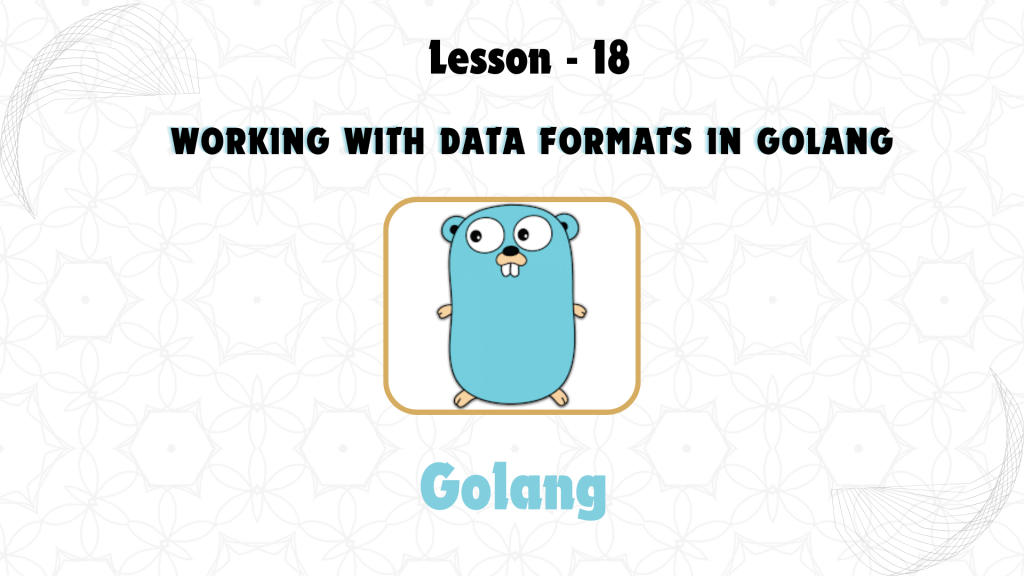
In Lesson 18, we will explore how GoLang handles two of the most commonly used data formats in modern programming: JSON and XML. GoLang provides built-in libraries to work with these formats, making it easy to serialize and deserialize data between different systems.
1. Working with JSON in GoLang
JSON (JavaScript Object Notation) is a lightweight data interchange format that is easy for humans to read and write and easy for machines to parse and generate. GoLang provides the encoding/json
package to handle JSON encoding and decoding.
Encoding (Marshalling) Go Structs to JSON
Marshalling is the process of converting Go structs (or other Go data types) into JSON.
Example:
package main
import (
"encoding/json"
"fmt"
)
type Person struct {
Name string `json:"name"`
Age int `json:"age"`
Gender string `json:"gender"`
}
func main() {
p := Person{Name: "Alice", Age: 30, Gender: "Female"}
jsonData, err := json.Marshal(p)
if err != nil {
fmt.Println(err)
}
fmt.Println(string(jsonData)) // Output: {"name":"Alice","age":30,"gender":"Female"}
}
Explanation:
- The
json.Marshal()
function converts thePerson
struct to a JSON string. - Struct tags like
json:"name"
are used to specify the key names for the JSON fields.
Decoding (Unmarshalling) JSON into Go Structs
Unmarshalling is the process of converting a JSON string into a Go struct.
Example:
package main
import (
"encoding/json"
"fmt"
)
type Person struct {
Name string `json:"name"`
Age int `json:"age"`
Gender string `json:"gender"`
}
func main() {
jsonData := `{"name":"Alice","age":30,"gender":"Female"}`
var p Person
err := json.Unmarshal([]byte(jsonData), &p)
if err != nil {
fmt.Println(err)
}
fmt.Println(p.Name, p.Age, p.Gender) // Output: Alice 30 Female
}
Explanation:
- The
json.Unmarshal()
function takes a JSON string (as[]byte
) and converts it into a Go struct. In this example, it populates thePerson
struct with data from the JSON string.
2. Working with XML in GoLang
XML (Extensible Markup Language) is another format used for data exchange, often found in older web services and configuration files. GoLang provides the encoding/xml
package for working with XML.
Encoding (Marshalling) Go Structs to XML
Marshalling Go structs into XML format is similar to JSON encoding.
Example:
package main
import (
"encoding/xml"
"fmt"
)
type Person struct {
XMLName xml.Name `xml:"person"`
Name string `xml:"name"`
Age int `xml:"age"`
Gender string `xml:"gender"`
}
func main() {
p := Person{Name: "Alice", Age: 30, Gender: "Female"}
xmlData, err := xml.MarshalIndent(p, "", " ")
if err != nil {
fmt.Println(err)
}
fmt.Println(string(xmlData))
}
Output:
<person>
<name>Alice</name>
<age>30</age>
<gender>Female</gender>
</person>
Explanation:
xml.MarshalIndent()
converts the struct into a formatted XML string with indentation for readability.- The
xml:"name"
tags define how the struct fields are mapped to XML elements.
Decoding (Unmarshalling) XML into Go Structs
Just like JSON, XML data can be unmarshalled into Go structs.
Example:
package main
import (
"encoding/xml"
"fmt"
)
type Person struct {
Name string `xml:"name"`
Age int `xml:"age"`
Gender string `xml:"gender"`
}
func main() {
xmlData := `<person><name>Alice</name><age>30</age><gender>Female</gender></person>`
var p Person
err := xml.Unmarshal([]byte(xmlData), &p)
if err != nil {
fmt.Println(err)
}
fmt.Println(p.Name, p.Age, p.Gender) // Output: Alice 30 Female
}
Explanation:
- The
xml.Unmarshal()
function takes the XML string (as[]byte
) and maps it into the corresponding Go struct fields.
Key Takeaways:
- GoLang makes working with JSON and XML data formats straightforward through its
encoding/json
andencoding/xml
packages. - You can easily marshal and unmarshal Go data types like structs into JSON or XML formats, making Go ideal for building web APIs or handling data interchange between services.
- Understanding how to work with these data formats is crucial for building applications that need to communicate with external services or systems.
With this knowledge, you will be able to handle data formats efficiently in your GoLang projects.