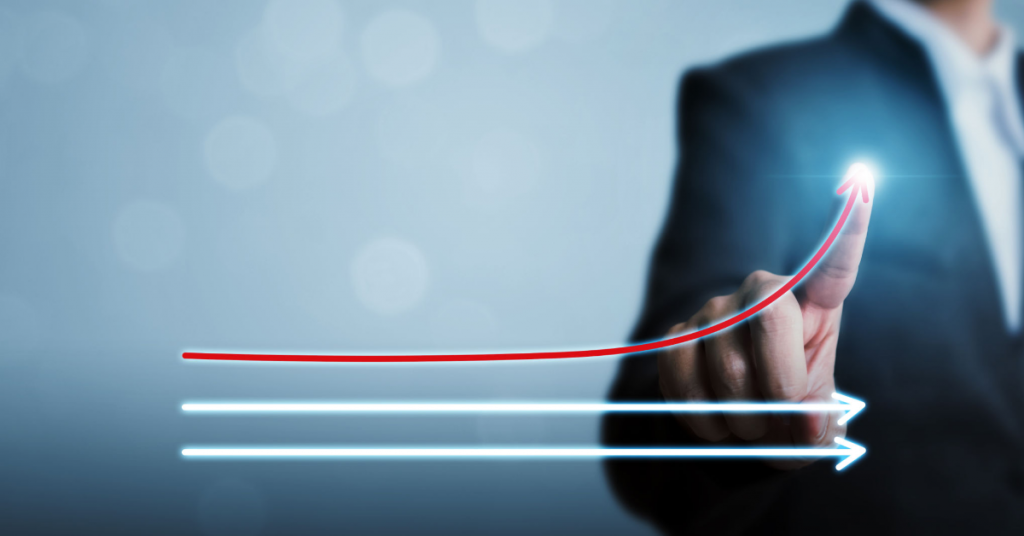
Brief Overview of RESTful APIs
RESTful APIs, or Representational State Transfer APIs, are a set of rules that developers follow when creating APIs. These APIs are designed to be simple, scalable, and stateless, allowing for communication between client and server over the web using standard HTTP methods such as GET, POST, PUT, and DELETE. RESTful APIs often return data in JSON format, making them easily readable and writable by both humans and machines. Key characteristics of RESTful APIs include:
- Statelessness: Each API call is independent, with no stored context between requests on the server.
- Scalability: Designed to handle a growing amount of work or its potential to accommodate growth.
- Uniform Interface: Consistent interface constraints simplify interactions between different components.
Importance of Testing RESTful APIs
Testing RESTful APIs is crucial for several reasons:
- Reliability: Ensures that the API performs as expected under different conditions and returns the correct responses for various requests.
- Security: Identifies vulnerabilities and ensures that unauthorized access is prevented.
- Performance: Helps to determine if the API can handle a large number of requests efficiently.
- Compliance: Ensures that the API adheres to the standards and protocols required by the industry.
- User Satisfaction: Enhances the overall user experience by providing a robust and dependable service.
Without thorough testing, APIs might fail to meet these criteria, leading to system crashes, security breaches, and unsatisfied users.
Introduction to PyTest and Why It’s a Good Choice for Testing APIs
PyTest is a powerful testing framework for Python that is widely used for writing simple as well as scalable test cases. Its features make it an excellent choice for testing RESTful APIs:
- Simplicity: PyTest requires minimal boilerplate code, making it easy to write and understand test cases.
- Flexibility: It supports a wide range of testing needs, from simple unit tests to complex functional tests.
- Fixtures: PyTest’s fixture system allows for efficient setup and teardown of test environments.
- Extensibility: With numerous plugins available, PyTest can be easily extended to meet specific testing requirements.
- Assertions: Provides a rich set of assertion methods to validate API responses effectively.
- Community Support: As an open-source project, PyTest has a large community, ensuring continuous improvements and support.
By leveraging PyTest for RESTful API testing, developers can ensure their APIs are reliable, secure, and performant, ultimately leading to better software quality and user satisfaction.
Setting Up the Environment
Before diving into testing RESTful APIs with PyTest, it’s crucial to set up a proper development environment. This ensures that your testing setup is isolated, manageable, and easy to replicate. Follow these steps to get your environment ready:
Installing PyTest
PyTest is a versatile and easy-to-use testing framework for Python. Installing PyTest is straightforward with pip, Python’s package installer. Open your terminal or command prompt and run the following command:
pip install pytest
This command downloads and installs the latest version of PyTest from the Python Package Index (PyPI).
Setting Up a Virtual Environment
Using a virtual environment is a best practice in Python development. It allows you to create an isolated environment for your project, ensuring that dependencies are managed separately from your global Python installation. Here’s how to set up a virtual environment:
- 1. Install virtualenv (if not already installed):
pip install virtualenv
2. Create a virtual environment:
Navigate to your project directory and run:
virtualenv venv
This command creates a new directory named venv
containing the virtual environment.
3. Activate the virtual environment:
On Windows:
.\venv\Scripts\activate
On macOS and Linux:
source venv/bin/activate
- Once activated, your terminal prompt will change to indicate that you are now working within the virtual environment.
Installing Necessary Packages
With your virtual environment activated, you can now install the necessary packages for testing your RESTful APIs. The most common packages you’ll need are requests
and pytest
. The requests
library is a simple HTTP library for Python, making it easy to send HTTP requests and handle responses.
Run the following command to install both packages:
pip install requests pytest
You can also list these dependencies in a requirements.txt
file for easy installation in the future. Create a requirements.txt
file in your project directory and add the following lines:
requests
pytest
Then, install the packages by running:
pip install -r requirements.txt
By following these steps, you’ve set up a clean, isolated environment for your API testing project. You’ve installed PyTest and other necessary packages, ensuring that your testing setup is ready to go. With this foundation in place, you can now proceed to write and run your RESTful API tests with confidence.
Understanding RESTful APIs
What are RESTful APIs?
RESTful APIs, or Representational State Transfer APIs, are a set of architectural principles for designing networked applications. They use HTTP requests to perform CRUD (Create, Read, Update, Delete) operations on resources, which are typically represented in a structured format like JSON. RESTful APIs are designed to be stateless, meaning each request from a client to the server must contain all the information needed to understand and process the request. This makes RESTful APIs scalable, flexible, and easy to use, making them the de facto standard for web services.
Key Components of RESTful APIs
To understand RESTful APIs, it’s essential to grasp their key components:
- Endpoints:
- Endpoints are specific URLs that represent the resources within a RESTful API. Each endpoint corresponds to a unique resource, such as users, products, or orders. For example,
/api/users
might represent a collection of users, while/api/users/{id}
represents a specific user. - Endpoints are the access points through which clients interact with the API. They are typically organized in a hierarchical structure to reflect the relationship between resources.
- Endpoints are specific URLs that represent the resources within a RESTful API. Each endpoint corresponds to a unique resource, such as users, products, or orders. For example,
- HTTP Methods (GET, POST, PUT, DELETE):
- GET: Retrieves data from the server. It is used to read or fetch information without making any changes to the resource. For example,
GET /api/users
fetches a list of users. - POST: Sends data to the server to create a new resource. For example,
POST /api/users
with user data in the request body creates a new user. - PUT: Updates an existing resource with new data. For example,
PUT /api/users/{id}
updates the information of a specific user. - DELETE: Removes a resource from the server. For example,
DELETE /api/users/{id}
deletes a specific user from the database. - These methods correspond to CRUD operations and are the primary means by which clients interact with resources.
- GET: Retrieves data from the server. It is used to read or fetch information without making any changes to the resource. For example,
- Status Codes:
- Status codes are three-digit numbers that the server returns in response to a client’s request, indicating the outcome of the operation. Common status codes include:
- 200 OK: The request was successful, and the server returned the requested data.
- 201 Created: The request was successful, and a new resource was created.
- 400 Bad Request: The server could not understand the request due to invalid syntax.
- 401 Unauthorized: Authentication is required to access the resource.
- 404 Not Found: The requested resource could not be found.
- 500 Internal Server Error: The server encountered an error while processing the request.
- Status codes help clients understand the result of their requests and take appropriate actions.
- Status codes are three-digit numbers that the server returns in response to a client’s request, indicating the outcome of the operation. Common status codes include:
JSON Format and Its Significance in RESTful APIs
JSON (JavaScript Object Notation) is a lightweight data interchange format that is easy for humans to read and write, and easy for machines to parse and generate. It is the most common format used for transferring data in RESTful APIs due to its simplicity and compatibility with most programming languages.
- Structure: JSON data is organized in key-value pairs, arrays, and nested objects, making it highly flexible for representing complex data structures.
- Significance:
- Interoperability: JSON is language-agnostic, meaning it can be used across different programming languages, making it ideal for APIs that need to communicate between various systems.
- Efficiency: JSON’s lightweight nature reduces the amount of data transmitted over the network, improving the performance of RESTful APIs.
- Human-Readable: JSON’s simplicity and clear structure make it easy for developers to understand and debug.
In RESTful APIs, JSON is typically used in both request bodies (e.g., sending data to create or update resources) and response bodies (e.g., retrieving data from the server). This consistent use of JSON helps maintain a standard communication protocol between clients and servers.
Creating a Sample RESTful API
To demonstrate RESTful API testing with PyTest, we’ll create a simple API using Flask, a lightweight web framework for Python. Flask is ideal for building small to medium-sized APIs quickly and with minimal overhead. Below is a step-by-step guide to setting up a basic RESTful API with Flask and creating sample endpoints for testing.
1. Setting Up Flask
First, ensure that Flask is installed in your virtual environment. You can install Flask using pip:
pip install Flask
Once Flask is installed, you can start building your API.
2. Creating a Simple API
Create a new Python file named app.py
and add the following code:
from flask import Flask, jsonify, request
app = Flask(__name__)
# Sample data to simulate a database
users = [
{"id": 1, "name": "John Doe", "email": "john@example.com"},
{"id": 2, "name": "Jane Smith", "email": "jane@example.com"}
]
# GET /api/users - Get all users
@app.route('/api/users', methods=['GET'])
def get_users():
return jsonify(users)
# GET /api/users/<id> - Get a user by ID
@app.route('/api/users/<int:id>', methods=['GET'])
def get_user(id):
user = next((user for user in users if user["id"] == id), None)
if user:
return jsonify(user)
return jsonify({"error": "User not found"}), 404
# POST /api/users - Create a new user
@app.route('/api/users', methods=['POST'])
def create_user():
new_user = request.json
new_user["id"] = users[-1]["id"] + 1 if users else 1
users.append(new_user)
return jsonify(new_user), 201
# PUT /api/users/<id> - Update an existing user
@app.route('/api/users/<int:id>', methods=['PUT'])
def update_user(id):
user = next((user for user in users if user["id"] == id), None)
if user:
user.update(request.json)
return jsonify(user)
return jsonify({"error": "User not found"}), 404
# DELETE /api/users/<id> - Delete a user
@app.route('/api/users/<int:id>', methods=['DELETE'])
def delete_user(id):
user = next((user for user in users if user["id"] == id), None)
if user:
users.remove(user)
return jsonify({"message": "User deleted"}), 200
return jsonify({"error": "User not found"}), 404
if __name__ == '__main__':
app.run(debug=True)
3. Explanation of Sample Endpoints
GET /api/users:
This endpoint returns a list of all users in JSON format.
Example request: GET http://localhost:5000/api/users
Example response
[
{"id": 1, "name": "John Doe", "email": "john@example.com"},
{"id": 2, "name": "Jane Smith", "email": "jane@example.com"}
]
GET /api/users/{id}:
- This endpoint returns a specific user based on their ID.
- Example request:
GET http://localhost:5000/api/users/1
- Example response:
{"id": 1, "name": "John Doe", "email": "john@example.com"}
POST /api/users:
- This endpoint creates a new user. The user data must be sent in the request body as JSON.
- Example request:
POST http://localhost:5000/api/users
- Request body
{"name": "Alice Johnson", "email": "alice@example.com"}
Example response:
{"id": 3, "name": "Alice Johnson", "email": "alice@example.com"}
PUT /api/users/{id}:
- This endpoint updates an existing user’s data based on their ID.
- Example request:
PUT http://localhost:5000/api/users/1
- Request body:
{"name": "Johnathan Doe", "email": "johnathan@example.com"}
Example response:
{"id": 1, "name": "Johnathan Doe", "email": "johnathan@example.com"}
DELETE /api/users/{id}:
- This endpoint deletes a user based on their ID.
- Example request:
DELETE http://localhost:5000/api/users/1
- Example response:
{"message": "User deleted"}
4. Running the API
To run the API, execute the following command in your terminal:
python app.py
The API will start, and you can access it at http://localhost:5000
. You can use tools like Postman, cURL, or even a web browser to interact with the endpoints.
This simple Flask-based RESTful API provides a basic framework for understanding how APIs work and allows you to create, retrieve, update, and delete user data. These sample endpoints will serve as the foundation for testing with PyTest, helping you practice and refine your API testing skills.
Writing Basic Tests with PyTest
Testing is a crucial part of the development process, and PyTest makes it straightforward and efficient. In this section, we’ll cover the basics of PyTest, how to write your first test case to check API responses, and how to use the requests
library to make HTTP requests.
Introduction to PyTest Basics
PyTest is a flexible testing framework for Python that allows you to write simple test functions as well as complex test suites. Here are some key concepts:
- Test Functions: In PyTest, a test is simply a Python function whose name starts with
test_
. PyTest automatically discovers and runs all such functions when you execute thepytest
command. - Assertions: PyTest uses Python’s built-in
assert
statement to verify that certain conditions hold true. If the condition fails, PyTest reports it as a failed test. - Fixtures: Fixtures are used to set up a test environment. They can be used to create test data, establish database connections, or perform any other setup tasks needed before a test runs. Fixtures are defined using the
@pytest.fixture
decorator.
Writing Your First Test Case to Check the API Response
Let’s start by writing a basic test case that checks if our API’s /api/users
endpoint is returning the correct status code and response data.
Create a new file named test_api.py
in your project directory and add the following code:
import pytest
import requests
BASE_URL = "http://localhost:5000/api/users"
def test_get_users():
# Send a GET request to the /api/users endpoint
response = requests.get(BASE_URL)
# Check that the response status code is 200 OK
assert response.status_code == 200
# Check that the response body contains a list of users
users = response.json()
assert isinstance(users, list)
assert len(users) > 0
# Check the structure of the first user in the list
first_user = users[0]
assert "id" in first_user
assert "name" in first_user
assert "email" in first_user
Explanation of the Test Case
- Importing Libraries:
- We import
pytest
andrequests
. While we don’t explicitly usepytest
in this simple test, it’s required for running the test suite. requests
is used to send HTTP requests to the API.
- We import
- Base URL:
BASE_URL
is set to the endpoint we want to test. In this case, it’s/api/users
.
- Sending a GET Request:
- The test sends a
GET
request to the/api/users
endpoint usingrequests.get(BASE_URL)
.
- The test sends a
- Asserting the Status Code:
- We use
assert
to check that the status code of the response is200 OK
, indicating that the request was successful.
- We use
- Asserting the Response Structure:
- The response body is converted from JSON to a Python list using
response.json()
. - We then check that the response is a list and contains at least one user.
- Finally, we check that the first user in the list has the expected keys:
id
,name
, andemail
.
- The response body is converted from JSON to a Python list using
Running the Test
To run the test, make sure your Flask API is running, then execute the following command in your terminal:
pytest test_api.py
PyTest will discover the test_get_users
function and run it. If everything is set up correctly, you should see output indicating that the test passed.
Using the requests
Library to Make HTTP Requests
The requests
library is a powerful tool for interacting with HTTP services. It simplifies sending HTTP requests and handling responses. Here’s how you can use it in the context of testing:
- GET Request: Used to retrieve data from the server.
response = requests.get(BASE_URL)
POST Request: Used to create new resources.
new_user = {"name": "Alice Johnson", "email": "alice@example.com"}
response = requests.post(BASE_URL, json=new_user)
PUT Request: Used to update existing resources.
updated_user = {"name": "Alice Brown", "email": "alice.brown@example.com"}
response = requests.put(f"{BASE_URL}/1", json=updated_user)
DELETE Request: Used to delete resources.
response = requests.delete(f"{BASE_URL}/1")
Each of these methods returns a response
object, which you can inspect to verify the status code, response headers, and body content.
By understanding the basics of PyTest, writing your first test case, and using the requests
library to interact with your API, you’ve taken the first steps towards effective RESTful API testing. These skills will allow you to ensure that your API behaves as expected and that any changes or new features do not introduce regressions.
Advanced Testing Techniques
In this section, we’ll dive into more advanced testing techniques for RESTful APIs using PyTest. You’ll learn how to test different HTTP methods, validate response status codes, assert response payloads and headers, and implement data-driven tests using PyTest.
1. Testing Different HTTP Methods (GET, POST, PUT, DELETE)
For comprehensive API testing, you need to test all supported HTTP methods to ensure your API handles various operations correctly.
Example Test Cases
Let’s expand our test_api.py
file to include tests for each HTTP method.
import pytest
import requests
BASE_URL = "http://localhost:5000/api/users"
def test_get_users():
response = requests.get(BASE_URL)
assert response.status_code == 200
users = response.json()
assert isinstance(users, list)
assert len(users) > 0
first_user = users[0]
assert "id" in first_user
assert "name" in first_user
assert "email" in first_user
def test_get_user_by_id():
user_id = 1
response = requests.get(f"{BASE_URL}/{user_id}")
assert response.status_code == 200
user = response.json()
assert user["id"] == user_id
assert "name" in user
assert "email" in user
def test_create_user():
new_user = {"name": "Alice Johnson", "email": "alice@example.com"}
response = requests.post(BASE_URL, json=new_user)
assert response.status_code == 201
created_user = response.json()
assert created_user["name"] == new_user["name"]
assert created_user["email"] == new_user["email"]
assert "id" in created_user
def test_update_user():
user_id = 1
updated_user = {"name": "Johnathan Doe", "email": "johnathan@example.com"}
response = requests.put(f"{BASE_URL}/{user_id}", json=updated_user)
assert response.status_code == 200
user = response.json()
assert user["id"] == user_id
assert user["name"] == updated_user["name"]
assert user["email"] == updated_user["email"]
def test_delete_user():
user_id = 2
response = requests.delete(f"{BASE_URL}/{user_id}")
assert response.status_code == 200
result = response.json()
assert result["message"] == "User deleted"
# Ensure the user no longer exists
response = requests.get(f"{BASE_URL}/{user_id}")
assert response.status_code == 404
Explanation of the Test Cases
- GET /api/users: Tests the retrieval of all users and verifies the response status code and structure.
- GET /api/users/{id}: Tests the retrieval of a specific user by ID.
- POST /api/users: Tests the creation of a new user and verifies that the correct data is returned in the response.
- PUT /api/users/{id}: Tests updating an existing user’s details.
- DELETE /api/users/{id}: Tests deleting a user and verifies that the user is no longer retrievable.
2. Validating Response Status Codes
Validating the status code is a fundamental aspect of API testing. Every request should return an expected status code based on the operation performed.
def test_create_user_invalid_data():
# Attempt to create a user with invalid data (e.g., missing email)
invalid_user = {"name": "Invalid User"}
response = requests.post(BASE_URL, json=invalid_user)
assert response.status_code == 400 # Expecting a Bad Request response
In this example, we deliberately send invalid data to the API and expect a 400 Bad Request
response. This ensures the API handles invalid input correctly.
3. Asserting Response Payloads and Headers
In addition to status codes, you should validate the response payload (body) and headers to ensure the API returns the correct data and metadata.
def test_get_user_headers():
user_id = 1
response = requests.get(f"{BASE_URL}/{user_id}")
assert response.status_code == 200
assert response.headers["Content-Type"] == "application/json"
user = response.json()
assert "id" in user
assert "name" in user
assert "email" in user
This test checks the Content-Type
header to confirm that the API is returning JSON data, in addition to validating the payload.
4. Using Parameters and Data-Driven Tests with PyTest
PyTest supports parameterized testing, allowing you to run the same test function with different inputs. This is especially useful for data-driven tests, where the same logic needs to be tested with various inputs.
Example of Parameterized Test
@pytest.mark.parametrize("user_id, expected_name", [
(1, "John Doe"),
(2, "Jane Smith"),
])
def test_get_user_by_id_parametrized(user_id, expected_name):
response = requests.get(f"{BASE_URL}/{user_id}")
assert response.status_code == 200
user = response.json()
assert user["id"] == user_id
assert user["name"] == expected_name
In this test, pytest.mark.parametrize
is used to run the test_get_user_by_id_parametrized
function twice, once for each tuple in the parameter list. This ensures that multiple scenarios are covered with minimal duplication of code.
5. Data-Driven Tests with External Data
You can also drive your tests with data from external sources like JSON files, CSV files, or databases. Here’s an example using a JSON file:
import json
@pytest.mark.parametrize("user_data", json.load(open("test_data.json")))
def test_create_user_with_external_data(user_data):
response = requests.post(BASE_URL, json=user_data)
assert response.status_code == 201
created_user = response.json()
assert created_user["name"] == user_data["name"]
assert created_user["email"] == user_data["email"]
Here, the test reads user data from test_data.json
and uses it to create new users, ensuring that the API works correctly with various inputs.
By utilizing advanced testing techniques such as testing different HTTP methods, validating status codes, asserting response payloads and headers, and implementing data-driven tests, you can ensure that your RESTful APIs are robust and reliable. PyTest’s flexibility and powerful features make it an excellent tool for comprehensive API testing, allowing you to maintain high-quality software with confidence.
Handling Authentication in API Testing
API authentication is essential for securing access to your resources and ensuring that only authorized clients can interact with your API. In this section, we’ll cover the basics of API authentication, specifically Basic Authentication and Token-based Authentication. We’ll also discuss how to write tests for authenticated endpoints and manage authentication tokens in your PyTest tests.
1. Introduction to API Authentication
API Authentication is a process that verifies the identity of a client attempting to access your API. There are several methods to authenticate API requests, but two common approaches are Basic Authentication and Token-based Authentication.
Basic Authentication
- Basic Authentication is a simple authentication method where the client sends a username and password encoded in Base64 in the
Authorization
header of the HTTP request. - While easy to implement, Basic Auth is not secure unless used over HTTPS, as the credentials are easily decoded.
Example of a request with Basic Auth:
GET /api/secure-data HTTP/1.1
Host: api.example.com
Authorization: Basic dXNlcm5hbWU6cGFzc3dvcmQ=
Token-Based Authentication
- Token-Based Authentication is more secure and flexible. After the client successfully authenticates (usually by providing credentials), the server returns a token (like a JWT) that the client includes in subsequent requests.
- The token is usually included in the
Authorization
header as a Bearer token.
Example of a request with a Bearer token:
GET /api/secure-data HTTP/1.1
Host: api.example.com
Authorization: Bearer your_jwt_token_here
2. Writing Tests for Authenticated Endpoints
When testing APIs with authenticated endpoints, your tests must correctly handle authentication to access the resources. Below are examples of testing both Basic and Token-based authenticated endpoints using PyTest.
Example: Basic Authentication
Let’s assume you have an endpoint /api/secure-data
that requires Basic Authentication.
import pytest
import requests
from requests.auth import HTTPBasicAuth
BASE_URL = "http://localhost:5000/api/secure-data"
def test_basic_auth():
username = "testuser"
password = "testpassword"
response = requests.get(BASE_URL, auth=HTTPBasicAuth(username, password))
assert response.status_code == 200
assert "secure" in response.json()["data"]
Explanation:
- HTTPBasicAuth: A helper from the
requests
library to handle Basic Auth. - The test checks that a valid username and password allow access to the endpoint and that the response contains the expected data.
Example: Token-Based Authentication
Suppose you have an endpoint /api/login
that returns a JWT token, which is required to access /api/secure-data
.
import pytest
import requests
BASE_URL = "http://localhost:5000"
LOGIN_URL = f"{BASE_URL}/api/login"
SECURE_URL = f"{BASE_URL}/api/secure-data"
def get_auth_token():
# Simulate logging in to get a token
credentials = {"username": "testuser", "password": "testpassword"}
response = requests.post(LOGIN_URL, json=credentials)
assert response.status_code == 200
token = response.json().get("token")
return token
def test_token_auth():
token = get_auth_token()
# Access secure data with the token
headers = {"Authorization": f"Bearer {token}"}
response = requests.get(SECURE_URL, headers=headers)
assert response.status_code == 200
assert "secure" in response.json()["data"]
Explanation:
- get_auth_token: A helper function that logs in and retrieves the JWT token.
- The
Authorization
header is set with the Bearer token, and the test checks if access to the secure data is granted.
3. Managing Authentication Tokens in Tests
Handling authentication tokens efficiently is crucial for maintaining clean and manageable tests, especially when multiple tests require authentication.
Using PyTest Fixtures for Token Management
PyTest fixtures can be used to manage tokens, ensuring that your test suite can access authenticated endpoints seamlessly.
import pytest
import requests
BASE_URL = "http://localhost:5000"
LOGIN_URL = f"{BASE_URL}/api/login"
SECURE_URL = f"{BASE_URL}/api/secure-data"
@pytest.fixture(scope="session")
def auth_token():
credentials = {"username": "testuser", "password": "testpassword"}
response = requests.post(LOGIN_URL, json=credentials)
assert response.status_code == 200
token = response.json().get("token")
return token
def test_secure_endpoint(auth_token):
headers = {"Authorization": f"Bearer {auth_token}"}
response = requests.get(SECURE_URL, headers=headers)
assert response.status_code == 200
assert "secure" in response.json()["data"]
Explanation:
auth_token
Fixture: This fixture logs in once per test session (controlled byscope="session"
) and provides the token to all tests that require it.- This avoids logging in repeatedly, speeding up the test suite and reducing unnecessary server load.
Handling authentication in API testing involves understanding the authentication method (Basic Auth, Token-based Auth), writing tests that properly include authentication credentials, and managing these credentials efficiently. By using PyTest fixtures and understanding how to interact with authenticated endpoints, you can ensure that your API tests are both comprehensive and maintainable.
Testing Error Responses
Error handling is a crucial aspect of API development, and it’s equally important to test how your API behaves in various error scenarios. This ensures that your API provides meaningful and consistent error messages, which helps developers and clients to diagnose issues effectively.
Importance of Testing Error Scenarios
Testing error scenarios is vital for several reasons:
- User Experience: Proper error handling and messaging enhance the user experience by providing clear feedback on what went wrong.
- Security: Ensuring that your API handles errors gracefully can prevent potential security vulnerabilities, such as exposing sensitive information.
- Robustness: Testing how your API responds to various erroneous inputs helps identify edge cases and ensures that your API is robust against unexpected usage.
Writing Tests for Common Error Responses
Let’s look at how to write tests for common error responses like 404 Not Found
and 400 Bad Request
.
Example: 404 Not Found
The 404 Not Found
error occurs when a client requests a resource that does not exist. It’s important to ensure that your API correctly returns this status code and provides an appropriate error message.
import requests
BASE_URL = "http://localhost:5000/api/users"
def test_get_nonexistent_user():
non_existent_user_id = 9999
response = requests.get(f"{BASE_URL}/{non_existent_user_id}")
assert response.status_code == 404
error_message = response.json().get("error")
assert error_message == "User not found"
Explanation:
- Nonexistent Resource: The test attempts to retrieve a user with an ID that doesn’t exist.
- 404 Assertion: The test checks that the API returns a
404 Not Found
status code. - Error Message: The test verifies that the response includes a meaningful error message, such as
"User not found"
.
Example: 400 Bad Request
The 400 Bad Request
error occurs when the client sends invalid data or parameters. Testing for this scenario ensures that your API validates inputs and provides clear feedback when something is wrong.
def test_create_user_with_invalid_data():
invalid_user_data = {"name": ""} # Missing email, name is empty
response = requests.post(BASE_URL, json=invalid_user_data)
assert response.status_code == 400
error_message = response.json().get("error")
assert error_message == "Invalid input data"
Explanation:
- Invalid Data: The test sends a POST request with incomplete or invalid data.
- 400 Assertion: The test checks that the API returns a
400 Bad Request
status code. - Error Message: The test ensures the response includes a clear error message explaining why the request was rejected.
Simulating Server Errors and Testing the API’s Response
It’s also important to test how your API handles server-side errors, such as when the server encounters an unexpected condition that prevents it from fulfilling the request. A common status code for server errors is 500 Internal Server Error
.
Example: Simulating a Server Error
Simulating server errors can be more complex, as they usually depend on the internal state of the server. However, you can create test scenarios that trigger such conditions or use mock testing to simulate these errors.
import requests
from unittest.mock import patch
BASE_URL = "http://localhost:5000/api/users"
@patch("requests.get")
def test_server_error(mock_get):
# Simulate a server error response
mock_get.return_value.status_code = 500
mock_get.return_value.json.return_value = {"error": "Internal Server Error"}
response = requests.get(f"{BASE_URL}/1")
assert response.status_code == 500
error_message = response.json().get("error")
assert error_message == "Internal Server Error"
Explanation:
- Mocking: The
unittest.mock.patch
decorator is used to simulate the server’s behavior. In this case, we simulate a500 Internal Server Error
. - 500 Assertion: The test checks that the API returns the correct status code and error message when the server encounters an error.
Example: Triggering a Server Error in a Flask API
If you have control over the API, you can create an endpoint that deliberately triggers a server error for testing purposes.
from flask import Flask, jsonify
app = Flask(__name__)
@app.route("/api/trigger-error")
def trigger_error():
raise Exception("Deliberate server error")
# Test for the above endpoint
def test_trigger_server_error():
response = requests.get("http://localhost:5000/api/trigger-error")
assert response.status_code == 500
error_message = response.json().get("error")
assert error_message == "Internal Server Error"
Explanation:
- Error Endpoint: A special route in the API deliberately raises an exception to simulate a server error.
- 500 Assertion: The test checks that this error condition results in the correct status code and error message.
Testing error responses is critical to ensuring that your API is user-friendly, secure, and robust. By writing tests for common error scenarios such as 404 Not Found
and 400 Bad Request
, and by simulating server errors, you can ensure that your API handles all possible situations gracefully. This comprehensive approach to error testing will make your API more reliable and easier to work with for developers and clients alike.
Using Fixtures for Setup and Teardown
PyTest fixtures provide a powerful way to set up and tear down test environments. They help manage test data and state, ensuring that each test runs in a consistent and isolated environment. This section will introduce PyTest fixtures, show how to create fixtures for setting up test data, and explain how to use teardown methods to clean up after tests.
Introduction to PyTest Fixtures
PyTest fixtures are functions that allow you to define setup and teardown code that can be shared across multiple tests. They are used to prepare the environment before tests run and clean up afterward. Fixtures can be applied to individual tests or across an entire test suite.
Key Concepts:
- Fixture Function: A fixture function is defined using the
@pytest.fixture
decorator. It can be used to initialize test data, create objects, or establish a database connection. - Scope: The scope of a fixture determines how long it lasts. Common scopes are:
function
: The fixture is set up and torn down for each test function (default).class
: The fixture is set up once per test class.module
: The fixture is set up once per module (file).session
: The fixture is set up once per test session.
Creating Fixtures for Setting Up Test Data
Fixtures can be used to prepare data or objects that tests need. Here’s an example of how to create a fixture for setting up test data:
Example: Creating a Fixture for Test Data
Suppose you have an API that requires user data to be tested. You can create a fixture to set up this user data.
import pytest
import requests
# URL for the API
BASE_URL = "http://localhost:5000/api/users"
@pytest.fixture
def test_user():
# Set up: Create a user
user_data = {"name": "Test User", "email": "testuser@example.com"}
response = requests.post(BASE_URL, json=user_data)
assert response.status_code == 201
created_user = response.json()
yield created_user
# Teardown: Delete the user
user_id = created_user["id"]
requests.delete(f"{BASE_URL}/{user_id}")
Explanation:
- Setup: The fixture
test_user
creates a user and returns the created user data. - Yield: The
yield
statement is used to return the test data to the test function. - Teardown: After the test function using the fixture completes, the code following
yield
is executed to clean up (e.g., delete the user).
Using Fixtures in Tests
You can use the fixture in your test functions by including it as a parameter.
def test_user_creation(test_user):
assert test_user["name"] == "Test User"
assert test_user["email"] == "testuser@example.com"
Explanation:
- Test Function: The
test_user
fixture is passed to the test function, providing the created user data for assertions.
Using Teardown Methods to Clean Up After Tests
Teardown methods ensure that any resources allocated during setup are properly released after the test has finished. This is particularly important for resources like files, database connections, or API states.
Example: Teardown in Fixtures
In the earlier example, the teardown is handled by the code after yield
in the test_user
fixture. However, you can also use autouse
fixtures or explicit teardown functions if needed.
@pytest.fixture(scope="module")
def setup_database():
# Setup: Initialize database connection
db = initialize_database_connection()
yield db
# Teardown: Close database connection
db.close()
def test_database_query(setup_database):
db = setup_database
result = db.query("SELECT * FROM users")
assert len(result) > 0
Explanation:
- Setup: The fixture
setup_database
initializes a database connection. - Yield: Returns the database connection to the test.
- Teardown: After the test, the database connection is closed.
Using autouse
Fixtures for Global Setup
If you want a fixture to run automatically for every test without explicitly including it as a parameter, you can use autouse
.
@pytest.fixture(autouse=True)
def global_setup_and_teardown():
# Global setup
print("\nSetting up global environment")
yield
# Global teardown
print("\nTearing down global environment")
Explanation:
- Autouse: The
autouse=True
parameter ensures that the fixture runs for every test automatically. - Setup and Teardown: The setup and teardown code runs before and after every test, respectively.
PyTest fixtures are a powerful feature for managing test setup and teardown. By using fixtures, you can prepare test data and clean up resources efficiently, ensuring that each test runs in a consistent and isolated environment. Fixtures improve test reliability and maintainability, making it easier to manage complex test scenarios.
Mocking External Services
When testing APIs, it’s common to interact with external services, such as third-party APIs, databases, or other web services. However, relying on these services during testing can lead to challenges like network dependency, unpredictable response times, and potential costs. This is where mocking comes in handy. Mocking allows you to simulate the behavior of external services, enabling you to test your API in isolation.
Introduction to Mocking and Its Importance in API Testing
Mocking is the practice of replacing real objects or services with simulated ones that mimic their behavior. In the context of API testing, mocking is crucial for several reasons:
- Isolation: By mocking external services, you can test your API without relying on the actual services. This ensures that tests are consistent and not affected by external factors.
- Speed: Mocking external services can significantly speed up tests since there’s no need to wait for network responses.
- Cost: Some external services charge for API requests. Mocking allows you to test without incurring these costs.
- Control: Mocking gives you full control over the responses returned by external services, allowing you to simulate various scenarios, including edge cases and error conditions.
Using the responses
Library to Mock HTTP Responses
The responses
library is a popular Python tool for mocking HTTP responses. It works seamlessly with the requests
library, making it easy to test how your code handles different HTTP responses without actually making any network calls.
Installing the responses
Library
You can install the responses
library using pip:
pip install responses
Example: Mocking an HTTP GET Request
Let’s say your API makes a GET request to an external weather service. You can use responses
to mock this request.
import pytest
import requests
import responses
# URL of the external weather service
WEATHER_API_URL = "http://api.weather.com/v3/weather/conditions"
@responses.activate
def test_get_weather():
# Mock the GET request
responses.add(
responses.GET,
WEATHER_API_URL,
json={"temperature": 72, "condition": "Sunny"},
status=200
)
# Make the actual API call
response = requests.get(WEATHER_API_URL)
# Assertions
assert response.status_code == 200
data = response.json()
assert data["temperature"] == 72
assert data["condition"] == "Sunny"
Explanation:
responses.activate
: This decorator enables theresponses
library for the test function.responses.add
: This method is used to mock an HTTP GET request. It specifies the URL, the JSON response, and the status code.- Assertions: The test checks that the mocked response is as expected.
Example: Mocking an HTTP POST Request
You can also mock POST requests and simulate different scenarios, such as successful submissions or validation errors.
@responses.activate
def test_create_user():
# Mock the POST request
responses.add(
responses.POST,
"http://api.example.com/v1/users",
json={"id": 123, "name": "Test User"},
status=201
)
# Make the actual API call
response = requests.post(
"http://api.example.com/v1/users",
json={"name": "Test User"}
)
# Assertions
assert response.status_code == 201
data = response.json()
assert data["id"] == 123
assert data["name"] == "Test User"
Explanation:
- POST Request: The
responses.add
method is used to mock a POST request, returning a specific response when the API creates a user. - Assertions: The test checks that the user creation was successful and that the response contains the expected data.
Writing Tests with Mocked External Services
Mocking external services is not limited to simple success cases; it can also be used to test how your API handles various failure scenarios.
Example: Simulating a 500 Internal Server Error
Let’s simulate an internal server error from an external service.
@responses.activate
def test_external_service_error():
# Mock the GET request to simulate a server error
responses.add(
responses.GET,
WEATHER_API_URL,
json={"error": "Internal Server Error"},
status=500
)
# Make the actual API call
response = requests.get(WEATHER_API_URL)
# Assertions
assert response.status_code == 500
error_message = response.json().get("error")
assert error_message == "Internal Server Error"
Explanation:
- 500 Error Simulation: The test mocks a GET request that returns a 500 status code, simulating a server error.
- Assertions: The test verifies that the API correctly handles the error scenario.
Example: Simulating a Timeout
You can also simulate a timeout to test how your API handles slow or unresponsive external services.
@responses.activate
def test_external_service_timeout():
# Mock the GET request to simulate a timeout
responses.add(
responses.GET,
WEATHER_API_URL,
body=requests.exceptions.Timeout()
)
# Make the actual API call and handle the timeout
try:
response = requests.get(WEATHER_API_URL, timeout=1)
except requests.exceptions.Timeout:
response = None
# Assertions
assert response is None
Explanation:
- Timeout Simulation: The test mocks a GET request that raises a timeout exception.
- Handling Timeouts: The test checks if the API properly handles the timeout scenario without crashing.
Mocking external services is an essential technique in API testing that allows you to isolate your tests, control the test environment, and simulate various scenarios without relying on actual network calls. The responses
library is a powerful tool for mocking HTTP responses in Python, enabling you to create robust and reliable tests for your API. By using mocking, you can ensure that your API behaves correctly under different conditions, including success, failure, and edge cases.
Continuous Integration and Automation
Integrating your PyTest-based API tests into a Continuous Integration (CI) and Continuous Deployment/Delivery (CD) pipeline ensures that your tests run automatically whenever code changes occur. This process helps catch issues early, maintain code quality, and streamline the deployment process. In this section, we’ll explore how to integrate PyTest with CI/CD pipelines, automatically run API tests on code changes, and generate test reports and coverage metrics.
Integrating PyTest with CI/CD Pipelines
CI/CD pipelines are automated workflows that build, test, and deploy code changes. Tools like GitHub Actions and Jenkins are popular choices for setting up these pipelines.
Example: Integrating PyTest with GitHub Actions
GitHub Actions is a powerful CI/CD tool that allows you to automate workflows directly in your GitHub repository. Here’s how you can integrate PyTest with GitHub Actions:
- Create a GitHub Actions Workflow File:In your repository, create a new directory named
.github/workflows
and add a file namedpython-tests.yml
:
name: Python Tests
on: [push, pull_request]
jobs:
test:
runs-on: ubuntu-latest
steps:
- name: Checkout code
uses: actions/checkout@v2
- name: Set up Python
uses: actions/setup-python@v2
with:
python-version: '3.8'
- name: Install dependencies
run: |
python -m venv venv
source venv/bin/activate
pip install -r requirements.txt
- name: Run tests
run: |
source venv/bin/activate
pytest --junitxml=results.xml --cov=.
- name: Upload test results
if: always()
uses: actions/upload-artifact@v2
with:
name: test-results
path: results.xml
- name: Upload coverage results
if: always()
uses: actions/upload-artifact@v2
with:
name: coverage-report
path: htmlcov/
2. Explanation:
name: Defines the name of the workflow.
on: Specifies the triggers for the workflow. In this case, it runs on every push and pull request.
jobs: Defines the jobs to be executed. Each job runs on a specific environment (in this case, ubuntu-latest
).
Checkout code: Uses actions/checkout@v2
to pull the code from the repository.
Set up Python: Uses actions/setup-python@v2
to set up the Python environment.
Install dependencies: Installs the required Python packages listed in requirements.txt
.
Run tests: Runs the PyTest command, generating a test report (results.xml
) and coverage metrics.
Upload test results: Uploads the test report as an artifact that can be reviewed later.
Upload coverage results: Uploads the coverage report, which can be viewed as an HTML file.
Example: Integrating PyTest with Jenkins
Jenkins is another popular CI/CD tool that can be used to automate your testing process. Here’s how you can integrate PyTest with Jenkins:
Install Jenkins and Set Up a Job:
Install Jenkins on your server or local machine.
Create a new job (e.g., “Python API Tests”) in Jenkins.
Configure the Job:
Source Code Management: Connect your job to your repository (e.g., using Git).
Build Triggers: Set up triggers to start the job automatically, such as when code is pushed to the repository.
Build Environment: Configure your build environment, such as specifying the Python version.
3. Add Build Steps:
Execute Shell: Add a shell command to set up a virtual environment, install dependencies, and run tests.
python -m venv venv
source venv/bin/activate
pip install -r requirements.txt
pytest --junitxml=results.xml --cov=.
4. Post-build Actions:
Publish JUnit Test Result Report: Configure Jenkins to parse the results.xml
file generated by PyTest and display the results in the Jenkins interface.
Publish Coverage Report: You can use plugins like “Cobertura” or “HTML Publisher” to publish and visualize the coverage report.
Running API Tests Automatically on Code Changes
By integrating PyTest into your CI/CD pipeline, you can ensure that your API tests run automatically whenever code changes occur. This helps catch issues early in the development cycle and prevents faulty code from being merged or deployed.
Workflow:
- Commit and Push: Developers commit and push changes to the repository.
- Trigger CI/CD Pipeline: The CI/CD pipeline is triggered automatically by the push or pull request.
- Run Tests: PyTest runs all API tests, verifying the new code’s functionality.
- Test Results: The pipeline generates test reports, and if all tests pass, the code can be merged or deployed.
Generating Test Reports and Coverage Metrics
Generating test reports and coverage metrics is essential for tracking the effectiveness of your tests and ensuring code quality.
Test Reports
PyTest can generate various types of test reports, including XML and HTML formats.
JUnit XML Report:
PyTest can generate a JUnit-style XML report using the --junitxml
option.
This report can be used with CI/CD tools to display test results in a structured format.
pytest --junitxml=results.xml
HTML Report:
- You can use the
pytest-html
plugin to generate a more user-friendly HTML report.
pytest --html=report.html
Coverage Metrics
- 1. Install Coverage:
- Install the
coverage
package using pip:
- Install the
Code coverage metrics help you understand how much of your code is tested. PyTest integrates with the coverage.py
tool to generate these metrics.
pip install coverage
2. Run PyTest with Coverage:
Run your tests with coverage enabled:
pytest --cov=your_package_name --cov-report=html
This command generates a coverage report that can be viewed in HTML format (htmlcov/index.html
).
3. Viewing Coverage Reports:
Open the HTML coverage report in a browser to see detailed coverage information, including which lines of code were executed during the tests and which were not.
Integrating PyTest with CI/CD pipelines like GitHub Actions and Jenkins allows you to automate your API tests, ensuring they run consistently with every code change. This setup helps maintain code quality by catching issues early. By generating test reports and coverage metrics, you can track the effectiveness of your tests and ensure that your codebase remains robust and well-tested.
Best Practices for RESTful API Testing
Testing RESTful APIs is crucial for ensuring the reliability, performance, and security of your application. Following best practices in API testing helps you create maintainable, effective tests that catch issues early and adapt to changes over time. Below are some key best practices to keep in mind when testing RESTful APIs.
Writing Clear and Concise Test Cases
- Use Descriptive Test Names:
- Test names should clearly describe what the test is verifying. This makes it easier to understand the purpose of the test at a glance.
- Example:
test_get_user_returns_200_for_existing_user
instead oftest_user
.
- Focus on Single Responsibility:
- Each test case should focus on a single aspect of the API’s behavior. Avoid testing multiple things in a single test to make it easier to diagnose failures.
- Example: Write separate tests for checking the response status code, validating the response payload, and ensuring correct headers.
- Keep Tests Simple and Focused:
- Tests should be simple and to the point. Avoid unnecessary complexity in test code, which can lead to brittle tests that are hard to maintain.
- Example: Avoid complex logic in test cases; use helper functions or fixtures for setup.
- Document Test Cases:
- Add comments or docstrings to explain the purpose of complex tests, especially when testing edge cases or specific scenarios.
- Example: A comment explaining why a particular edge case is being tested can save time for future developers.
Keeping Tests Independent and Idempotent
- Ensure Test Independence:
- Each test should be able to run independently of other tests. Tests should not rely on the side effects of other tests, such as data being created or deleted in previous tests.
- Example: If your tests create a user, ensure that the test also cleans up by deleting the user afterward.
- Use Fixtures to Manage State:
- Use PyTest fixtures to set up and tear down data or state before and after tests. This ensures that each test starts with a clean slate.
- Example: Use a fixture to create a user before a test and delete the user after the test, so the state is consistent.
- Idempotency in Tests:
- API tests should be idempotent, meaning that running the same test multiple times should produce the same result each time. This is particularly important for tests involving data creation, modification, or deletion.
- Example: Ensure that POST requests that create resources are tested with unique data or that the test cleans up afterward to avoid conflicts in subsequent runs.
- Mock External Dependencies:
- To maintain test independence, mock external services and dependencies. This avoids reliance on third-party APIs or databases that could cause tests to fail due to issues outside of your control.
- Example: Use the
responses
library to mock HTTP requests to external APIs.
Regularly Updating Tests to Cover New Endpoints and Changes
- Keep Tests in Sync with API Changes:
- As your API evolves, update your tests to cover new endpoints, parameters, and response formats. Ensure that deprecated endpoints are removed from tests.
- Example: When adding a new
/api/orders
endpoint, create corresponding test cases that validate its functionality.
- Automate Regression Testing:
- Set up CI/CD pipelines to automatically run tests whenever code changes are made. This ensures that new changes don’t introduce regressions in existing functionality.
- Example: Integrate your test suite with a CI tool like GitHub Actions or Jenkins to run tests on every pull request.
- Review and Refactor Tests Regularly:
- Periodically review your test suite to remove redundant tests, refactor complex test cases, and ensure coverage for critical paths. Regular maintenance keeps your test suite lean and effective.
- Example: Conduct code reviews focused on the test suite to identify and refactor outdated or unnecessary tests.
- Use Coverage Reports to Identify Gaps:
- Use coverage tools like
coverage.py
to identify parts of your code that are not covered by tests. Focus on increasing coverage for critical parts of your API. - Example: If coverage reports show that a new validation rule isn’t tested, add tests that cover both valid and invalid inputs for that rule.
- Use coverage tools like
- Incorporate Feedback Loops:
- Use feedback from production issues, user reports, or QA teams to identify gaps in your test coverage. Incorporate these insights into your test cases to prevent future issues.
- Example: If users report a bug that wasn’t caught by the tests, add a test case to ensure it doesn’t happen again.
Conclusion:
Following these best practices for RESTful API testing helps ensure that your tests are effective, maintainable, and capable of catching issues early. Writing clear and concise test cases makes it easier to understand and manage your tests, while keeping them independent and idempotent ensures reliability across different environments. Regularly updating your tests to cover new endpoints and changes in your API ensures that your test suite evolves with your application, maintaining high quality and robustness over time.