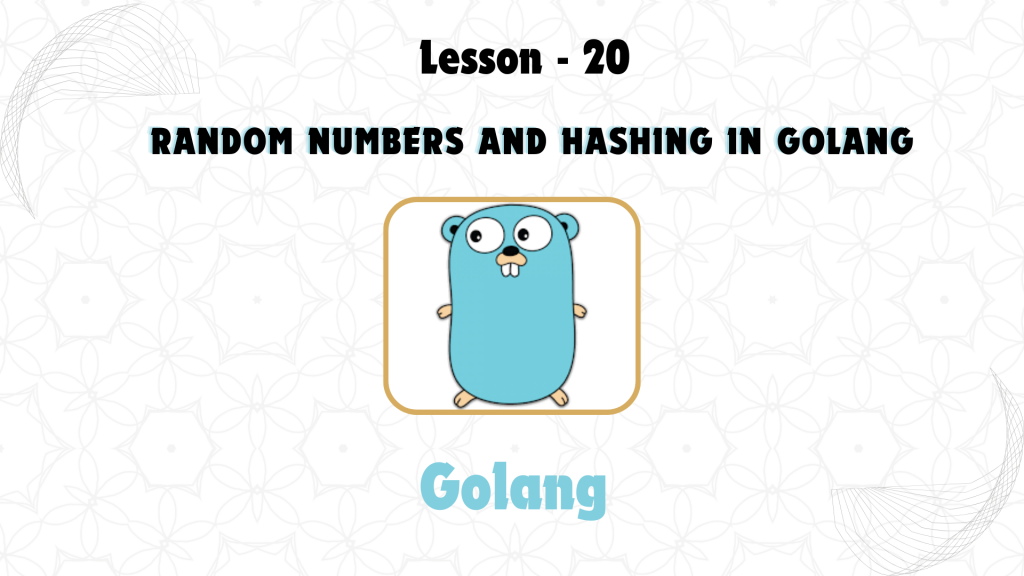
In Lesson 20, we explore two important concepts in GoLang: generating random numbers and hashing. Random numbers are useful in a variety of applications like games, cryptography, and simulations. Hashing, on the other hand, is fundamental to security, data verification, and many algorithms.
1. Generating Random Numbers in GoLang
The GoLang math/rand
package allows us to generate pseudo-random numbers. These are numbers generated using a deterministic algorithm, but they appear random.
Generating Random Integers
You can generate random integers using the rand.Intn()
function, which produces a non-negative pseudo-random number in the range [0, n)
.
Example:
package main
import (
"fmt"
"math/rand"
"time"
)
func main() {
rand.Seed(time.Now().UnixNano()) // Seed the random number generator
randomNumber := rand.Intn(100) // Random number between 0 and 99
fmt.Println("Random number:", randomNumber)
}
Explanation:
rand.Seed(time.Now().UnixNano())
: Seeds the random number generator using the current time (to ensure different results each time).rand.Intn(100)
: Generates a random integer between 0 and 99.
Generating Random Floats
You can also generate random floating-point numbers in the range [0.0, 1.0)
using rand.Float64()
.
Example:
package main
import (
"fmt"
"math/rand"
)
func main() {
randomFloat := rand.Float64() // Random float between 0.0 and 1.0
fmt.Println("Random float:", randomFloat)
}
Generating Random Numbers in a Range
To generate random numbers within a specific range (e.g., between 10 and 20), you can use simple arithmetic.
Example:
package main
import (
"fmt"
"math/rand"
)
func main() {
min := 10
max := 20
randomInRange := rand.Intn(max-min) + min
fmt.Println("Random number between 10 and 20:", randomInRange)
}
2. Hashing in GoLang
Hashing is a process of transforming input data into a fixed-size value or hash code. It is used for data integrity checks, password storage, digital signatures, and more. In GoLang, the crypto
package provides several hashing algorithms like SHA256, MD5, and SHA1.
SHA256 Hashing
The crypto/sha256
package provides functions to compute SHA-256 hashes.
Example:
package main
import (
"crypto/sha256"
"fmt"
)
func main() {
data := []byte("Hello, GoLang!")
hash := sha256.Sum256(data)
fmt.Printf("SHA-256 hash: %x\n", hash)
}
Explanation:
sha256.Sum256(data)
: Computes the SHA-256 hash of the input data (Hello, GoLang!
).%x
: Formats the byte slice as a hexadecimal string.
Comparing Hashes
Hashing is often used to compare data, for example, comparing two files or verifying data integrity.
Example:
package main
import (
"crypto/sha256"
"fmt"
)
func main() {
data1 := []byte("Hello, GoLang!")
data2 := []byte("Hello, GoLang!")
hash1 := sha256.Sum256(data1)
hash2 := sha256.Sum256(data2)
if hash1 == hash2 {
fmt.Println("Hashes are equal")
} else {
fmt.Println("Hashes are different")
}
}
Explanation:
- The code compares the SHA-256 hashes of
data1
anddata2
to check if they are equal.
Base64 Encoding
Base64 encoding is used to encode binary data into an ASCII string. This is useful when you need to represent binary data in text form, such as in URLs, file paths, or JSON.
Example:
package main
import (
"encoding/base64"
"fmt"
)
func main() {
data := "Hello, GoLang!"
encoded := base64.StdEncoding.EncodeToString([]byte(data))
fmt.Println("Base64 Encoded:", encoded)
decoded, _ := base64.StdEncoding.DecodeString(encoded)
fmt.Println("Base64 Decoded:", string(decoded))
}
Explanation:
base64.StdEncoding.EncodeToString([]byte(data))
: Encodes the stringHello, GoLang!
to base64 format.base64.StdEncoding.DecodeString(encoded)
: Decodes the base64 string back to its original form.
Key Takeaways:
- Random number generation in GoLang is done using the
math/rand
package, and you can generate integers, floats, and numbers within a specific range. - Hashing, specifically using algorithms like SHA256, is crucial for security and data verification.
- Base64 encoding is commonly used to represent binary data as ASCII strings.
Mastering these concepts will prepare you for tasks ranging from cryptography to secure data transmission in GoLang applications.