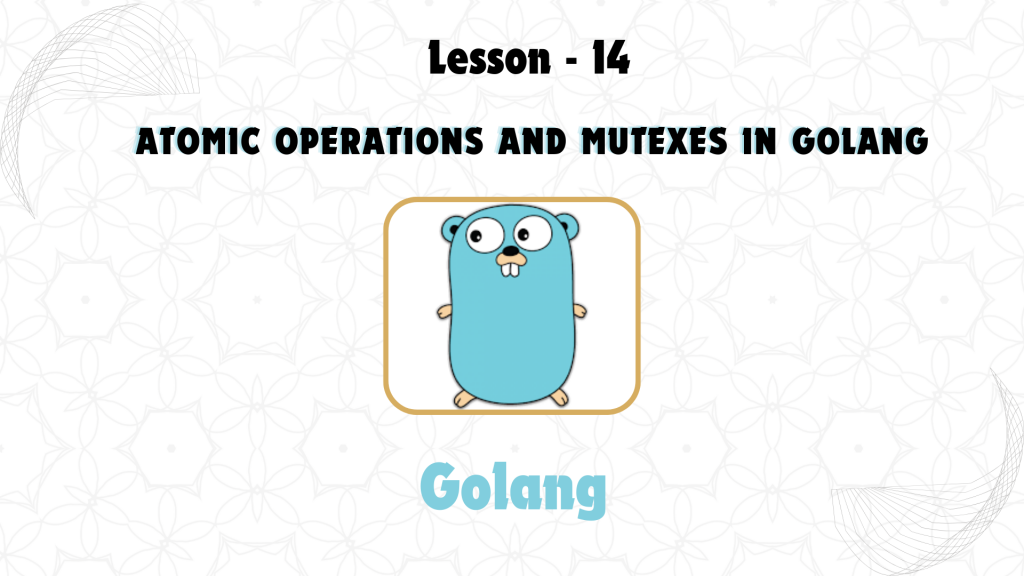
Concurrency is a fundamental aspect of GoLang, and managing shared data between goroutines safely is critical for writing correct concurrent programs. This lesson covers atomic operations, mutexes, and stateful goroutines, which are essential for controlling access to shared resources and preventing race conditions.
1. Atomic Operations in GoLang
Atomic operations allow you to perform operations on shared variables atomically, meaning that these operations are indivisible and cannot be interrupted by other goroutines. This prevents race conditions when multiple goroutines try to update the same variable concurrently.
GoLang provides atomic operations in the sync/atomic
package, which includes functions to atomically read, write, add, or swap integers, pointers, and booleans.
Common Atomic Functions:
atomic.LoadInt32(&val)
– Atomically loads the value of an integer.atomic.StoreInt32(&val, newVal)
– Atomically stores a new value in the integer.atomic.AddInt32(&val, delta)
– Atomically adds a delta to the integer and returns the new value.atomic.CompareAndSwapInt32(&val, old, new)
– Compares the current value and swaps it with a new one if they match.
Example:
package main
import (
"fmt"
"sync"
"sync/atomic"
)
func main() {
var counter int32 = 0
var wg sync.WaitGroup
for i := 0; i < 10; i++ {
wg.Add(1)
go func() {
defer wg.Done()
for j := 0; j < 1000; j++ {
atomic.AddInt32(&counter, 1)
}
}()
}
wg.Wait()
fmt.Println("Final counter value:", counter)
}
Explanation:
atomic.AddInt32(&counter, 1)
increments thecounter
atomically, preventing race conditions when multiple goroutines update it simultaneously.- The final value of
counter
is printed once all goroutines complete their execution.
2. Mutexes in GoLang
A mutex (short for “mutual exclusion”) is a synchronization primitive that provides exclusive access to shared resources. When one goroutine locks the mutex, no other goroutine can access the shared resource until the mutex is unlocked.
GoLang’s sync.Mutex
allows you to lock and unlock sections of code, ensuring that only one goroutine can access the critical section at a time.
Key Mutex Functions:
mutex.Lock()
– Locks the mutex. If another goroutine has already locked it, the current goroutine will block until the mutex is unlocked.mutex.Unlock()
– Unlocks the mutex, allowing other waiting goroutines to proceed.
Example:
package main
import (
"fmt"
"sync"
)
func main() {
var counter int
var mutex sync.Mutex
var wg sync.WaitGroup
for i := 0; i < 10; i++ {
wg.Add(1)
go func() {
defer wg.Done()
for j := 0; j < 1000; j++ {
mutex.Lock() // Lock before accessing the shared counter
counter++
mutex.Unlock() // Unlock after updating the counter
}
}()
}
wg.Wait()
fmt.Println("Final counter value:", counter)
}
Explanation:
mutex.Lock()
ensures that only one goroutine can access thecounter
at a time.- After the goroutine updates the
counter
, it callsmutex.Unlock()
to release the lock, allowing other goroutines to proceed.
3. Deadlocks and Avoiding Them
A deadlock occurs when two or more goroutines are waiting for each other to release a resource, and neither can proceed. This usually happens when mutexes are not properly managed, leading to situations where no progress can be made.
Common Causes of Deadlocks:
- Forgetting to unlock a mutex after locking it.
- Locking multiple mutexes in different orders across different goroutines.
To avoid deadlocks:
- Always ensure that every
Lock()
has a correspondingUnlock()
. - Use
defer
to ensure the mutex is unlocked after the critical section is complete.
Example of Avoiding Deadlocks with defer:
mutex.Lock()
defer mutex.Unlock()
// Critical section code here
4. RWMutex in GoLang
In addition to sync.Mutex
, GoLang also provides sync.RWMutex
, which is a read-write mutex. It allows multiple readers to access the shared resource simultaneously, but only one writer can access it at a time. This can improve performance in scenarios where reading is more frequent than writing.
Key RWMutex Functions:
rwmutex.RLock()
– Acquires a read lock. Multiple goroutines can hold a read lock simultaneously.rwmutex.RUnlock()
– Releases the read lock.rwmutex.Lock()
– Acquires a write lock. Only one goroutine can hold the write lock, and no other goroutines can hold read or write locks simultaneously.rwmutex.Unlock()
– Releases the write lock.
Example:
package main
import (
"fmt"
"sync"
)
func main() {
var counter int
var rwmutex sync.RWMutex
var wg sync.WaitGroup
// Simulate readers
for i := 0; i < 5; i++ {
wg.Add(1)
go func(id int) {
defer wg.Done()
rwmutex.RLock() // Acquire read lock
fmt.Printf("Reader %d read value: %d\n", id, counter)
rwmutex.RUnlock() // Release read lock
}(i)
}
// Simulate a writer
wg.Add(1)
go func() {
defer wg.Done()
rwmutex.Lock() // Acquire write lock
counter = 42
fmt.Println("Writer updated counter to:", counter)
rwmutex.Unlock() // Release write lock
}()
wg.Wait()
}
Explanation:
- The readers acquire a read lock using
rwmutex.RLock()
and can read the sharedcounter
variable concurrently. - The writer acquires a write lock using
rwmutex.Lock()
and updates the value ofcounter
. No other goroutines can read or write during this time.
5. Stateful Goroutines
Stateful goroutines are an alternative approach to managing shared state in GoLang. Instead of using mutexes to lock and unlock shared resources, stateful goroutines encapsulate the state within a goroutine and use channels to communicate with other goroutines.
Key Advantages:
- Avoids the complexity of mutexes and the risk of deadlocks.
- Ensures that only one goroutine can modify the shared state at any given time.
Example:
package main
import (
"fmt"
"time"
)
func main() {
counter := make(chan int)
done := make(chan bool)
// Stateful goroutine
go func() {
count := 0
for {
select {
case <-done:
return
case counter <- count:
count++
}
}
}()
// Read the counter values
for i := 0; i < 5; i++ {
fmt.Println("Counter:", <-counter)
}
// Signal the stateful goroutine to stop
done <- true
time.Sleep(time.Second)
}
Explanation:
- The
counter
goroutine maintains the state (count
), and other goroutines communicate with it via channels. This approach avoids the need for mutexes entirely.
Key Takeaways:
- Atomic operations ensure thread-safe manipulation of shared variables without the need for locking mechanisms.
- Mutexes are used to protect critical sections of code, allowing only one goroutine to access shared resources at a time.
- RWMutex allows multiple concurrent readers but only one writer, improving performance in read-heavy applications.
- Stateful goroutines encapsulate state management within a goroutine, avoiding the complexity of mutexes and deadlocks.