR") // Unsetting the environment variable
fmt.Println("MY_VAR after unset:", os.Getenv("MY_VAR"))
}
Explanation:
os.Setenv()
: Sets an environment variable.os.Unsetenv()
: Unsets an environment variable.
2. Implementing Logging in GoLang
Logging is a fundamental aspect of software development for tracking and diagnosing issues during runtime. GoLang provides a built-in log
package for logging messages with different levels of severity.
Basic Logging:
package main
import (
"log"
)
func main() {
log.Println("This is a basic log message.")
}
Explanation:
log.Println()
: Logs a message with a timestamp.
Logging with Different Severity Levels:
You can use the following methods to log messages with different levels of importance.
- Info:
log.Println("INFO: Informational message.")
Fatal:
log.Fatal("FATAL: This will log the message and then terminate the program.")
Panic:
log.Panic("PANIC: This will log the message and then panic.")
Explanation:
log.Fatal()
: Logs a message and callsos.Exit(1)
to terminate the program.log.Panic()
: Logs a message and causes a runtime panic.
Customizing Log Output:
You can customize the logging output using log.SetFlags()
and log.SetPrefix()
.
package main
import (
"log"
"os"
)
func main() {
log.SetPrefix("INFO: ")
log.SetFlags(log.Ldate | log.Ltime | log.Lshortfile)
file, err := os.OpenFile("app.log", os.O_CREATE|os.O_WRONLY|os.O_APPEND, 0666)
if err != nil {
log.Fatal(err)
}
log.SetOutput(file)
log.Println("This is a log message written to the file.")
}
Explanation:
log.SetPrefix("INFO: ")
: Adds a prefix to every log message.log.SetFlags()
: Adds additional information (e.g., date, time, and file) to the log messages.log.SetOutput()
: Redirects the log output to a file.
Logging to a File:
In this example, logs are written to a file named app.log
. The file is opened with the os.OpenFile()
function, and the log.SetOutput()
method is used to direct the log output to the file.
Creating Custom Loggers:
For more complex applications, you can create custom loggers.
package main
import (
"log"
"os"
)
func main() {
logger := log.New(os.Stdout, "CUSTOM: ", log.LstdFlags)
logger.Println("This is a custom logger message.")
}
Explanation:
log.New()
: Creates a custom logger that writes to the specifiedio.Writer
(in this case,os.Stdout
).
Key Takeaways:
- Environment Variables: Use environment variables to store configuration values without hardcoding them into your application. You can access, set, and unset environment variables using the
os
package. - Logging: GoLang’s
log
package provides simple and powerful tools for logging messages. You can customize log output, write logs to files, and even create custom loggers.