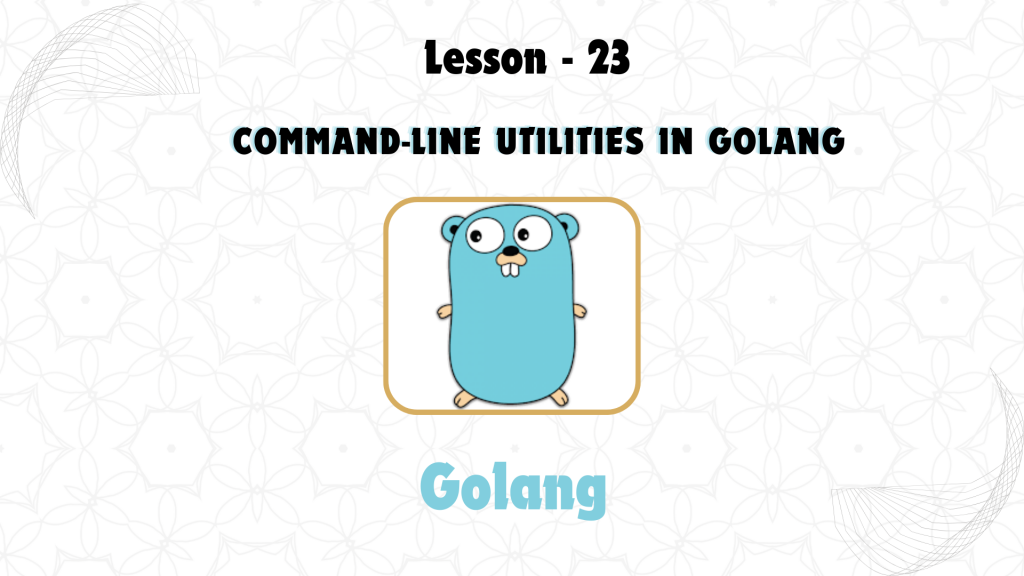
Command-line utilities are one of the most common ways of interacting with programs, and GoLang provides robust support for building command-line tools. This lesson will guide you through the process of creating and handling command-line utilities in GoLang, focusing on argument parsing, flags, and subcommands.
1. Command-Line Arguments in GoLang
GoLang allows you to access command-line arguments via the os
package. The arguments are provided as a slice of strings (os.Args
), where the first element is always the name of the program, and the subsequent elements are the actual arguments passed by the user.
Basic Example:
package main
import (
"fmt"
"os"
)
func main() {
args := os.Args
fmt.Println("Program Name:", args[0])
fmt.Println("Arguments:", args[1:])
}
Explanation:
os.Args
: A slice containing the command-line arguments.args[0]
holds the program’s name, andargs[1:]
holds the rest of the arguments.
Running the Program:
If the program is named cmdutil
, you can run it like this:
go run cmdutil.go arg1 arg2 arg3
The output would be:
Program Name: cmdutil
Arguments: [arg1 arg2 arg3]
2. Using Command-Line Flags
GoLang provides the flag
package for parsing command-line options (also called flags). Flags allow you to specify options to customize the behavior of your program.
Defining Flags:
package main
import (
"flag"
"fmt"
)
func main() {
name := flag.String("name", "GoLang", "Your name")
age := flag.Int("age", 0, "Your age")
flag.Parse()
fmt.Printf("Hello, %s! You are %d years old.\n", *name, *age)
}
Explanation:
flag.String("name", "GoLang", "Your name")
: Defines a string flag with the namename
, a default value ofGoLang
, and a description.flag.Int("age", 0, "Your age")
: Defines an integer flag with the nameage
.flag.Parse()
: Parses the command-line flags and assigns them to the corresponding variables.
Running the Program with Flags:
go run cmdutil.go -name="John" -age=30
Output:
Hello, John! You are 30 years old.
3. Command-Line Subcommands
Subcommands are useful for building more complex command-line utilities, where you have different commands performing different tasks. GoLang can handle subcommands using the flag
package in conjunction with custom logic.
Defining Subcommands:
package main
import (
"flag"
"fmt"
"os"
)
func main() {
addCmd := flag.NewFlagSet("add", flag.ExitOnError)
subtractCmd := flag.NewFlagSet("subtract", flag.ExitOnError)
addA := addCmd.Int("a", 0, "First number")
addB := addCmd.Int("b", 0, "Second number")
subtractA := subtractCmd.Int("a", 0, "First number")
subtractB := subtractCmd.Int("b", 0, "Second number")
if len(os.Args) < 2 {
fmt.Println("Expected 'add' or 'subtract' subcommands.")
os.Exit(1)
}
switch os.Args[1] {
case "add":
addCmd.Parse(os.Args[2:])
fmt.Println("Result:", *addA + *addB)
case "subtract":
subtractCmd.Parse(os.Args[2:])
fmt.Println("Result:", *subtractA - *subtractB)
default:
fmt.Println("Unknown subcommand.")
os.Exit(1)
}
}
Explanation:
flag.NewFlagSet()
: Creates a new flag set for the subcommand.addCmd.Parse(os.Args[2:])
: Parses the arguments for the “add” subcommand.subtractCmd.Parse(os.Args[2:])
: Parses the arguments for the “subtract” subcommand.- The program switches between
add
andsubtract
based on the first argument passed.
Running the Program with Subcommands:
go run cmdutil.go add -a=5 -b=3
Output:
Result: 8
go run cmdutil.go subtract -a=10 -b=4
Output:
Result: 6
4. Third-Party Libraries for Command-Line Utilities
For more advanced command-line utilities, there are third-party libraries like Cobra and Kingpin that provide additional features, including nested subcommands, better argument parsing, and help generation.
Cobra Library Example:
The Cobra package simplifies building modern CLI applications.
go get -u github.com/spf13/cobra/cobra
You can create your command-line applications with predefined patterns and better code organization using Cobra. It’s widely used by projects like Kubernetes and Docker.
Key Takeaways:
- Command-line utilities are a great way to interact with programs from the terminal.
- GoLang provides native support for handling command-line arguments using the
os
andflag
packages. - With subcommands, you can build more complex command-line tools for different tasks.
- For more advanced use cases, third-party libraries like Cobra are recommended.