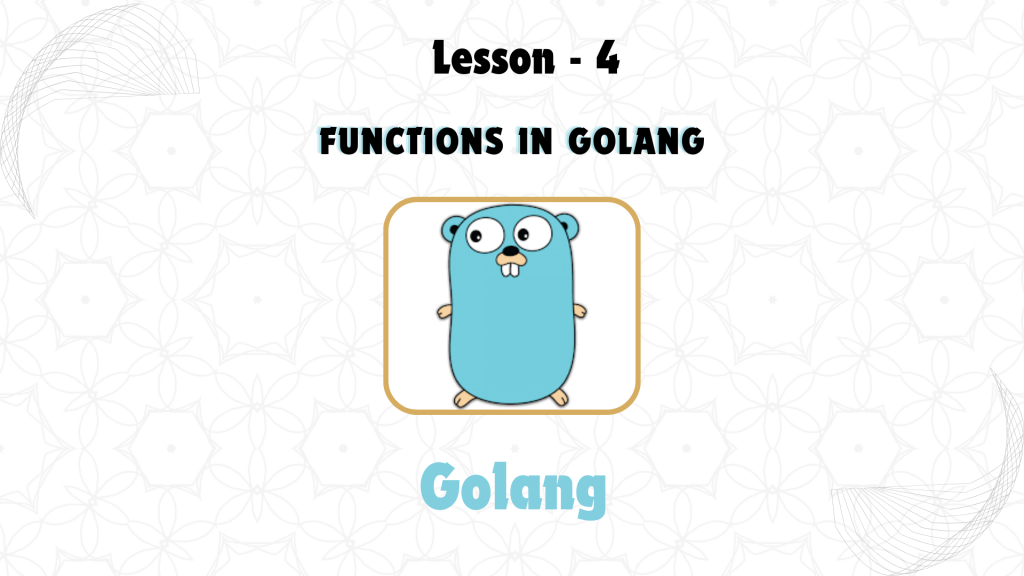
Functions in GoLang are reusable blocks of code that allow you to encapsulate logic and perform specific tasks. Functions enable cleaner, more organized, and modular code by promoting the “DRY” principle—Don’t Repeat Yourself. In this lesson, we will cover how to define and call functions, pass parameters, return values, and explore advanced concepts like variadic functions and closures.
1. Defining and Calling Functions
A function in GoLang is defined using the func
keyword, followed by the function name, a set of parameters, and a return type (if any).
Syntax:
func functionName(parameters) returnType {
// function body
}
Example:
package main
import "fmt"
func greet() {
fmt.Println("Hello, World!")
}
func main() {
greet() // Calling the function
}
Key Points:
- The
func
keyword is used to define a function. - Functions can be called by using their name followed by parentheses.
- A function can return zero or more values.
2. Function Parameters and Return Values
Functions in GoLang can accept parameters and return values, making them highly versatile.
Example:
package main
import "fmt"
// Function with parameters and return value
func add(a int, b int) int {
return a + b
}
func main() {
result := add(5, 3) // Calling the function
fmt.Println("Sum:", result)
}
Multiple Return Values:
GoLang allows functions to return multiple values, which is a very useful feature, especially for error handling.
func divide(a, b float64) (float64, error) {
if b == 0 {
return 0, fmt.Errorf("Division by zero")
}
return a / b, nil
}
func main() {
result, err := divide(10, 2)
if err != nil {
fmt.Println("Error:", err)
} else {
fmt.Println("Result:", result)
}
}
Key Points:
- You can define functions that return more than one value, which is useful for returning results along with errors.
- Return values are listed in the parentheses after the function’s parameter list.
3. Variadic Functions
A variadic function in GoLang can accept a variable number of arguments. This is particularly useful when you don’t know how many arguments will be passed into the function.
Syntax:
func functionName(arg ...type) {
// function body
}
Example:
package main
import "fmt"
// Variadic function that sums numbers
func sum(numbers ...int) int {
total := 0
for _, num := range numbers {
total += num
}
return total
}
func main() {
result := sum(1, 2, 3, 4, 5)
fmt.Println("Sum:", result)
}
Key Points:
- The
...
before the type allows the function to accept any number of arguments of that type. - You can pass a slice to a variadic function by appending
...
to the slice variable.
4. Anonymous Functions and Closures
GoLang allows you to define anonymous functions, which are functions without a name. These functions can be immediately invoked or assigned to variables. Closures are a special type of anonymous function that “closes over” its environment, meaning it can access variables from the scope in which it was created.
Example of Anonymous Function:
package main
import "fmt"
func main() {
// Anonymous function
func() {
fmt.Println("Anonymous function executed!")
}()
}
Example of Closure:
package main
import "fmt"
func main() {
// Closure capturing the outer variable `x`
x := 10
increment := func() int {
x++
return x
}
fmt.Println("Incremented Value:", increment())
fmt.Println("Incremented Again:", increment())
}
Key Points:
- Anonymous functions can be used as function literals or assigned to variables.
- Closures allow functions to “remember” and reference variables from their surrounding scope even after the outer function has completed execution.
Conclusion
In this lesson, we explored GoLang functions, covering topics such as defining and calling functions, passing parameters, and handling return values. We also dived into advanced topics like variadic functions and closures. These concepts are fundamental for writing modular, reusable, and efficient GoLang programs.
In the next lesson, we will look at Arrays, Slices, and Maps, which are GoLang’s essential data structures. Stay tuned!