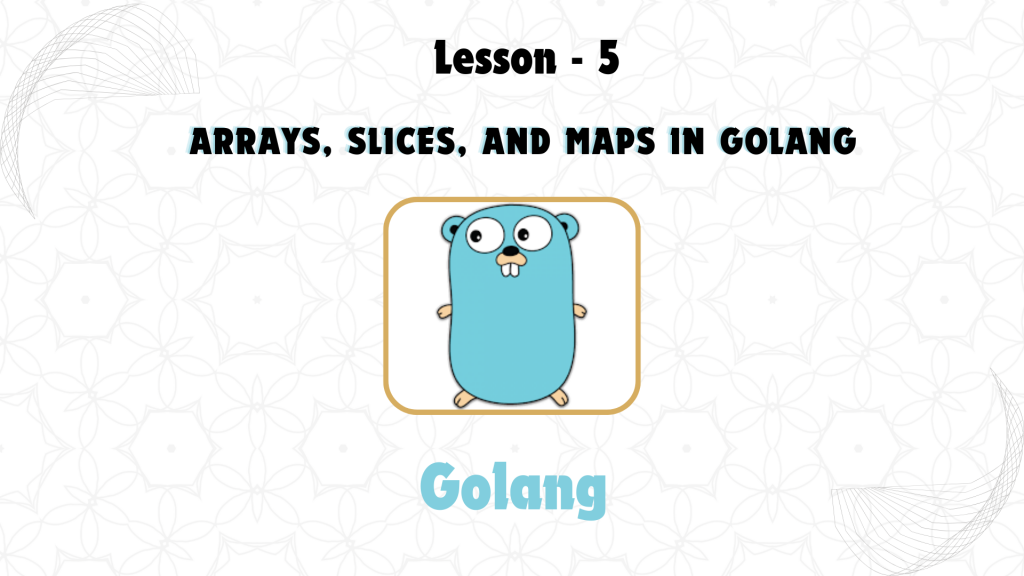
1. Arrays in GoLang An array is a fixed-size sequence of elements of the same type. The size of an array is defined at the time of declaration, and once set, it cannot change.
Here’s how you declare an array in Go:
var numbers [5]int // An array of 5 integers
numbers[0] = 1 // Assigning value to the first element
fmt.Println(numbers) // Output: [1 0 0 0 0]
You can also declare and initialize an array in one line:
arr := [3]string{"Go", "is", "awesome"}
fmt.Println(arr) // Output: [Go is awesome]
2. Slices in GoLang Slices are more flexible than arrays, as they provide a dynamic, resizable view into the elements of an array. Slices have a length and a capacity, but unlike arrays, their size can change dynamically.
Creating a slice from an array:
numbers := [5]int{1, 2, 3, 4, 5}
slice := numbers[1:4] // Creates a slice from index 1 to 3
fmt.Println(slice) // Output: [2 3 4]
Go also has a built-in make
function for creating slices:
slice := make([]int, 5) // Creates a slice of length 5
You can append elements to a slice using the append
function:
slice = append(slice, 6, 7, 8)
fmt.Println(slice) // Output: [0 0 0 0 0 6 7 8]
3. Maps in GoLang Maps in Go are key-value data structures, like dictionaries in other languages. They are extremely efficient and offer fast lookups, insertion, and deletion of elements.
Here’s how you declare and initialize a map in Go:
person := make(map[string]string)
person["name"] = "John"
person["age"] = "30"
fmt.Println(person) // Output: map[age:30 name:John]
You can also initialize a map with values:
person := map[string]string{
"name": "Alice",
"age": "25",
}
fmt.Println(person) // Output: map[age:25 name:Alice]
To access and update elements in a map, you use the key:
fmt.Println(person["name"]) // Output: Alice
person["age"] = "26" // Updating the value for the key "age"
To delete an element from a map, use the delete
function:
delete(person, "age")
fmt.Println(person) // Output: map[name:Alice]
You can also check if a key exists in a map using the comma-ok idiom:
value, ok := person["name"]
if ok {
fmt.Println("Key exists:", value)
} else {
fmt.Println("Key does not exist")
}
Lesson Summary:
- Arrays in Go are fixed-size sequences of elements of the same type.
- Slices are more flexible and dynamic, allowing resizing and easy manipulation.
- Maps store key-value pairs and allow efficient access and modifications.
This concludes Lesson 5: Arrays, Slices, and Maps in GoLang. By mastering these core data structures, you’ll be able to store and manipulate collections of data effectively in Go!