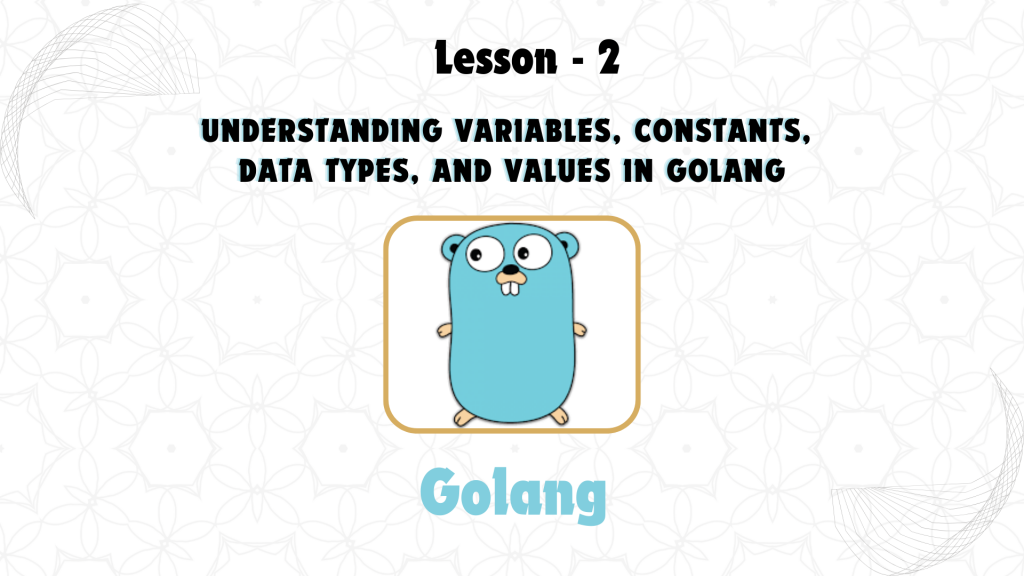
In this lesson, we’ll dive into the core building blocks of GoLang: variables, constants, data types, and values. These are essential for writing efficient, maintainable, and readable Go programs. By the end of this lesson, you will understand how to declare variables, use constants, work with different data types, and understand GoLang values.
1. Variables in GoLang
Variables in GoLang are used to store data that can be manipulated during program execution. Unlike some languages, GoLang requires you to declare a variable before using it, and it has a unique way of handling types with its type inference.
Declaring Variables
There are several ways to declare variables in Go:
- 1. Using the
var
keyword:- The traditional way to declare a variable is by using the
var
keyword:
- The traditional way to declare a variable is by using the
var name string
name = "GoLang"
2. Shorthand Declaration:
- Go offers a shorthand for declaring and initializing a variable in one step using the
:=
operator:
name := "GoLang"
- This form is useful when Go can infer the type of the variable based on its initial value.
Multiple Variable Declaration
Go allows you to declare multiple variables in one line:
var x, y int = 1, 2
Or using shorthand:
x, y := 1, 2
Zero Values
When you declare a variable without initializing it, Go assigns a zero value by default:
0
for numeric typesfalse
for boolean""
(empty string) for stringsnil
for pointers, slices, maps, etc.
Example:
package main
import "fmt"
func main() {
var name string // Declaring a string variable
var age int // Declaring an integer variable
var isDeveloper bool // Declaring a boolean variable
name = "Alice"
age = 30
isDeveloper = true
fmt.Println("Name:", name)
fmt.Println("Age:", age)
fmt.Println("Is Developer:", isDeveloper)
}
2. Constants in GoLang
Constants are variables whose values cannot be changed once they are assigned. They are declared using the const
keyword.
Declaring Constants
const Pi = 3.14
const Language = "GoLang"
- Constants can only be of basic types like
int
,float64
,string
, orbool
. - Constants must be assigned a value at the time of declaration, and this value cannot be modified later in the program.
Example:
package main
import "fmt"
func main() {
const Pi = 3.14159
const Language = "GoLang"
fmt.Println("Pi:", Pi)
fmt.Println("Language:", Language)
}
3. Data Types in GoLang
GoLang is a statically typed language, which means that every variable has a specific data type that must be declared. Some common data types include:
- Integer Types:
int
,int8
,int16
,int32
,int64
- Unsigned Integer Types:
uint
,uint8
,uint16
,uint32
,uint64
- Floating-Point Types:
float32
,float64
- Boolean Type:
bool
- String Type:
string
Numeric Data Types
Go supports both signed and unsigned integers of varying sizes. It also supports floating-point numbers for handling decimals.
var a int = 42
var b float64 = 3.1415
Boolean Data Type
Boolean values represent true
or false
:
var isActive bool = true
String Data Type
Strings in Go are sequences of characters. You can concatenate strings using the +
operator.
var greeting string = "Hello"
var language string = "GoLang"
fmt.Println(greeting + ", " + language)
4. Values in GoLang
Values in GoLang are the actual data that the variables hold. They can be literal values like 42
, "Hello, Go"
, or 3.14
. These values are assigned to variables or constants and used in the program. Values can be categorized based on their data types, such as:
- Integer Values:
0
,1
,-5
,42
- Floating-Point Values:
3.14
,-2.718
,1.0
- String Values:
"Hello"
,"GoLang"
- Boolean Values:
true
,false
Example of Using Values
package main
import "fmt"
func main() {
var x int = 42 // Integer value
var pi float64 = 3.14 // Floating-point value
var name string = "GoLang" // String value
var isActive bool = true // Boolean value
fmt.Println("Integer Value:", x)
fmt.Println("Floating Value:", pi)
fmt.Println("String Value:", name)
fmt.Println("Boolean Value:", isActive)
}
5. Type Conversion
In Go, variables of one type cannot be directly assigned to a variable of another type. You need to explicitly convert the types.
Example of Type Conversion:
var x int = 42
var y float64 = float64(x)
fmt.Println(y) // Output: 42.0
Here, the integer x
is converted to a float64
before being assigned to y
.
Example Program: Variables, Constants, Data Types, and Values
package main
import "fmt"
func main() {
// Declaring variables
var name string = "GoLang"
var version float64 = 1.17
var isStable bool = true
// Declaring constants
const Pi = 3.14159
const Company = "Google"
// Printing variable values
fmt.Println("Language:", name)
fmt.Println("Version:", version)
fmt.Println("Is Stable:", isStable)
// Printing constant values
fmt.Println("Pi:", Pi)
fmt.Println("Company:", Company)
// Using values
var age int = 30
var salary float64 = 60000.50
var employee string = "Alice"
var isManager bool = false
fmt.Println("Employee:", employee)
fmt.Println("Age:", age)
fmt.Println("Salary:", salary)
fmt.Println("Is Manager:", isManager)
// Type Conversion Example
var ageInFloat float64 = float64(age)
fmt.Println("Age in float:", ageInFloat)
}
Conclusion
In this lesson, you’ve learned how to declare and use variables, constants, and different data types, and you’ve seen how values are assigned and used in GoLang. You’ve also explored type conversion, which is useful when working with mixed data types. These fundamental concepts are the building blocks of GoLang programming and are essential for developing more advanced applications.
In the next lesson, we’ll cover control structures such as loops, conditionals, and how to manage flow control in GoLang programs.