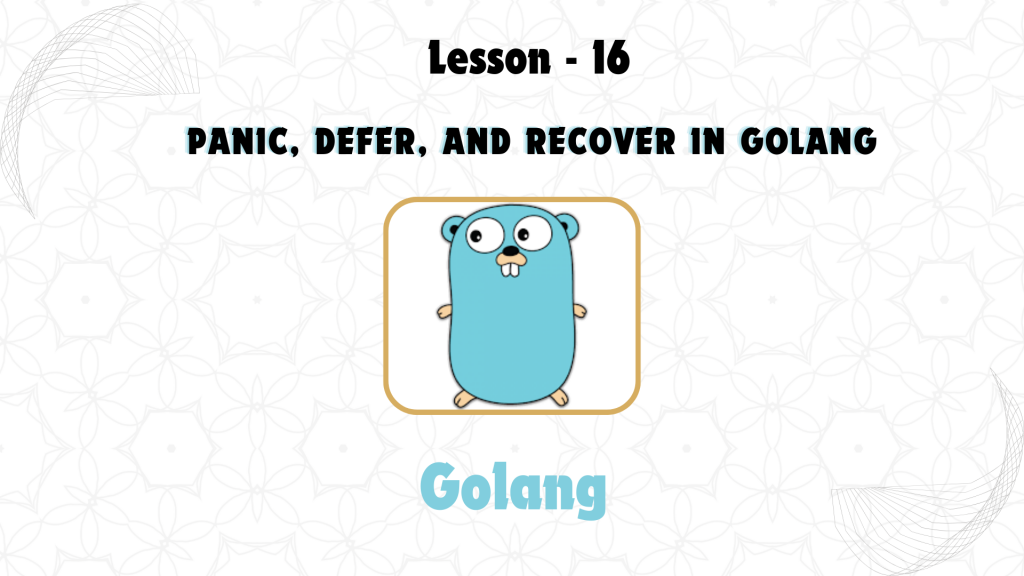
In GoLang, error handling is done explicitly through functions, but sometimes, you need mechanisms to manage unexpected conditions like runtime errors. In this lesson, we will explore panic, defer, and recover in GoLang. These keywords are essential for building robust applications and handling unexpected scenarios gracefully.
1. What is Panic in GoLang?
A panic in GoLang occurs when the program encounters an unexpected error that it cannot handle. It stops the execution of the current function, starts unwinding the stack, and triggers the defer
statements along the way. Panic is typically used for scenarios where the program cannot recover from the error (e.g., critical failures).
Example:
package main
import "fmt"
func divide(x, y int) int {
if y == 0 {
panic("cannot divide by zero")
}
return x / y
}
func main() {
fmt.Println(divide(4, 2))
fmt.Println(divide(4, 0)) // This will trigger a panic
}
Explanation:
- When trying to divide by zero, a panic is triggered using
panic("cannot divide by zero")
. - The program will stop at the point of panic and start unwinding the stack.
2. What is Defer in GoLang?
A defer statement delays the execution of a function until the surrounding function returns. Deferred functions are commonly used to release resources like file handles, database connections, or to perform clean-up tasks. Even if a panic occurs, deferred functions will still execute before the program terminates.
Syntax for Defer:
defer functionName()
Example:
package main
import (
"fmt"
"os"
)
func writeFile() {
file, err := os.Create("test.txt")
if err != nil {
panic(err)
}
defer file.Close() // Ensures the file is closed when the function returns
fmt.Fprintln(file, "Hello, GoLang!")
}
func main() {
writeFile()
}
Explanation:
defer file.Close()
ensures that the file is closed whenwriteFile
finishes, even if the function exits early due to an error or a panic.- Defer is particularly useful for resource management tasks.
3. Using Defer with Panic
When a panic occurs, deferred functions are executed in reverse order before the program crashes. This is useful for cleaning up resources or logging errors before termination.
Example:
package main
import "fmt"
func main() {
defer fmt.Println("Deferred: This will run even after panic")
panic("A critical error occurred")
}
Explanation:
- Even though a panic is triggered, the deferred function will execute before the program terminates.
4. Recovering from Panic
Recover is a built-in function that allows you to regain control of a panicking goroutine. It can only be used inside deferred functions. Recover is used to stop a panic and resume normal execution instead of terminating the program.
Syntax for Recover:
recover()
Example:
package main
import "fmt"
func safeDivision(x, y int) {
defer func() {
if r := recover(); r != nil {
fmt.Println("Recovered from panic:", r)
}
}()
result := x / y
fmt.Println("Result:", result)
}
func main() {
safeDivision(4, 2)
safeDivision(4, 0) // This will trigger a panic, but it will be recovered
}
Explanation:
- The
defer
function checks for a panic usingrecover()
. - If a panic occurs (in this case, dividing by zero),
recover()
catches the panic, and the program continues without crashing.
5. Best Practices for Panic, Defer, and Recover
- Avoid panics for normal errors: Instead of using panic for expected errors (e.g., file not found), return an error and handle it gracefully. Use panic only for critical, unrecoverable errors.
- Always close resources with defer: Use
defer
to ensure that resources like files, database connections, or network sockets are closed, even if the function encounters an error. - Use recover wisely: Only use
recover()
in scenarios where it makes sense to regain control. For example, in server applications where you want to prevent a single request from crashing the entire server.
Key Takeaways:
- Panic is used to indicate a severe problem that the program cannot recover from. It stops the normal execution flow.
- Defer is used to delay the execution of a function until the surrounding function returns. It is particularly useful for clean-up tasks and resource management.
- Recover is used inside deferred functions to handle panics and prevent the program from crashing.
- Effective use of panic, defer, and recover ensures that your GoLang applications are more resilient and can handle unexpected scenarios gracefully.