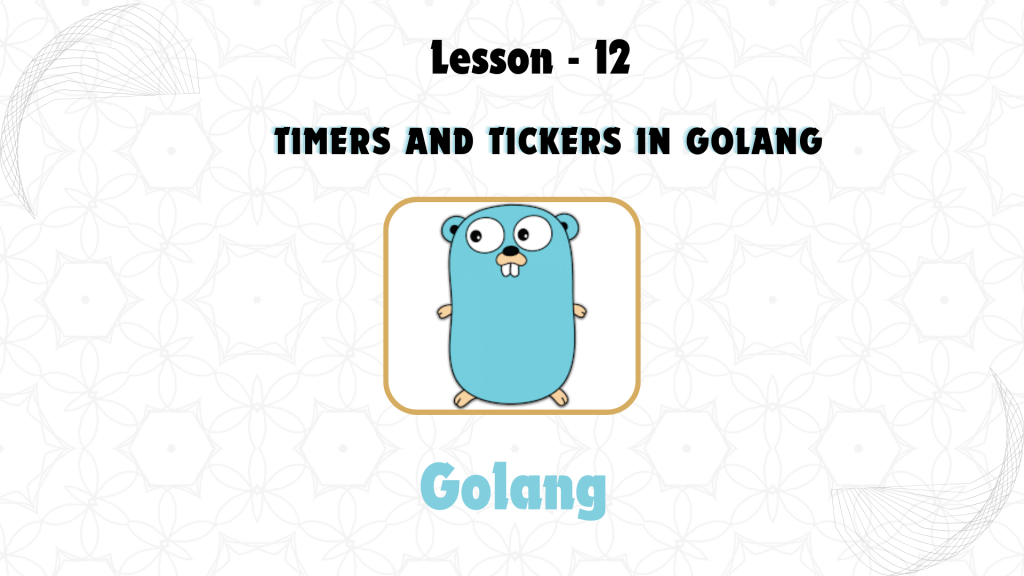
Timers and tickers are fundamental tools in GoLang for managing time-based operations. They allow developers to execute code at specific intervals or after a delay, providing precise control over time-based functionality in concurrent programs.
1. Timers in GoLang
A timer in GoLang represents a single event that will occur after a set duration. It’s often used to trigger an action after a delay. The time.NewTimer
function is used to create a timer, and it sends a message on its channel when the timer expires.
Example:
package main
import (
"fmt"
"time"
)
func main() {
timer := time.NewTimer(2 * time.Second)
fmt.Println("Timer started")
<-timer.C // Blocking until timer sends a message
fmt.Println("Timer expired")
}
- In this example, a timer is created to expire after 2 seconds. The program waits (blocks) until the timer expires, then prints a message.
Stopping and Resetting Timers
- You can stop a timer before it expires using
timer.Stop()
, which prevents the event from firing. - To reuse an expired timer, you can use
timer.Reset(duration)
to reset its duration.
Example:
timer := time.NewTimer(5 * time.Second)
go func() {
<-timer.C
fmt.Println("Timer expired")
}()
time.Sleep(2 * time.Second)
timer.Stop() // Timer is stopped before expiring
fmt.Println("Timer stopped")
2. Tickers in GoLang
A ticker in GoLang is similar to a timer, but it repeatedly “ticks” at a specified interval, sending events on its channel. Tick-based events are useful for periodic tasks, like polling or updating a UI.
You can create a ticker using time.NewTicker
.
Example:
package main
import (
"fmt"
"time"
)
func main() {
ticker := time.NewTicker(1 * time.Second)
defer ticker.Stop()
for i := 0; i < 5; i++ {
<-ticker.C
fmt.Println("Tick at", time.Now())
}
}
- In this example, the ticker ticks every second, and the program prints a message each time it ticks.
- After five ticks, the ticker is stopped using
ticker.Stop()
to free up resources.
Stopping Tickers
- Just like timers, tickers can be stopped using
ticker.Stop()
when you no longer need the periodic events. - Stopping tickers is crucial in concurrent applications to avoid memory leaks caused by unused tickers that continue running.
Practical Use Cases for Timers and Tickers
- Timers are ideal for scenarios where you want to delay the execution of a task or handle timeouts in concurrent operations.
- Example: Implementing a retry mechanism after waiting for a certain duration.
- Tickers are best suited for regularly occurring tasks, such as sending periodic updates, polling an external service, or running scheduled tasks.
- Example: Monitoring system health by checking metrics every minute.
3. Time.After Function
GoLang provides a utility function time.After(duration)
that returns a channel. This channel is sent a signal after the specified duration, which behaves similarly to a timer.
Example:
package main
import (
"fmt"
"time"
)
func main() {
fmt.Println("Waiting for 2 seconds...")
<-time.After(2 * time.Second)
fmt.Println("2 seconds passed")
}
- This function is often used when you don’t need the control provided by
NewTimer
(e.g., resetting or stopping the timer).
4. Ticker with Select
In concurrent Go programs, you may want to handle ticker events along with other channel operations. The select
statement allows you to listen to multiple channels, including ticker channels, enabling more sophisticated control over time-based events.
Example:
package main
import (
"fmt"
"time"
)
func main() {
ticker := time.NewTicker(500 * time.Millisecond)
done := make(chan bool)
go func() {
time.Sleep(2 * time.Second)
done <- true
}()
for {
select {
case <-done:
fmt.Println("Ticker stopped")
ticker.Stop()
return
case t := <-ticker.C:
fmt.Println("Tick at", t)
}
}
}
- In this example, a ticker ticks every 500 milliseconds, but it is stopped when the
done
channel signals completion after 2 seconds.
5. Comparing Timers and Tickers
- Timers are one-off events. You use them when you need to trigger something after a specific delay.
- Tickers are recurring events. You use them when you need to execute code periodically at regular intervals.
Key Takeaways:
- Timers are used for one-time delays.
- Tickers are used for periodic, repeating events.
- Both timers and tickers rely on channels to signal when an event occurs.
- Managing timers and tickers efficiently by stopping them when no longer needed is crucial to avoid memory leaks in concurrent applications.
Timers and tickers, combined with GoLang’s goroutines and channels, provide a powerful mechanism for building time-dependent and concurrent applications.