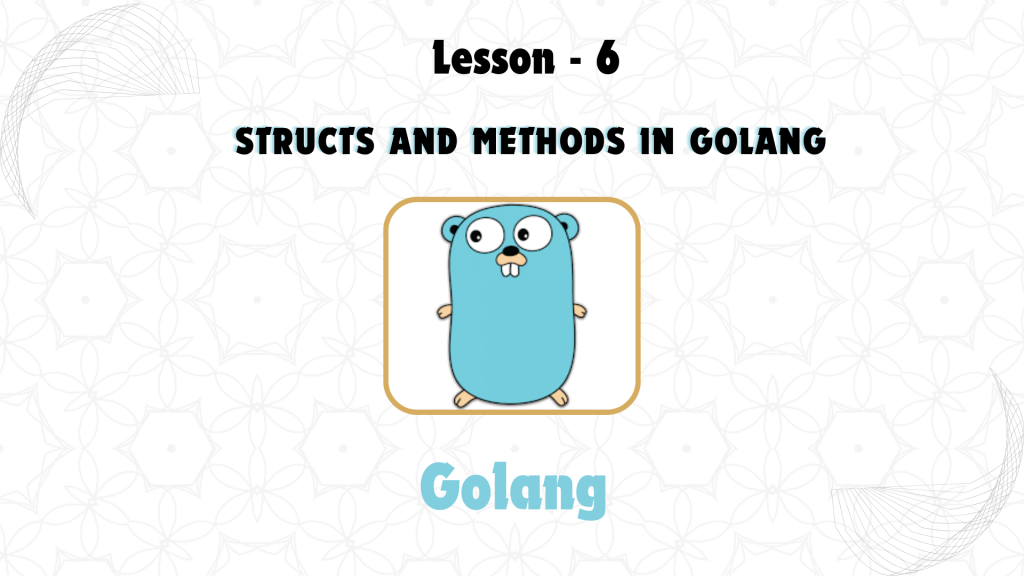
1. Understanding Structs in GoLang Structs in GoLang are composite data types that allow you to group variables of different types under a single name. They are like classes in other programming languages but without the concept of inheritance.
Here’s how you define a struct:
type Person struct {
Name string
Age int
}
You can then create instances of this struct and access its fields:
p := Person{Name: "Alice", Age: 25}
fmt.Println(p.Name) // Output: Alice
fmt.Println(p.Age) // Output: 25
You can also create an instance and set fields separately:
var p Person
p.Name = "Bob"
p.Age = 30
fmt.Println(p) // Output: {Bob 30}
2. Defining and Using Methods In Go, methods are functions that are associated with a specific type, particularly structs. You can define methods with a receiver argument, allowing you to call functions on struct instances.
Here’s how you define a method for the Person
struct:
func (p Person) Greet() {
fmt.Printf("Hello, my name is %s and I am %d years old.\n", p.Name, p.Age)
}
You can then call this method on an instance of Person
:
p := Person{Name: "Alice", Age: 25}
p.Greet() // Output: Hello, my name is Alice and I am 25 years old.
3. Pointer Receivers By default, Go passes copies of values to methods. If you want to modify the original struct data inside a method, you need to use pointer receivers.
Here’s an example:
func (p *Person) HaveBirthday() {
p.Age++
}
This method modifies the Age
field of the original Person
struct:
p := Person{Name: "Bob", Age: 30}
p.HaveBirthday()
fmt.Println(p.Age) // Output: 31
4. Embedding Structs in GoLang Go does not support inheritance, but you can achieve similar behavior using struct embedding. This allows you to reuse fields and methods from one struct in another.
For example:
type Employee struct {
Person // Embedding the Person struct
ID int
Position string
}
Now, instances of Employee
will inherit the fields and methods of Person
:
e := Employee{
Person: Person{Name: "Charlie", Age: 28},
ID: 101,
Position: "Developer",
}
fmt.Println(e.Name) // Output: Charlie
fmt.Println(e.Position) // Output: Developer
You can also call methods from the embedded struct:
e.Greet() // Output: Hello, my name is Charlie and I am 28 years old.
Lesson Summary:
- Structs allow you to define custom types with multiple fields.
- Methods can be defined on structs, allowing you to associate behavior with data.
- Pointer receivers allow methods to modify the original data.
- Struct embedding helps in reusing fields and methods from other structs.
This concludes Lesson 6: Structs and Methods in GoLang. Understanding how to work with structs and methods is essential for organizing data and behavior in GoLang programs.
Stay tuned for Lesson 7, where we’ll continue to expand your GoLang knowledge and help you build a solid foundation for your programming journey.