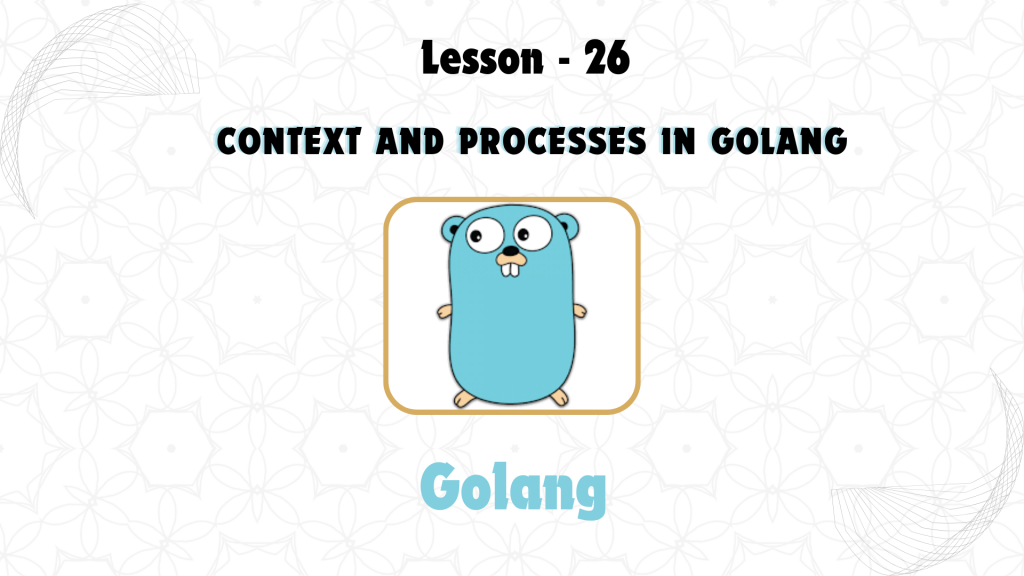
In this lesson, we’ll cover context and process management in GoLang. Context is used to manage deadlines, cancelation signals, and other request-scoped values across API boundaries and between processes. Process management, on the other hand, deals with creating, controlling, and terminating operating system processes.
1. Understanding context
in Go
The context
package in GoLang provides a way to carry deadlines, cancelation signals, and other request-scoped values through a program. It’s primarily used for controlling the lifecycle of requests and services in concurrent programming.
Key Functions in the context
Package:
context.Background()
: Returns an empty context, typically used at the top level of a function call.context.WithCancel()
: Returns a copy of the parent context with cancelation functionality.context.WithDeadline()
: Returns a context with a specific deadline.context.WithTimeout()
: Returns a context that automatically cancels after a specified duration.
Example of Using context.WithCancel
:
package main
import (
"context"
"fmt"
"time"
)
func main() {
ctx, cancel := context.WithCancel(context.Background())
go func() {
time.Sleep(2 * time.Second)
cancel() // Cancel the context after 2 seconds
}()
<-ctx.Done() // Wait for the context to be canceled
fmt.Println("Context canceled:", ctx.Err())
}
Explanation:
- A background context is created with
context.Background()
. - The
cancel()
function cancels the context after a 2-second delay. ctx.Done()
waits until the context is canceled, then prints the cancellation message.
2. Context with Timeout
Timeouts are important in programs that need to ensure operations are completed within a specific time frame. GoLang’s context.WithTimeout
allows you to set an automatic timeout for your context.
Example of context.WithTimeout
:
package main
import (
"context"
"fmt"
"time"
)
func main() {
ctx, cancel := context.WithTimeout(context.Background(), 3*time.Second)
defer cancel()
select {
case <-time.After(5 * time.Second):
fmt.Println("Task completed")
case <-ctx.Done():
fmt.Println("Context timeout:", ctx.Err())
}
}
Explanation:
- The context will be canceled automatically after 3 seconds.
- If the task takes more than 3 seconds, the context will time out, and a timeout message will be printed.
3. Passing Values with Context
Contexts can also store and pass values between functions. This is useful in situations like passing request-specific metadata (e.g., user authentication tokens) throughout a system.
Example of Passing Values:
package main
import (
"context"
"fmt"
)
func main() {
ctx := context.WithValue(context.Background(), "userID", 12345)
processRequest(ctx)
}
func processRequest(ctx context.Context) {
userID := ctx.Value("userID").(int)
fmt.Println("Processing request for user ID:", userID)
}
Explanation:
- A value (
userID
) is stored in the context usingcontext.WithValue
. - The
processRequest
function retrieves the value from the context usingctx.Value
.
4. Managing OS Processes in Go
GoLang provides the os/exec
package for executing external processes. You can start, control, and manage system-level processes (like running a shell command or invoking an external program).
Running a Command with exec.Command
:
package main
import (
"fmt"
"os/exec"
)
func main() {
out, err := exec.Command("echo", "Hello, World!").Output()
if err != nil {
fmt.Println("Error:", err)
return
}
fmt.Printf("Output: %s\n", out)
}
Explanation:
exec.Command
runs the external commandecho Hello, World!
.- The output is captured and printed.
5. Starting and Managing Processes
You can also manage processes with the exec.Command
function, including starting a process, waiting for it to finish, and checking its exit status.
Starting a Process and Waiting for it to Finish:
package main
import (
"fmt"
"os/exec"
)
func main() {
cmd := exec.Command("sleep", "3")
err := cmd.Start()
if err != nil {
fmt.Println("Error starting command:", err)
return
}
fmt.Println("Waiting for command to finish...")
err = cmd.Wait() // Wait for the process to finish
if err != nil {
fmt.Println("Error:", err)
return
}
fmt.Println("Command finished successfully")
}
Explanation:
cmd.Start()
starts thesleep
process.cmd.Wait()
waits for the process to complete before proceeding.
6. Context with Processes
You can also combine context with external processes to handle timeouts and cancellations.
Example of Context with External Processes:
package main
import (
"context"
"fmt"
"os/exec"
"time"
)
func main() {
ctx, cancel := context.WithTimeout(context.Background(), 2*time.Second)
defer cancel()
cmd := exec.CommandContext(ctx, "sleep", "5")
err := cmd.Start()
if err != nil {
fmt.Println("Error starting command:", err)
return
}
err = cmd.Wait()
if ctx.Err() == context.DeadlineExceeded {
fmt.Println("Process timed out")
} else if err != nil {
fmt.Println("Error:", err)
} else {
fmt.Println("Process finished successfully")
}
}
Explanation:
- The
exec.CommandContext
function starts thesleep
command with a context. - If the context’s timeout is exceeded, the process is terminated, and a timeout message is printed.
Key Takeaways:
- Context Management: GoLang’s
context
package is essential for managing deadlines, cancellation, and passing request-scoped values across goroutines and API boundaries. - Process Management: You can use GoLang’s
os/exec
package to run, manage, and control system processes. - Context and Processes: Combining
context
with process management allows you to effectively control process timeouts and cancellation, improving the robustness of your applications.
This lesson provides you with the knowledge to effectively use context
for concurrency control and process management in GoLang.