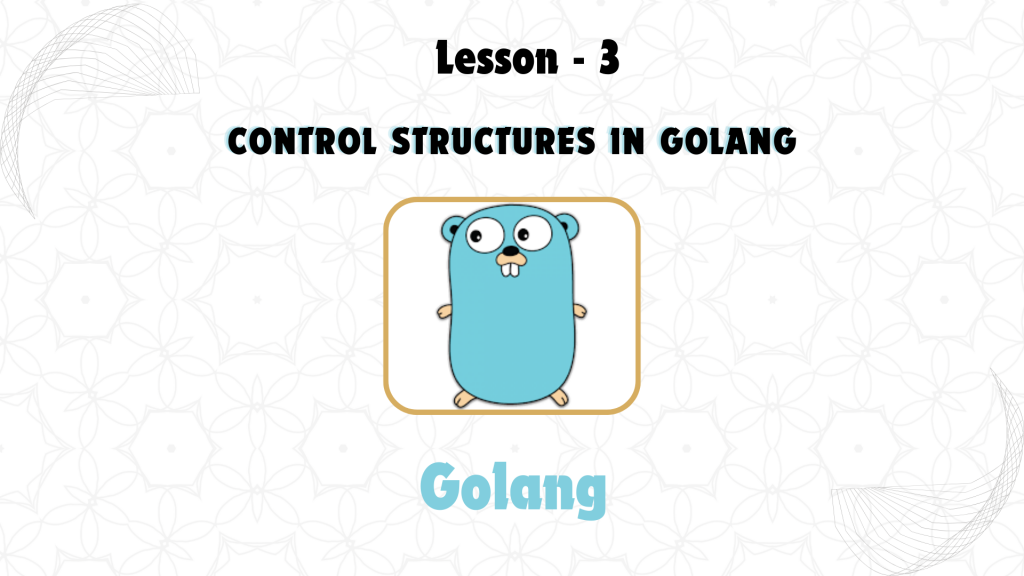
Control structures allow you to direct the flow of your program’s execution. GoLang provides a variety of control structures, including conditional statements, loops, and switch cases, which are crucial for writing logical, dynamic, and efficient code.
Syntax:
if condition {
// code to be executed if condition is true
} else {
// code to be executed if condition is false
}
Example:
package main
import "fmt"
func main() {
age := 18
if age >= 18 {
fmt.Println("You're eligible to vote!")
} else {
fmt.Println("You're not eligible to vote yet.")
}
}
Key Points:
- The condition inside
if
must evaluate to a boolean (true
orfalse
). - You can also use
else if
to check multiple conditions.
2. Loops in GoLang
GoLang has one main loop: the for
loop. This powerful loop can be adapted to act like while
, do-while
, or infinite loops.
Basic for
Loop Syntax:
for initialization; condition; post {
// code to execute
}
Example:
package main
import "fmt"
func main() {
for i := 0; i < 5; i++ {
fmt.Println("Iteration", i)
}
}
Range Loops:
range
is used to iterate over elements in a collection (like arrays, slices, maps, etc.).
Example:
package main
import "fmt"
func main() {
nums := []int{2, 4, 6, 8}
for index, num := range nums {
fmt.Printf("Index %d: %d\n", index, num)
}
}
3. Switch Case in GoLang
switch
in GoLang is a powerful and concise way to handle multiple conditions. Unlike other languages, Go’s switch
doesn’t need to use break
as it automatically exits after a match.
Syntax:
switch expression {
case value1:
// code for case 1
case value2:
// code for case 2
default:
// code if no match
}
Example:
package main
import "fmt"
func main() {
day := "Monday"
switch day {
case "Monday":
fmt.Println("Start of the work week!")
case "Friday":
fmt.Println("Almost the weekend!")
default:
fmt.Println("A regular day.")
}
}
Key Points:
switch
can be used with expressions, variables, and constants.- No need to use
break
explicitly.
4. Break and Continue in Loops
Go provides the break
and continue
statements to control loop execution.
Break:
Exits the loop immediately.
Example:
package main
import "fmt"
func main() {
for i := 0; i < 10; i++ {
if i == 5 {
break
}
fmt.Println(i)
}
}
Continue:
Skips the remaining code in the current iteration and moves to the next iteration.
Example:
package main
import "fmt"
func main() {
for i := 0; i < 10; i++ {
if i%2 == 0 {
continue // Skip even numbers
}
fmt.Println(i)
}
}
Conclusion:
In this lesson, we explored some of the most important control structures in GoLang, such as if-else
, for
loops, switch
cases, and control flow using break
and continue
. Mastering these will enable you to write more dynamic and flexible Go programs.
In the next lesson, we will delve into GoLang Functions, where you’ll learn how to create and use functions, pass parameters, return values, and more. Stay tuned!